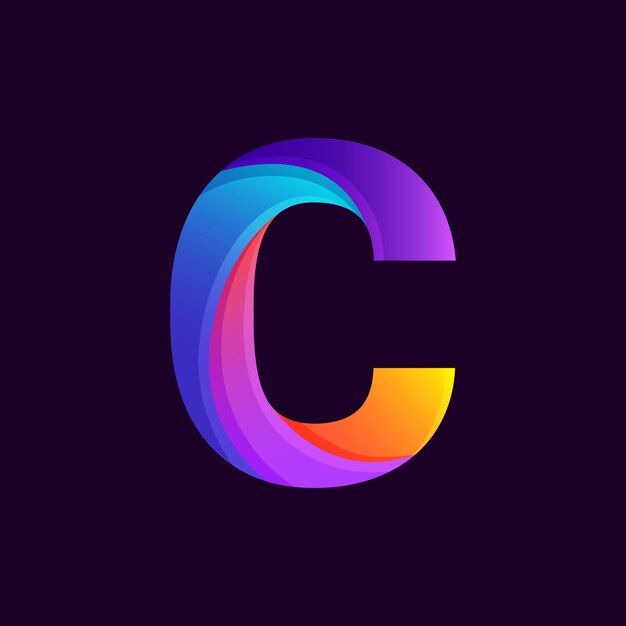
Templates in C++
What are Templates in C++?
- In C++, templates are a powerful feature that allows you to write generic code.
- They enable you to define functions, classes, and data structures that can work with different data types without having to rewrite the code for each specific type.
- Templates are a fundamental part of C++'s support for generic programming.
Types of Templates in C++
- Function Template
- Class Template
C++ Function Template
- Function template in C++ is a blueprint for creating generic functions that can work with different data types.
- It allows you to write a single function definition that can be used with various data types.
- Function templates use a placeholder type (typically denoted as T, though you can use any valid identifier) to represent the generic data type.
Here's the basic syntax of a function template:
1template <typename T>
2ReturnType FunctionName(Parameters) {
3 // Function body that can use the type T
4}
- template <typename T>: This declares a template with a type parameter T.
- ReturnType: Replace this with the actual return type of the function.
- FunctionName: Replace this with the name of your function.
- Parameters: Replace this with the function's parameters, which can also use the generic type T.
Function Template Example
Here's a simple C++ code example of a function template that finds the maximum of two values:
1#include <iostream>
2using namespace std;
3
4// Function template to find the maximum of two values
5template <typename T>
6T Max(T a, T b)
7{
8 return (a > b) ? a : b;
9}
10
11int main()
12{
13 // Example 1: Using the Max function template with integers
14 int intResult = Max(5, 8);
15 cout << "Maximum of 5 and 8 is: " << intResult << endl;
16
17 // Example 2: Using the Max function template with doubles
18 double doubleResult = Max(3.1, 4.5);
19 cout << "Maximum of 3.1 and 4.5 is: " << doubleResult << endl;
20
21 // Example 3: Using the Max function template with characters
22 char charResult = Max('A', 'B');
23 cout << "Maximum of 'A' and 'B' is: " << charResult << endl;
24
25 return 0;
26}
- We define a function template Max that takes two parameters of the same type T and returns the maximum of the two values.
- In the main function, we demonstrate the use of the Max function template with different data types: integers, doubles, and characters.
- The code outputs the maximum value for each data type.
C++ Class Template
- A class template in C++ is a blueprint for creating generic classes that can work with different data types.
- It allows you to define a single class template with placeholders for types, typically denoted as T,
- Here's the basic syntax of a class template:
1template <typename T>
2class ClassName {
3public:
4 // Member variables and functions that use the type T
5};
- template <typename T>: This declares a template with a type parameter T.
- ClassName: Replace this with the desired name for your class.
- Inside the class template, you can define member variables, member functions, and methods that use the generic type T.
Class Template Example
1#include <iostream>
2#include <string>
3
4template <typename ToyType>
5class ToyBox
6{
7private:
8 ToyType toy; // This is where we store our toy
9
10public:
11 // Constructor to add a toy when we create a ToyBox
12 ToyBox(const ToyType &initialToy)
13 {
14 toy = initialToy;
15 }
16
17 // Function to show the toy inside the ToyBox
18 void showToy()
19 {
20 std::cout << "I found a " << toy << " in my ToyBox!" << std::endl;
21 }
22};
23
24int main()
25{
26 // Create a ToyBox for a Teddy Bear
27 ToyBox<std::string> teddyBearBox("Teddy Bear");
28
29 // Show the Teddy Bear inside the ToyBox
30 teddyBearBox.showToy();
31
32 // Create a ToyBox for a Toy Car
33 ToyBox<std::string> toyCarBox("Toy Car");
34
35 // Show the Toy Car inside the ToyBox
36 toyCarBox.showToy();
37
38 return 0;
39}
- We use a class template called ToyBox that can store any type of toy. In our example, we're using strings to represent different types of toys.
- When we create a ToyBox, we put a toy inside it by using the constructor.
- We have a function called showToy() that displays the toy inside the ToyBox.
Overloading Template function in C++
Overloading template functions in C++ allows you to define multiple function templates with the same name but different parameter lists or type constraints.
1include <iostream>
2#include <string>
3using namespace std;
4
5template <typename MagicType>
6class MagicBox
7{
8private:
9 MagicType item;
10
11public:
12 MagicBox(const MagicType &initialItem)
13 {
14 item = initialItem;
15 }
16
17 void doMagic()
18 {
19 cout << "Magic Box does something magical with " << item << "!" << endl;
20 }
21};
22
23// Overload the MagicBox for a "Toy" type
24template <>
25class MagicBox<string>
26{
27private:
28 string toy;
29
30public:
31 MagicBox(const string &initialToy)
32 {
33 toy = initialToy;
34 }
35
36 void doMagic()
37 {
38 cout << "Magic Box makes the " << toy << " disappear!" << endl;
39 }
40};
41
42int main()
43{
44 // Create a Magic Box with a "Rabbit" inside
45 MagicBox<string> rabbitBox("Rabbit");
46 rabbitBox.doMagic();
47
48 // Create a Magic Box with a "Coin" inside
49 MagicBox<string> coinBox("Coin");
50 coinBox.doMagic();
51
52 // Create a generic Magic Box with a number inside
53 MagicBox<int> numberBox(7);
54 numberBox.doMagic();
55
56 return 0;
57}
- We have a MagicBox class template that can do magic with different types of objects.
- When we create a MagicBox, we put an object inside it, and the box performs magic based on the object's type.
Conclusion
Templates in C++ are a powerful feature that allows you to write generic code. Templates enable you to create functions and classes that work with different data types, promoting code reusability and flexibility.