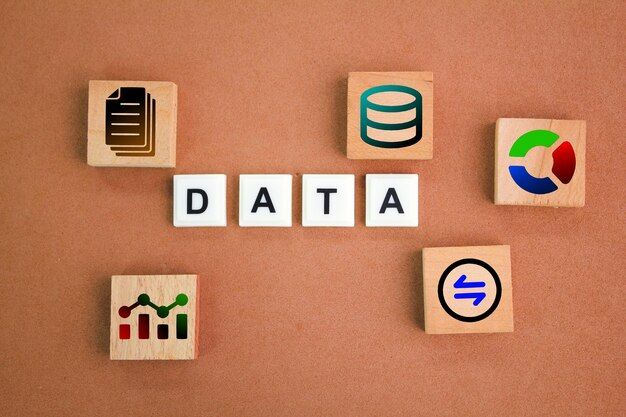
What are Data types in C++
- In C++, data types are classifications that specify which type of data a variable can hold.
- Data types in C++ are crucial because they help ensure type safety, memory allocation, and efficient use of resources. C++ provides a wide range of data types such as
Data Types in C++
- Primary or Built-in or Fundamental data type
- Derived data types
- User-defined data types
Primary or Built-in or Fundamental data type
- Primary data types are the most basic data types in C++. They represent individual values and include:
- int: Represents integers (whole numbers).
- float: Represents single-precision floating-point numbers.
- double: Represents double-precision floating-point numbers.
- char: Represents a single character.
- bool: Represents boolean values (true or false). for example
1#include <iostream>
2using namespace std;
3
4int main() {
5 int integerVar = 42;
6 float floatVar = 3.14;
7 double doubleVar = 2.71828;
8 char charVar = 'A';
9 bool boolVar = true;
10
11 cout << "Integer: " << integerVar << endl;
12 cout << "Float: " << floatVar << endl;
13 cout << "Double: " << doubleVar << endl;
14 cout << "Char: " << charVar << endl;
15 cout << "Bool: " << boolVar << endl;
16
17 return 0;
18}
Derived Data Types
- Derived data types are created by combining fundamental data types or other derived types. Common derived data types include:
- Array: Represents a gathering of elements that share the same data type.
- Pointer: Holds the memory location or address of another variable.
User-Defined Data Types
- User-defined data types are created by programmers to meet specific requirements.
- The most common form of user-defined data types is classes, which encapsulate data and behavior into a single unit.
What are identifiers in C++?
- In C++, an identifier is a name given to various program elements such as variables, functions, classes, and more.
- Identifiers are used to uniquely identify and access these elements within your C++ program. for instance:
Naming Rules
- C++ is case-sensitive, so uppercase and lowercase letters are distinct. For example, "myVar" and "myvar" are treated as different identifiers.
- Identifiers cannot be C++ keywords (reserved words used for language-specific purposes).
Examples of Identifiers
- Variable identifiers: int age, double salary.
- Function identifiers: void calculateArea(), int main().
- Class identifiers: class Rectangle, class Student.
C++ keywords
- C++ keywords, also known as reserved words, are a set of predefined words in the C++ programming language that have special meanings and are reserved for specific purposes.
- These keywords cannot be used as identifiers (names for variables, functions, classes, etc.) because they have predefined roles within the language.
- Here are some common C++ keywords:
- Data Types in C++
- int, double, char, bool, void.
- Control Flow
- if, else, switch, case, default.
- Loops
- while, for, do
- Functions
- return, void
- Classes and Objects
- class, struct, new, delete
- Access Control
- public, private, protected
- Others
- const, static, sizeof, namespace.
C++ Data literals
In C++, data literals, also known as literals, are constant values that are directly specified within the source code of a program.
C++ Type Conversion
- In C++, type conversion (also known as type casting or data type conversion) refers to the process of converting one data type into another.
- This is done to facilitate operations between values of different data types or to ensure that data is compatible with a specific operation.
Conclusion
In C++, data types define the kind of values variables can hold, enabling precise memory allocation and operations.