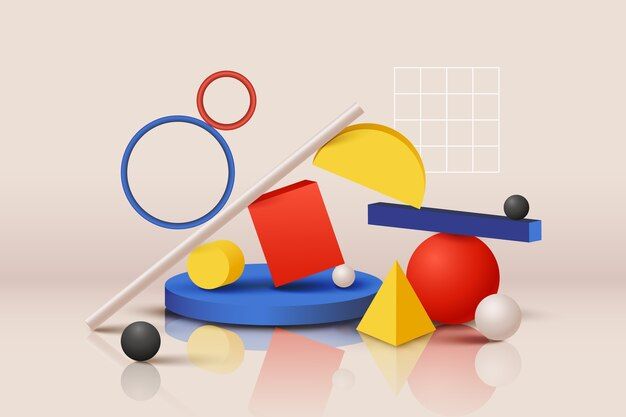
Composition in C++
What is Composition in C++?
- Composition in C++ is a design principle and a relationship between classes that represents a whole-part or contains association.
- It's a more specific form of aggregation where one class (the whole) is composed of one or more objects of another class (the parts).
Key Characteristics of Composition in C++
- Composition is a strong has-a relationship where one class contains another class as a fundamental part.
- Composition allows for encapsulating the behavior and properties of the composed objects within the containing object.
Composition in C++ Example
1#include <iostream>
2using namespace std;
3
4class Engine {
5public:
6 void start() {
7 cout << "Engine started." << endl;
8 }
9};
10
11class Car {
12public:
13 Car() {
14 // Composition: Car "has-a" Engine
15 engine.start();
16 }
17
18 void drive() {
19 cout << "Car is driving." << endl;
20 }
21
22private:
23 Engine engine; // Composition: Car contains an Engine
24};
25
26
27int main() {
28 Car myCar;
29 myCar.drive();
30
31 return 0;
32}
- Car is the containing class, and Engine is the composed class.
- The Car class contains an Engine object as a part of its structure, demonstrating composition.
- When a Car object is created, it automatically starts the engine.
- The Car class encapsulates the behavior of both the car and the engine.
Types of Object Composition
Aggregation
- Aggregation is a "has-a" relationship where one class contains another class as a part.
- It represents a weaker relationship than composition, as the contained objects can exist independently of the containing object.
Composition
- Composition is a strong "has-a" relationship where one class contains another class as a fundamental part.
- Examples include a car containing an engine, or a computer containing a motherboard.
Association
- Association represents a more loosely coupled relationship between classes.
- It indicates that two classes are related but does not specify how one class is contained within the other.
- Associations can be bi-directional, where both classes reference each other, or uni-directional, where one class knows about the other but not vice versa.
- Examples include a teacher having an association with students or a library book being associated with a borrower.
Composition vs Classification
Composition and classification are two different concepts in the context of object-oriented programming:
Composition
- Composition refers to the relationship between classes where one class is composed of or contains another class as a part.
- Independence: Composed objects cannot exist independently of the containing object. They are tightly coupled.
- Examples: A car containing an engine, a computer containing a motherboard, or a house containing rooms are examples of composition.
Classification
- Classification refers to grouping objects into classes based on their common characteristics and behaviors.
- It is the process of categorizing and organizing similar objects under a common class.
- Independence: In classification, objects within the same class may share common characteristics, but they are independent instances. Each instance can exist on its own.
- Examples: In a programming context, classifying animals like "mammals," "birds," or "reptiles" based on their shared characteristics is an example of classification.
Conclusion
Composition in C++ is a fundamental design principle where one class contains another as a part. It represents a strong has-a relationship, enabling the creation of complex, well-structured objects by combining simpler components.