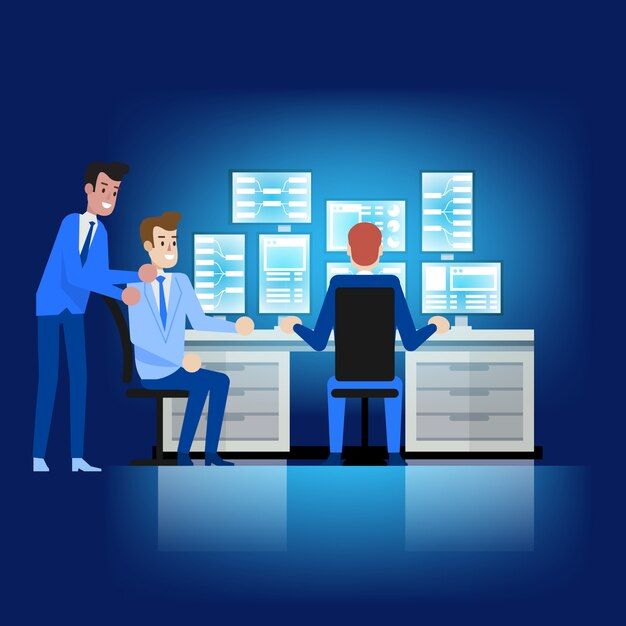
C++ Operators
- Operator in C++ is a symbol or keyword used to perform operations on operands.
- Operands can be variables, constants, or expressions, and operators dictate how these operands are combined.
Operators in C++
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Unary Operators
- Ternary or Conditional Operators
- Miscellaneous Operators
Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations. Here are some commonly used arithmetic operators in C++:
- Addition (+): Adds two values together.
- Subtraction (-): Subtracts one value from another.
- Multiplication (*): Multiplies two values.
- Division (/): Divides one value by another.
- Modulus (%): Returns the remainder of a division operation.
1#include <iostream>
2using namespace std;
3
4int main() {
5 int num1 = 10, num2 = 5;
6
7 // Addition
8 int sum = num1 + num2;
9 cout << "Sum: " << sum << endl;
10
11 // Subtraction
12 int difference = num1 - num2;
13 cout << "Difference: " << difference << endl;
14
15 // Multiplication
16 int product = num1 * num2;
17 cout << "Product: " << product << endl;
18
19 // Division
20 int quotient = num1 / num2;
21 cout << "Quotient: " << quotient << endl;
22
23 // Modulus (remainder)
24 int remainder = num1 % num2;
25 cout << "Remainder: " << remainder << endl;
26
27 return 0;
28}
29
output:
1Sum: 15
2Difference: 5
3Product: 50
4Quotient: 2
5Remainder: 0
Relational Operators
- Relational operators are used to comparing values and determine the relationship between them.
- These operators return either true or false. Common relational operators include:
- Equal to (==): Checks if two values are equal.
- Not equal to (!=): Checks if two values are not equal.
- Greater than (>): Checks if one value is greater than another.
- Less than (<): Check if one value is less than another.
- Greater than or equal to (>=): Checks if one value is greater than or equal to another.
- Less than or equal to (<=): Checks if one value is less than or equal to another.
1#include <iostream>
2using namespace std;
3
4int main() {
5 int num1 = 10, num2 = 5;
6
7 // Equality
8 bool isEqual = (num1 == num2);
9 cout << "Equal: " << isEqual << endl;
10
11 // Inequality
12 bool isNotEqual = (num1 != num2);
13 cout << "Not Equal: " << isNotEqual << endl;
14
15 // Greater than
16 bool isGreaterThan = (num1 > num2);
17 cout << "Greater Than: " << isGreaterThan << endl;
18
19 // Less than
20 bool isLessThan = (num1 < num2);
21 cout << "Less Than: " << isLessThan << endl;
22
23 // Greater than or equal to
24 bool isGreaterOrEqual = (num1 >= num2);
25 cout << "Greater or Equal: " << isGreaterOrEqual << endl;
26
27 // Less than or equal to
28 bool isLessOrEqual = (num1 <= num2);
29 cout << "Less or Equal: " << isLessOrEqual << endl;
30
31 return 0;
32}
output is :
1Equal: 0
2Not Equal: 1
3Greater Than: 1
4Less Than: 0
5Greater or Equal: 1
6Less or Equal: 0
Logical Operators
- Logical operators are used to perform logical operations on Boolean values.
- They are often used in conditional statements and loops. The common logical operators are:
- Logical AND (&&): Returns true if both operands are true.
- Logical OR (||): Returns true if at least one operand is true.
- Logical NOT (!): Returns the opposite of the operand's value.
1#include <iostream>
2using namespace std;
3
4int main() {
5 int a = 10, b = 0;
6
7 // Logical AND (&&)
8 int andResult = (a && b); // 0 (false)
9 cout << "AND Result: " << andResult << endl;
10
11 // Logical OR (||)
12 int orResult = (a || b); // 1 (true)
13 cout << "OR Result: " << orResult << endl;
14
15 // Logical NOT (!)
16 int notResultA = !a; // 0 (false)
17 cout << "NOT Result (a): " << notResultA << endl;
18
19 int notResultB = !b; // 1 (true)
20 cout << "NOT Result (b): " << notResultB << endl;
21
22 return 0;
23}
Logical AND (&&):
- Operand 1: a (with a value of 10)
- Operand 2: b (with a value of 0)
- Result: The logical AND operator (&&) evaluates to true (1) if both operands are true (non-zero). In this case, a && b evaluates to 0 (false) because b is 0, which is considered false.
Logical OR (||):
- Operand 1: a (with a value of 10)
- Operand 2: b (with a value of 0)
- Result: The logical OR operator (||) evaluates to true (1) if at least one operand is true (non-zero). In this case, a || b evaluates to 1 (true) because a is 10, which is considered true.
Logical NOT (!):
- Operand A: a (with a value of 10)
- Operand B: b (with a value of 0)
- Result (a): The logical NOT operator (!) negates the value of the operand. In this case, !a evaluates to 0 (false) because a is initially true (non-zero), and the NOT operator flips it to false (0).
- Result (b): Similarly, !b evaluates to 1 (true) because b is initially false (0), and the NOT operator flips it to true (1).
So, in summary:
- a && b evaluates to 0 (false) because both operands are not true.
- a || b evaluates to 1 (true) because at least one operand is true.
- !a flips the value of a from true to false.
- !b flips the value of b from false to true.
output is:
1AND Result: 0
2OR Result: 1
3NOT Result (a): 0
4NOT Result (b): 1
Bitwise Operators
- Bitwise operators work at the bit level and are used to manipulate individual bits within data.
- They are especially useful when dealing with hardware or low-level operations. Common bitwise operators include:
- Bitwise AND (&): Performs a bitwise AND operation.
- Bitwise OR (|): Performs a bitwise OR operation.
- Bitwise XOR (^): Performs a bitwise XOR (exclusive OR) operation.
1#include <iostream>
2using namespace std;
3
4int main() {
5 int num1 = 5, num2 = 3;
6
7 // Bitwise AND
8 int andResult = num1 & num2;
9 cout << "Bitwise AND Result: " << andResult << endl;
10
11 // Bitwise OR
12 int orResult = num1 | num2;
13 cout << "Bitwise OR Result: " << orResult << endl;
14
15 // Bitwise XOR
16 int xorResult = num1 ^ num2;
17 cout << "Bitwise XOR Result: " << xorResult << endl;
18
19 return 0;
20}
output is :
1Bitwise AND Result: 1
2Bitwise OR Result: 7
3Bitwise XOR Result: 6
Assignment Operators
The assignment operator (=) is used to assign a value to a variable. It is one of the most basic operators in C++.
1// Example of the assignment operator
2int variable = 42;
3
Unary Operators
- Unary operators operate on a single operand.
- An example is the unary minus (-) operator, which negates a value.
1// Example of unary operator
2int number = 10;
3int negatedNumber = -number; // -10
4
Ternary or Conditional Operators
- The ternary operator (? :) is a concise way to write conditional statements.
- It evaluates a condition and returns one of two values based on whether the condition is true or false.
1#include <iostream>
2using namespace std;
3
4int main() {
5 int num = 10;
6 string result = (num > 5) ? "Greater" : "Smaller";
7 cout << "Result: " << result << endl;
8
9 return 0;
10}
output is :
1Result: Greater
Miscellaneous Operators
- There are several other operators in C++, such as the address-of operator (&) and the pointer dereference operator (*), which are often used in more advanced programming scenarios.
- However, for beginners, understanding the operators mentioned above is a great starting point.