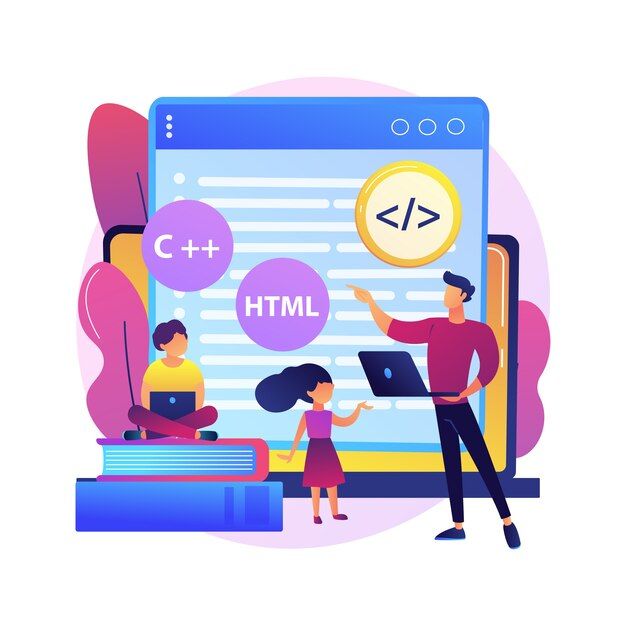
Static Member Function in C++
In C++, the static keyword is used to give special properties to variables, functions, or class members.
Static Member in C++
- In C++, a static data member is a class member variable that is associated with the class itself rather than with individual instances (objects) of the class.
- This means that all instances of the class share the same static data member.
- Static data members are declared using the static keyword within a class definition. for example:
1class MyClass {
2public:
3 static int staticVar; // Declaration of a static data member
4
5 MyClass() {
6 staticVar++;
7 }
8
9};
10
11int MyClass::staticVar = 0; // Initialization of the static data member
12
13int main() {
14 MyClass obj1;
15 MyClass obj2;
16 MyClass obj3;
17
18 // Accessing the static data member using the class name
19 cout << "Number of objects created: " << MyClass::staticVar << endl;
20
21 return 0;
22}
Static data members are useful when you need to maintain shared data or count instances of a class across all objects.
Static Member Function in C++
- In C++, a static member function is a member function of a class that is associated with the class itself rather than with individual instances (objects) of the class.
- This means that static member functions are not tied to any specific object, and they can be called using the class name, without the need to create instances of the class. for example
1class MathUtility {
2public:
3 static int add(int a, int b) {
4 return a + b;
5 }
6};
7
8int main() {
9 int sum = MathUtility::add(5, 3); // Calling a static member function
10
11 cout << "Sum: " << sum << endl;
12
13 return 0;
14}
- We define a class called MathUtility with a single static member function named add. This static member function takes two integer parameters, a and b, and returns their sum (a + b).
- In the main function, we start by declaring an integer variable sum.
- We then call the static member function MathUtility::add(5, 3) to calculate the sum of two integers, 5 and 3.
- The result of the MathUtility::add function call (which is the sum of 5 and 3, i.e., 8) is assigned to the sum variable.
Characteristics of Static Member Function in C++
- Static member functions are associated with the class and not with any particular object of the class.
- They can be thought of as "class-level" functions rather than "object-level" functions.
- Similar to static data members, static member functions can access and modify private static data members of the class.
- Static member functions are commonly used for utility functions that are related to the class but don't require access to instance-specific data.
Conclusion
Static member functions in C++ serve as class-level functions that are not tied to individual objects but rather associated with the class itself. They provide a way to encapsulate functionality related to the class without the need for instance-specific data.