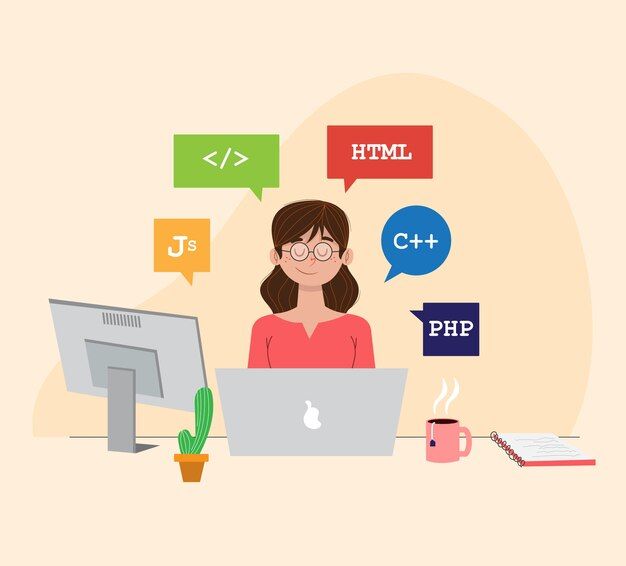
Virtual Function in C++
What is Virtual Function in C++
In C++, a Virtual function is a class member function declared using virtual keyword in the base class and can be overridden by derived classes.
Rules for Virtual Functions in C++
- Base classes declare virtual functions with the virtual keyword.
- Derived classes override these functions using the override keyword to match the base class.
- In C++, virtual functions are an essential feature for achieving runtime polymorphism and dynamic binding.
C++ Virtual Function Syntax
The syntax for declaring and using virtual functions in C++ is as follows:
Declaration in the Base Class
To declare a virtual function in the base class, use the virtual keyword before the function declaration:
1class Base {
2public:
3 virtual returnType functionName(parameters);
4};
- returnType: The return type of the virtual function.
- functionName: The name of the virtual function.
- parameters: The parameters (if any) that the virtual function takes.
Override in Derived Class
In the derived class, override the virtual function using the override keyword :
1class Derived : public Base {
2public:
3 returnType functionName(parameters) override {
4 // New implementation in the derived class
5 }
6};
- returnType: The return type of the overridden function should match the return type declared in the base class.
- functionName: The name of the virtual function, which should match the base class's function name.
- parameters: The parameters of the virtual function should match those in the base class.
Calling Virtual Functions
To call a virtual function through a base class pointer or reference, simply use the pointer or reference as if it were an object of the base class:
1Base* ptr = new Derived();
2ptr->functionName(parameters);
- ptr: A pointer to the base class that points to an object of the derived class.
- functionName(parameters): Call the virtual function as if it were a member function of the base class.
C++ Virtual Function Example
Let's understand the C++ Virtual function with an example :
1#inclue <iostream>
2using namespace std;
3
4// Base class with a virtual function
5class Animal {
6public:
7 virtual void speak() {
8 cout << "Animal makes a sound." << endl;
9 }
10};
11
12// Derived class 1
13class Dog : public Animal {
14public:
15 void speak() override {
16 cout << "Dog barks." << endl;
17 }
18};
19
20// Derived class 2
21class Cat : public Animal {
22public:
23 void speak() override {
24 cout << "Cat meows." << endl;
25 }
26};
27
28int main() {
29 Animal* myAnimal;
30 Dog dog;
31 Cat cat;
32
33 myAnimal = &dog;
34 myAnimal->speak(); // Calls Dog's speak()
35
36 myAnimal = &cat;
37 myAnimal->speak(); // Calls Cat's speak()
38
39 return 0;
40}
- We have a base class Animal with a virtual function speak().
- We also have two derived classes: Dog and Cat, both of which override the speak() function with their own implementations.
- In the main() function, we create objects of the Dog and Cat classes.
- We use a pointer myAnimal of type Animal* to point to different objects (dog and cat) of derived classes.
C++ Overirde Identifier
- In C++, the override identifier keyword was introduced in C++11 and later versions of the language.
- The override keyword in C++ enhances the clarity of code when dealing with virtual functions and function overriding.
- Here are the key uses of the override keyword:
- Clearly indicates the intent to override a virtual function.
- Ensures a match between the base and derived class function signatures.
- Helps catch errors at compile time.
- Enhances code readability and documentation.
Limitations of Virtual Functions
- Slight performance overhead.
- Not suitable for all functions.
- Limited support for operator overloading.
- Complexity in inheritance hierarchies.
- Incompatibility with certain programming techniques.
Conclusion
Virtual functions in C++ are a fundamental feature that enables polymorphism and dynamic binding. They allow derived classes to provide their own implementations of functions defined in a base class.