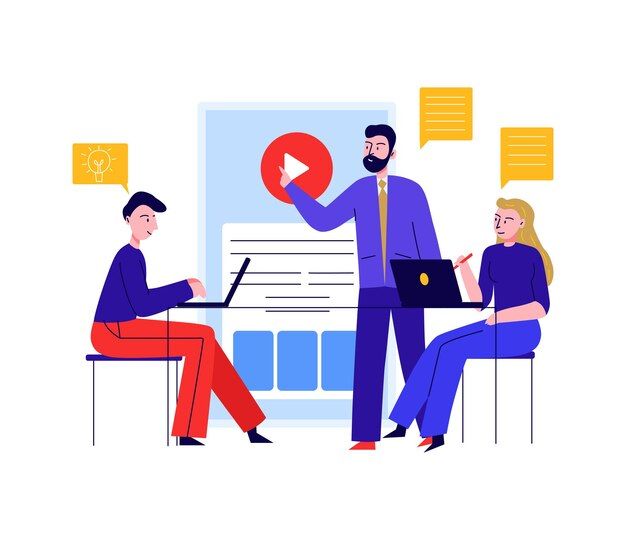
Pure Virtual function and Abstract Class in C++
What is Abstract Class in C++?
- An abstract class in C++ is a class that cannot be instantiated directly, meaning you cannot create objects of an abstract class.
- Instead, it serves as a template for other classes by defining a common interface and behavior that derived classes must adhere to.
Key Characteristics of an Abstract Class in C++
Pure Virtual Function
- Pure virtual function is a special type of virtual function that is declared in an abstract base class but has no implementation in that class.
- An abstract class typically contains one or more pure virtual functions, which are declared using the virtual keyword followed by = 0.
- These functions have no implementation in the abstract class and must be implemented by any class that derives from it.
1class AbstractClass {
2public:
3 virtual void pureVirtualFunction() = 0;
4 // declaration of Pure virtual function
5};
6
Inability to Instantiate
You cannot create objects of an abstract class. Attempting to do so will result in a compilation error.
1AbstractClass obj; // Error: Cannot create an instance of an abstract class
2
Derived Class Implementation
- Derived classes that inherit from an abstract class must provide concrete implementations for all pure virtual functions defined in the abstract base class.
- Not adhering to this will lead to a compilation error.
Abstract Class in C++ Syntax
1class AbstractClassName {
2public:
3 // Pure virtual function(s)
4 virtual returnType functionName(parameters) = 0;
5
6 // Other member functions and member variables
7 // ...
8};
- class AbstractClassName: Defines the abstract class with a specific name (AbstractClassName in this example).
- public: Specifies the access control for the class members.
- virtual returnType functionName(parameters) = 0: Declares a pure virtual function within the abstract class.
- The = 0 at the end of the declaration indicates that this is a pure virtual function with no implementation.
Abstract Class in C++ Example
let's understand abstract class with an example:
1#include <iostream>
2
3using namespace std; // Avoids using std:: prefix
4
5// Abstract base class with a pure virtual function
6class Shape {
7public:
8 // Pure virtual function to calculate area
9 virtual double calculateArea() const = 0;
10
11 // Non-pure virtual function
12 void displayInfo() {
13 cout << "This is a shape." << endl;
14 }
15};
16
17// Derived class: Circle
18class Circle : public Shape {
19private:
20 double radius;
21
22public:
23 Circle(double r) : radius(r) {}
24
25 // Override the pure virtual function to provide a concrete implementation
26 double calculateArea() const override {
27 return 3.14159 * radius * radius;
28 }
29};
30
31// Derived class: Rectangle
32class Rectangle : public Shape {
33private:
34 double length;
35 double width;
36
37public:
38 Rectangle(double l, double w) : length(l), width(w) {}
39
40 // Override the pure virtual function to provide a concrete implementation
41 double calculateArea() const override {
42 return length * width;
43 }
44};
45
46int main() {
47 // Create instances of derived classes
48 Circle circle(5.0);
49 Rectangle rectangle(4.0, 6.0);
50
51 // Declare a pointer to the base class
52 Shape* shapePtr;
53
54 // Point the base class pointer to a Circle object
55 shapePtr = &circle;
56
57 // Call the calculateArea() function through the base class pointer
58 cout << "Circle Area: " << shapePtr->calculateArea() << endl;
59
60 // Point the base class pointer to a Rectangle object
61 shapePtr = &rectangle;
62
63 // Call the calculateArea() function through the base class pointer
64 cout << "Rectangle Area: " << shapePtr->calculateArea() << endl;
65
66
67 return 0;
68}
- Shape is an abstract base class with a pure virtual function calculateArea(). Objects of the Shape class cannot be instantiated.
- Two derived classes, Circle and Rectangle, inherit from Shape and provide concrete implementations of the calculateArea() function.
Constructor in Abstract class?
- Yes, an abstract class in C++ can have a constructor. Abstract classes can have constructors just like any other class.
- When you create an object of a derived class, the constructor of the abstract base class is automatically called to set up the base class part of the object. for example
1#include <iostream>
2
3class AbstractClass {
4public:
5 AbstractClass(int value) : someValue(value) {
6 // Constructor implementation
7 }
8
9 virtual void pureVirtualFunction() = 0;
10
11 void displayInfo() {
12 std::cout << "This is a shape with value: " << someValue << std::endl;
13 }
14
15private:
16 int someValue;
17};
18
19class ConcreteClass : public AbstractClass {
20public:
21 ConcreteClass(int value) : AbstractClass(value) {
22 // Derived class constructor
23 }
24
25 void pureVirtualFunction() override {
26 // Implementation of the pure virtual function
27 }
28};
29
30int main() {
31 ConcreteClass concreteObj(42);
32 concreteObj.displayInfo();
33
34 return 0;
35}
In this example, both the abstract class AbstractClass and the concrete-derived class ConcreteClass have constructors.
Conclusion
Abstract classes act as blueprints, guiding the structure of derived classes, while pure virtual functions serve as placeholders for functionality that must be implemented by those derived classes.
Key takeaways:
- Abstract classes cannot be instantiated and are meant to be inherited from.
- Pure virtual functions have no implementation in the abstract class and must be implemented by derived classes.
- Abstract classes and pure virtual functions promote code modularity, extensibility, and polymorphism in object-oriented programming.