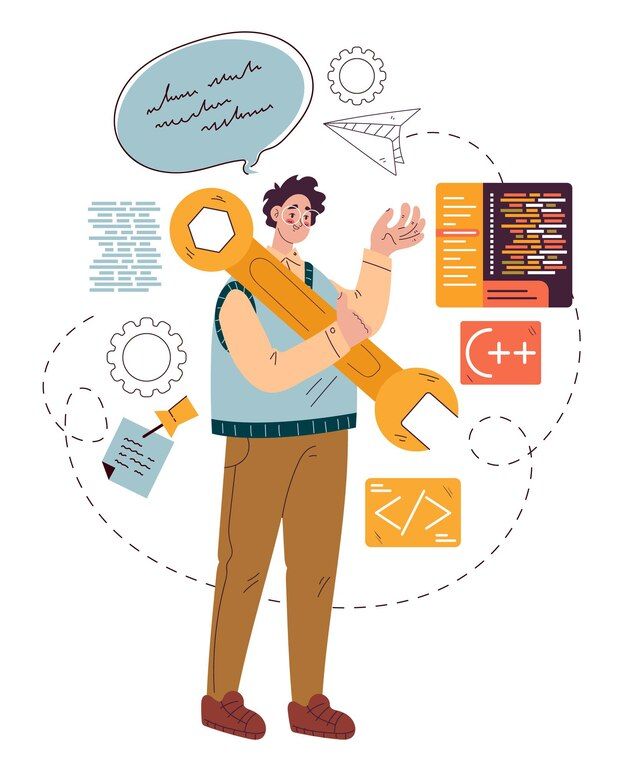
Functions in C++
- functions are self-contained blocks of code that perform a specific task.
- Functions in C++ allow you to break down your code into smaller, manageable parts.
C++ function Syntax
1return_type function_name(parameter_type1 parameter_name1, parameter_type2 parameter_name2, ...) {
2 // Function body
3 // Code to perform a specific task
4 return value_to_return; // (if the function has a return type)
5}
- Now, let's break down each part of the syntax:
- return_type: This is the data type that specifies what the function will return when it's called.
- It can be any valid C++ data type (e.g., int, double, char), or it can be void if the function doesn't return any value.
- function_name: Replace the desired name you choose for your function.
- It should follow the rules for valid C++ identifiers and be unique within your program's scope.
- parameter_typeX: These are the data types of the parameters that the function accepts.
- You can omit this part if the function doesn't take any parameters.
- parameter_nameX: These are the names you give to the parameters. You can omit this part if the function doesn't take any parameters.
- // Function body: This is where you place the code that defines what the process does. It is enclosed in curly braces { }.
- return value_to_return If the function has a return type other than void, you use the return statement to specify the value to be returned.
Here's a simple example of a C++ function:
1#include <iostream>
2using namespace std;
3
4int add(int a, int b) {
5 int sum = a + b;
6 return sum;
7}
8
9int main() {
10 int result = add(4, 4);
11 cout << "The result is: " << result << endl;
12 return 0;
13}
14
In this example, we have a function named add that takes two integer parameters (a and b), calculates their sum, and returns the result as an integer.
Why do we need functions?
- Each function performs a specific task, making the code more organized and easier to understand.
- Functions enable you to write a piece of code once and reuse it in multiple places within your program.
- Functions provide a level of abstraction, allowing you to use complex functionality without needing to understand how it works internally.
Types of functions
- User Defined Functions
- Built-in Functions
User Defined Functions
- User-defined functions are functions that you create yourself in your C++ program.
- Examples:
- Creating a function to calculate the average of a list of numbers.
- Writing a function to print a custom message to the console.
Built-In Functions
- Built-in functions, or standard library functions, are functions provided by the C++ Standard Library.
- They are part of the C++ language and are available for you to use without having to write their code.
- Examples:
- cout and cin for console input/output.
- sqrt() for square root calculation.
- strlen() for string length calculation.
Main Function
- The main() function in C++ is a special function that serves as the entry point for a C++ program.
- When you run a C++ program, the operating system starts executing the code from the main() function.
Here's what you need to know about the main() function:
Function Signature
The main() function has a specific signature, which looks like this:
1int main() {
2 // Code for your program goes here
3 return 0; // Optional, indicates a successful program execution
4}
Return Type
- The main() function typically has a return type of int.
- This return type allows the program to communicate its exit status to the operating system.
- A return value of 0 conventionally indicates a successful execution, while a non-zero value can be used to signal errors
Parameters
- The main() function can also accept command-line arguments as parameters.
- These are provided when you run the program from the command line.
Execution
- The code within the main() function is executed sequentially from top to bottom when you run your program.
- This is where you write the main logic of your program, including any input/output operations, calculations, and function calls.
Here's a simple example :
1#include <iostream>
2using namespace std;
3
4int main() {
5 cout << "Hello, World!" << endl;
6 return 0; // Indicates successful execution
7}
- In this example, the main() function prints "Hello, World!" to the console and returns 0, indicating a successful program run.
- The #include directive is used to include the iostream header, which provides input/output functionality.
C++ Passing Array to Function
1return_type function_name(data_type array_name[], int array_size) {
2 // Function body
3 // Access and manipulate elements of the array using array_name
4}
Inside the function body, you can access and manipulate the elements of the array using the array_name and perform any operations you need.
Function Overloading
- Function overloading allows you to define multiple functions in the same scope with the same name but different parameter lists.
- The appropriate function to be called is determined by the number or types of arguments provided during the function call.
1#include <iostream>
2using namespace std;
3
4// Function overloading examples
5int add(int a, int b) {
6 return a + b;
7}
8
9double add(double a, double b) {
10 return a + b;
11}
12
13string add(string a, string b) {
14 return a + b;
15}
16
17int main() {
18 cout << add(2, 3) << endl;
19 cout << add(2.5, 3.5) << endl;
20 cout << add("Hello, ", "world!") << endl;
21 return 0;
22}
- In this example, the add function is overloaded three times with different parameter types (int, double, and string).
Types of Functions in C++
- Void Function
- Functions with return value
- Parameterized Function
- Recursive Function
- Inline Function
- Member Function
- Static Member Function
- Friend Function
Void Function
- These functions don't return any value.
- They are often used to perform a task or operation without needing to provide a result. for example
1void printMessage() {
2 cout << "Hello, World!";
3}
Functions with Return Values
These functions return a value or result after performing a task.
1int add(int a, int b) {
2 return a + b;
3}
Parameterized Function
- These functions accept input values called parameters or arguments.
- They use these values to perform operations.
1void greet(string name) {
2 cout << "Hello, " << name << "!";
3}
C++ Recursive Function
- These functions call themselves within their own definition.
- They are often used for solving problems that can be broken down into smaller, similar sub-problems.
1int factorial(int n) {
2 if (n <= 1)
3 return 1;
4 else
5 return n * factorial(n - 1);
6}
7
C++ Inline Function
- In C++, an inline function is a special type of function that is used to optimize code by avoiding the overhead associated with regular function calls.
- Inline functions are typically small and are expanded in place during compilation.
- When you declare a function as inline using the inline keyword, you are suggesting to the compiler that it should replace the function call with the actual code of the function.
- The compiler will attempt to insert the code of the inline function directly at the call site. for example
1inline int multiply(int a, int b) {
2 return a * b;
3}
Member Function
- A member function in C++ is a function that is associated with a class.
- It is defined within the class and can operate on the data members of that class.
- Member functions are a fundamental part of encapsulation in object-oriented programming.
1class Calculator {
2private:
3 int result; // Data member
4
5public:
6 Calculator() { // Constructor
7 result = 0;
8 }
9
10 void add(int number) { // Member function
11 result += number;
12 }
13
14 void subtract(int number) { // Member function
15 result -= number;
16 }
17
18 int getResult() { // Member function
19 return result;
20 }
21};
22
23int main() {
24 Calculator calc; // Create an instance of the Calculator class
25
26 calc.add(5); // Call the 'add' member function
27 calc.subtract(3); // Call the 'subtract' member function
28
29 int finalResult = calc.getResult(); // Call the 'getResult' member function
30
31 return 0;
32}
Static Member Function
- A static member function is a special type of member function that belongs to a class rather than an instance of the class.
- You declare a static member function using the static keyword within the class definition.
1class Math {
2public:
3 static int square(int num) {
4 return num * num;
5 }
6};
Friend Functions
- Friend functions are not members of a class but have access to the private and protected members of the class.
- They are typically used for operations that need to access class internals but are not part of the class itself.
1class MyClass {
2 int data;
3public:
4 friend void modifyData(MyClass &obj);
5};
6
7void modifyData(MyClass &obj) {
8 obj.data = 42;
9}
Conclusion
These are some of the common types of functions in C++. The choice of which type to use depends on the specific task and requirements of your program.