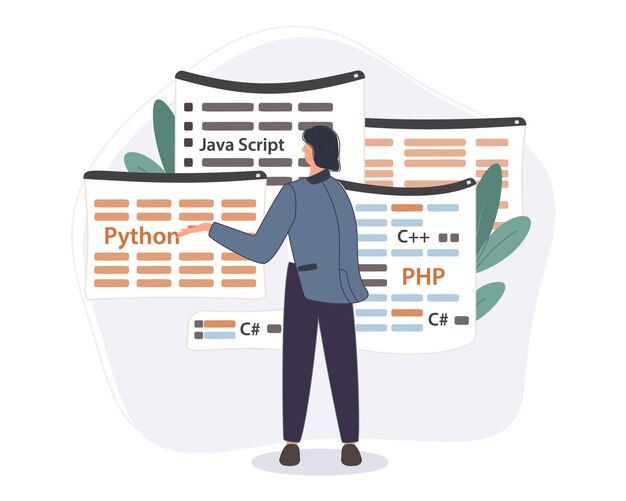
Constructors in C++
- Constructor in C++ is a special member function used to initialize an object when it is created.
- Constructors have the same name as the class and do not have a return type.
- Constructors are automatically invoked when an object is created.
- In C++, a class can have multiple constructors, each with a different set of parameters.
Constructors in C++
- Default Constructor
- Parameterized Constructor
- Copy Constructor
C++ Default Constructor
- A default constructor is a special constructor in C++ that doesn't take any arguments.
- Default constructors are automatically called when you create an object of a class.
1#include <iostream>
2using namespace std;
3
4class Person {
5public:
6 // Default constructor
7 Person() {
8 name = "Unknown";
9 age = 0;
10 }
11
12 void displayInfo() {
13 cout << "Name: " << name << ", Age: " << age << endl;
14 }
15
16private:
17 string name;
18 int age;
19};
20
21int main() {
22 Person person; // Calls the default constructor
23 person.displayInfo();
24
25 return 0;
26}
- The Person class has a default constructor, which initializes the name to "Unknown" and age to 0.
- When we create an object person without providing any arguments, the default constructor is automatically called,
C++ Parameterized Constructor
- A parameterized constructor is a special constructor that takes one or more arguments.
- Its purpose is to initialize an object with specific values for its attributes when it is created.
1#include <iostream>
2using namespace std;
3
4class Person {
5public:
6 // Parameterized constructor
7 Person(string n, int a) {
8 name = n;
9 age = a;
10 }
11
12 void displayInfo() {
13 cout << "Name: " << name << ", Age: " << age << endl;
14 }
15
16private:
17 string name;
18 int age;
19};
20
21int main() {
22 Person person("Alice", 25); // Calls the parameterized constructor
23 person.displayInfo();
24
25 return 0;
26}
- The Person class has a parameterized constructor that takes two arguments: n (for the name) and a (for the age).
- When we create an object person and provide values "Alice" and 25 as arguments, the parameterized constructor is called, and the object's attributes are initialized with these specific values.
C++ Copy Constructor
- A copy constructor is a special constructor that initializes an object using another object of the same class.
- It is used when objects are passed by value or when new objects are created as copies of existing ones.
1#include <iostream>
2using namespace std;
3
4class Person {
5public:
6 // Constructor
7 Person(string name) {
8 this->name = name;
9 }
10
11 // Copy constructor
12 Person(const Person& other) {
13 this->name = other.name;
14 cout << name << " is copied!" << endl;
15 }
16
17private:
18 string name;
19};
20
21int main() {
22 Person alice("Alice"); // Calls constructor
23 Person copyOfAlice = alice; // Calls copy constructor
24
25 return 0;
26}
27
- We have a copy constructor in the Person class that creates a copy of another Person object.
- When copyOfAlice is initialized with Alice, the copy constructor is called, creating a new object with the same attributes.
What is Constructor Overloading?
Constructor overloading in C++ allows you to define multiple constructors within a class, each with a different set of parameters. for example:
1#include <iostream>
2using namespace std;
3
4class Person {
5public:
6 // Default constructor
7 Person() {
8 name = "Unknown";
9 age = 0;
10 }
11
12 // Parameterized constructor with two arguments
13 Person(string n, int a) {
14 name = n;
15 age = a;
16 }
17
18 // Parameterized constructor with one argument
19 Person(string n) {
20 name = n;
21 age = 0;
22 }
23
24 void displayInfo() {
25 cout << "Name: " << name << ", Age: " << age << endl;
26 }
27
28private:
29 string name;
30 int age;
31};
32
33int main() {
34 Person person1; // Calls the default constructor
35 Person person2("Alice", 25); // Calls the parameterized constructor with two arguments
36 Person person3("Bob"); // Calls the parameterized constructor with one argument
37
38 person1.displayInfo();
39 person2.displayInfo();
40 person3.displayInfo();
41
42
43 return 0;
44}
- When you create person1, it uses the default constructor with no arguments.
- When you create person2, it uses the parameterized constructor with two arguments to set both name and age.
- When you create person3, it uses the parameterized constructor with one argument to set only the name while age defaults to 0.
What are the characteristics of a constructor?
- Name matches class.
- No return type.
- Automatically invoked.
- Initializes object.
- Can have parameters.
- Multiplicity with overloading.
- Access specifier (public).
- Default constructor provided if none.
What distinguishes constructors from a typical member function?
Name and Purpose
- Constructors have the same name as the class and are used to initialize objects of that class when they are created.
- Typical Member Functions have names that describe their purpose, and they perform various operations on objects after they have been initialized by a constructor.
Automatic Invocation
- Constructors are automatically invoked or called when an object is created.
- Typical Member Functions need to be called explicitly,
Return Type
- Constructors do not have a return type specified, not even void. They initialize the object's state but do not return any value.
- Typical Member Functions can have a return type, and they often return values.
What is Destructor?
- Destructor is a special member function used to clean up resources when an object is destroyed.
- It has the same name as the class preceded by a tilde (~) and does not take any parameters.
1#include <iostream>
2using namespace std;
3
4class Person {
5public:
6 // Constructor
7 Person(string name) {
8 this->name = name;
9 cout << name << " is born!" << endl;
10 }
11
12 // Destructor
13 ~Person() {
14 cout << name << " is gone!" << endl;
15 }
16
17private:
18 string name;
19};
20
21int main() {
22 Person person("Alice"); // Calls constructor
23
24// Destructor is automatically called when the object goes out of scope
25 return 0;
26}
- We have a class Person with a constructor and a destructor.
- The constructor initializes the name attribute.
- The destructor is automatically called when the object person goes out of scope and prints a message.