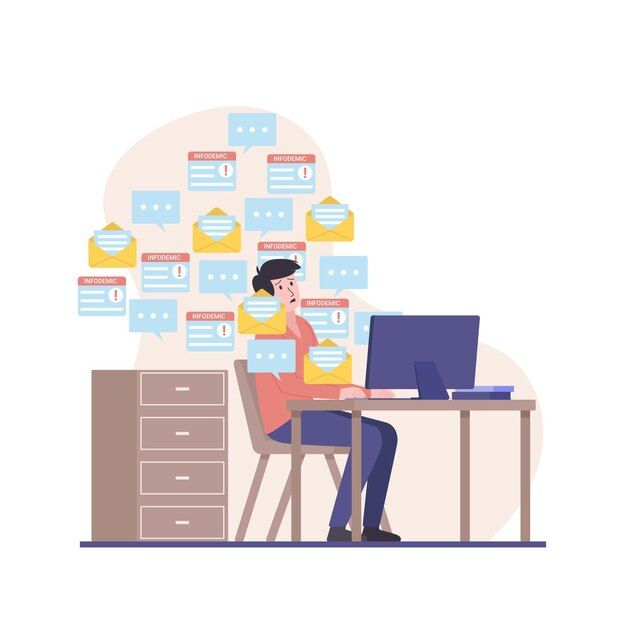
Operator Overloading in C++
What is Operator Overloading?
- Operator overloading in C++ allows you to define custom behaviors for operators when applied to objects of user-defined classes.
- In other words, it enables you to give new meanings to operators, beyond their standard operations on built-in data types.
Operator Overloading in C++ Syntax
Operator overloading in C++ follows a specific syntax. Here's the general syntax for overloading operators:
1return_type operator OPERATOR(parameters) {
2 // Implementation of the operator's behavior
3}
- return_type: This is the data type of the result that the operator will produce when it's applied to objects of your class.
- operator: The keyword operator followed by the specific operator you want to overload. For example, +, -, ==, !=, <<, etc.
- OPERATOR: Replace this with the actual operator you want to overload, such as +, -, ==, !=, <<, etc.
- parameters: These are the parameters that the overloaded operator function takes.
- The parameters depend on the operator being overloaded. For binary operators like + or -, etc.
Operator Functions vs. Normal Functions
Operator Functions
- Operator functions are special member functions used for overloading operators,
- while normal functions are regular functions used for various tasks.
Naming Conventions
- Operator functions have names like operator+, operator-, etc., and they have specific syntax and rules for overloading.
- Normal functions do not have these specific naming conventions or restrictions.
Can we overload all operators?
Not all operators can be overloaded. Some operators, like the scope resolution operator::, member access operator. and .*, and conditional operator? : cannot be overloaded. However, all other operators can be overloaded.
Rules for Operator Overloading
- Overloaded operators must have at least one user-defined type as an operand.
- Some operators must be overloaded as member functions of a class, while others can be overloaded as global functions.
- Operators retain their precedence and associativity unless changed explicitly.
Examples of Operator Overloading in C++
Unary Operator Overloading in C++
Unary operators are operators that work on a single operand. Let's overload the ++ and -- operators for a simple Counter class:
1#include <iostream>
2using namespace std;
3
4class Counter {
5public:
6 int count;
7
8 Counter(int initialCount) : count(initialCount) {}
9
10 // Overload the prefix increment operator ++
11 Counter operator++() {
12 count++;
13 return *this;
14 }
15
16 // Overload the prefix decrement operator --
17 Counter operator--() {
18 count--;
19 return *this;
20 }
21};
22
23int main() {
24 Counter counter(5);
25
26 ++counter; // Increment using overloaded operator
27 --counter; // Decrement using overloaded operator
28
29 cout << "Count: " << counter.count << endl;
30
31 return 0;
32}
In this example, we've overloaded the prefix increment (++) and decrement (--) operators for the Counter class, allowing us to increment and decrement the count member easily.
This Pointer in C++
- In C++, the "this" pointer is a special pointer that is automatically available within member functions of a class.
- It points to the object for which the member function is being called. Essentially, it refers to the current instance of the class.
Nameless Objects
Nameless objects are objects created without assigning them to a variable. They are often used for temporary calculations. Here's an example:
1#include <iostream>
2using namespace std;
3
4class Point {
5public:
6 int x;
7 int y;
8
9 Point(int a, int b) : x(a), y(b) {}
10
11 // Overload the + operator to add two points
12 Point operator+(const Point& other) {
13 return Point(x + other.x, y + other.y);
14 }
15};
16
17int main() {
18 Point result = Point(1, 2) + Point(3, 4); // Using nameless objects
19
20 cout << "Result: (" << result.x << ", " << result.y << ")" << endl;
21
22 return 0;
23}
- In this example, we create two Point objects without assigning them to variables and add them together using the overloaded + operator.
Binary Operator Overloading in C++
Binary operators work on two operands. Let's overload the + operator for a Complex class:
1#include <iostream>
2using namespace std;
3
4class Complex
5{
6public:
7 int real;
8 int imaginary;
9
10 Complex(int r, int i) : real(r), imaginary(i) {}
11
12 // Overload the + operator for complex number addition
13 Complex operator+(const Complex &other)
14 {
15 return Complex(real + other.real, imaginary + other.imaginary);
16 }
17};
18
19int main()
20{
21 Complex num1(3, 5);
22 Complex num2(2, 7);
23
24 Complex sum = num1 + num2; // Custom addition using overloaded operator
25
26 cout << "Sum: " << sum.real << " + " << sum.imaginary << "i" << endl;
27
28
29 return 0;
30}
- We define a Complex class to represent complex numbers with real and imaginary parts.
- We overload the + operator within the Complex class to add two complex numbers together.
- In the main function, we create two Complex objects, num1 and num2, with different values.
- We use the overloaded + operator to add num1 and num2, storing the result in the sum variable.
Operator overloading in C++ using the friend function
- Friend functions are non-member functions that are granted access to the private members of a class.
- Here's an example of a Point class with a friend function for addition:
1#include <iostream>
2using namespace std;
3
4class Point
5{
6private:
7 int x;
8 int y;
9
10public:
11 Point(int a, int b) : x(a), y(b) {}
12
13 // Declare a friend function for addition
14 friend Point operator+(const Point &p1, const Point &p2);
15
16 // Allow access to private members through a public function
17 int getX() const { return x; }
18 int getY() const { return y; }
19};
20
21// Define the friend function for addition
22Point operator+(const Point &p1, const Point &p2)
23{
24 return Point(p1.x + p2.x, p1.y + p2.y);
25}
26
27int main()
28{
29 Point result = Point(1, 2) + Point(3, 4); // Using a friend function for addition
30
31 cout << "Result: (" << result.getX() << ", " << result.getY() << ")" << endl;
32
33
34 return 0;
35}
In this example, we've defined a friend function operator+ that allows us to add two Point objects.
Conversion between Basic Types and User-Defined Types
- You can define conversion operators to convert between user-defined types and basic types.
- Here's an example where we convert a Distance class to an int:
1#include <iostream>
2
3class Distance {
4private:
5 int meters;
6
7public:
8 Distance(int m) : meters(m) {}
9
10 // Conversion operator to int
11 operator int() const {
12 return meters;
13 }
14};
15
16int main() {
17 Distance dist(100);
18 int meters = dist; // Conversion from Distance to int
19
20 std::cout << "Meters: " << meters << std::endl;
21
22
23 return 0;
24}
In this example, we've defined a conversion operator operator int() that allows us to convert a Distance object to an int.
Conclusion
Operator overloading in C++ empowers you to redefine the behavior of operators for user-defined classes.