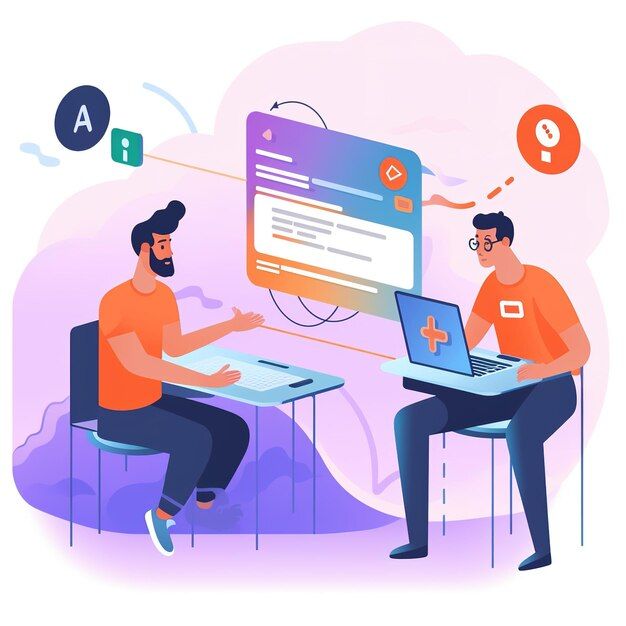
Friend Function in C++
What is Friend function in C++?
- In C++, a friend function is a function that is not a member of a class but is granted special access to the private and protected members of that class.
- A friend function is declared as a friend of the class using the friend keyword within the class definition.
Friend Function Syntax
Declaration of a friend function within a C++ class as follows:
1class YourClass {
2 friend return_type friendFunction(parameters);
3};
Characteristics of Friend function
- A friend function is not a member of the class it's associated with. It is defined outside the class.
- Friend functions are not inherited by derived classes. They are specific to the class in which they are declared as friends.
- Friend functions do not affect the member functions of a class.
C++ Friend Function Example
Example of a Friend Function:
1#include <iostream>
2using namespace std;
3
4class MyClass {
5private:
6 int data;
7
8public:
9 MyClass(int d) : data(d) {}
10
11 // Friend function declaration
12 friend void friendFunction(const MyClass&);
13
14 void display() {
15 cout << "Data: " << data << endl;
16 }
17};
18
19// Friend function definition
20void friendFunction(const MyClass& obj) {
21 cout << "Friend Function: Data = " << obj.data << endl;
22}
23
24int main() {
25 MyClass myObject(42);
26 myObject.display();
27
28 // Call the friend function
29 friendFunction(myObject);
30
31 return 0;
32}
- In this example:
- MyClass is a class with private member data.
- friendFunction is declared as a friend function within the MyClass class.
- Inside friendFunction, we can access and display the private member data of MyClass objects.
How to use friend function for two classes in C++?
let's see an example below:
1#include <iostream>
2using namespace std;
3
4class ClassB; // Forward declaration
5
6class ClassA {
7private:
8 int dataA;
9
10public:
11 ClassA(int d) : dataA(d) {}
12
13 // Friend function declaration for ClassB
14 friend void friendFunction(ClassA, ClassB);
15
16 void displayA() {
17 cout << "ClassA Data: " << dataA << endl;
18 }
19};
20
21class ClassB {
22private:
23 int dataB;
24
25public:
26 ClassB(int d) : dataB(d) {}
27
28 // Friend function declaration for ClassA
29 friend void friendFunction(ClassA, ClassB);
30
31 void displayB() {
32 cout << "ClassB Data: " << dataB << endl;
33 }
34};
35
36// Friend function definition
37void friendFunction(ClassA objA, ClassB objB) {
38 cout << "Friend Function: ClassA Data = " << objA.dataA << endl;
39 cout << "Friend Function: ClassB Data = " << objB.dataB << endl;
40}
41
42int main() {
43 ClassA objectA(10);
44 ClassB objectB(20);
45
46 objectA.displayA();
47 objectB.displayB();
48
49 // Call the friend function with objects of both classes
50 friendFunction(objectA, objectB);
51
52 return 0;
53}
- We have two classes, ClassA and ClassB, each with its own private data member (dataA and dataB, respectively).
- Both ClassA and ClassB declare the same friend function friendFunction using the friend keyword.
What is a friend class in C++?
- C++, a friend class is a class that is granted access to the private and protected members of another class.
- Here's an example of a friend class:
1#include <iotream>
2using namespace std;
3
4class FriendClass; // Forward declaration
5
6class MyClass {
7private:
8 int privateData;
9
10public:
11 MyClass(int data) : privateData(data) {}
12
13 friend class FriendClass; // Friend class declaration
14
15 void display() {
16 cout << "MyClass Private Data: " << privateData << endl;
17 }
18};
19
20class FriendClass {
21public:
22 void accessPrivateData(MyClass& obj) {
23 cout << "FriendClass accessing private data: " << obj.privateData << endl;
24 }
25};
26
27int main() {
28 MyClass myObject(42);
29 myObject.display();
30
31 FriendClass friendObj;
32 friendObj.accessPrivateData(myObject);
33
34 return 0;
35}
- We have two classes, MyClass and FriendClass.
- MyClass has a private data member privateData, and it declares FriendClass as a friend class using friend class FriendClass.
- This allows FriendClass to access the private members of MyClass.
What are forward declarations in C++?
- In C++, a forward declaration is a way to declare the existence of a class, function, or variable without providing its complete definition.
- It's essentially a way to tell the compiler that a certain entity exists and will be defined later in the code.
What is the difference between friend and member functions in C++?
- The key difference between friend and member functions in C++
- member functions are part of the class and have direct access to its private members,
- while friend functions are external to the class but can access its private members if explicitly declared as friends.
What is the difference between friend function and operator overloading?
- Friend Function: It can be a standalone function of another class but is not a member of the class it's providing access to.
- Operator Overloading: It typically involves defining member functions within the class, known as operator overloading functions.
- Friend Function: It can access and modify private members of a class by being explicitly declared as a friend.
- Operator Overloading: It can access and modify private members of a class if the operator overloading function is a member of that class.
Conclusion
A friend function in C++ is a non-member function that is granted special access to the private and protected members of a class. It is declared as a friend within the class, allowing it to work with the class's private data.