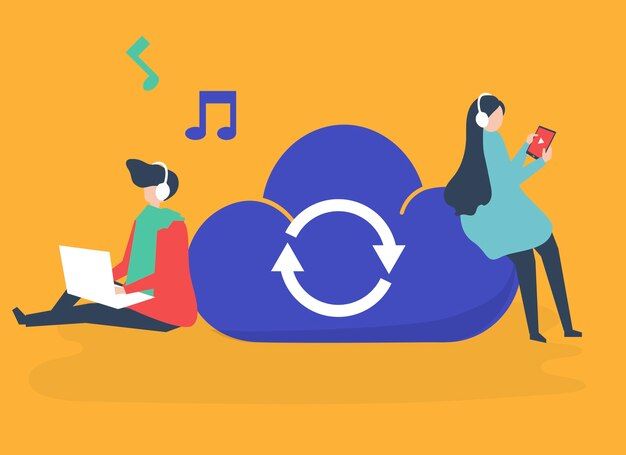
Aggregation in C++
- Aggregation in C++ is a relationship between two classes where one class, called the "aggregator" or "container" class, contains an instance of another class, called the "aggregated" or "contained" class.
- Aggregation represents a "has-a" relationship, where one class contains or is composed of another class as a part.
Key characteristics of aggregation in C++
- Ownership: In an aggregation relationship, the aggregator class owns the aggregated class, meaning that the lifetime of the contained object is managed by the aggregator.
- Multiplicity: Aggregation can represent one-to-one, one-to-many, or many-to-one relationships. For example, a university "has" many students (one-to-many), or a computer "has" a keyboard (one-to-one).
- Navigation: The aggregator class can access and manipulate the contained class's members and functions.
Aggregation in C++ Example
1#include <iostream>
2using namespace std;
3
4// Define a class called Tail
5class Tail {
6public:
7 // Member function to wag the tail
8 void wag() {
9 cout << "Tail is wagging." << endl;
10 }
11};
12
13// Define a class called Dog
14class Dog {
15public:
16 // Constructor for Dog
17 Dog() {}
18
19 // Member function to make the dog bark
20 void bark() {
21 cout << "Dog is barking." << endl;
22 }
23
24 // Member function to make the dog wag its tail
25 void wagTail(Tail& tail) {
26 tail.wag(); // Calls the wag() function of the Tail object
27 }
28};
29
30int main() {
31 // Create an instance of the Dog class called myDog
32 Dog myDog;
33
34 // Create an instance of the Tail class called myTail
35 Tail myTail;
36
37 // Call the bark() function of the Dog class
38 myDog.bark(); // Outputs "Dog is barking."
39
40 // Call the wagTail() function of the Dog class and pass myTail as an argument
41 myDog.wagTail(myTail); // Outputs "Tail is wagging."
42
43 return 0;
44}
This code demonstrates aggregation, where the Dog class contains a Tail object, and it can interact with and use the behavior of that Tail object through function calls.
Conclusion
Aggregation in C++ represents a relationship where one class contains another class as a part, creating a "has-a" association. It allows objects of one class to utilize the services or properties of another class without direct ownership.