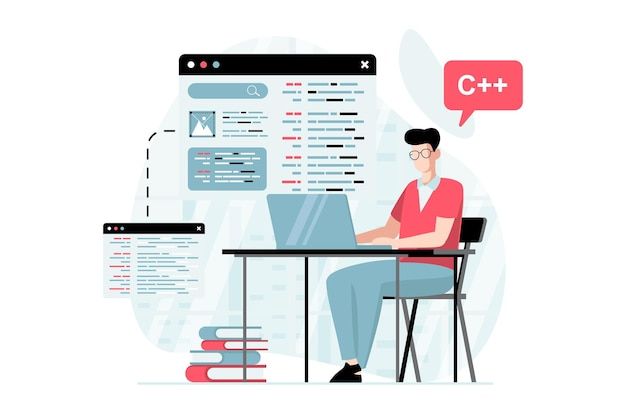
Call by value and call by reference in C++
- Call by value and call by reference are two different ways to pass arguments to functions in C++.
- Call by value works with copies of arguments while call by reference uses references to the original data allowing direct modifications.
Call by value in C++
- In Call by value, function parameters receive copies of the arguments passed to them.
- The function works with these copies and cannot modify the original data.
- Inside the function, any changes made to the parameter won't affect the original variable outside the function.
- This is like making a photocopy of a document: you can write on the copy, but it doesn't change the original.
1#include <iostream>
2using namespace std;
3
4void incrementValue(int x) {
5 x++; // Modifying the local parameter
6}
7
8int main() {
9 int num = 5;
10 incrementValue(num); // Call by value
11 cout << "Original value: " << num << endl; // Output: Original value: 5
12 return 0;
13}
- In this example, the incrementValue function takes an integer parameter x.
- When we call the function with incrementValue(num), it receives a copy of num.
- Inside the function, x is incremented, but this does not affect the original num variable.
Call by reference in C++
- In Call by reference, function parameters receive references to the original arguments.
- The function can directly modify the original data.
- Any modifications made to the parameters within the function directly affect the original arguments. for example
1#include <iostream>
2using namespace std;
3
4// Function to swap two numbers using call by reference
5void swapByReference(int &a, int &b) {
6 int temp = a;
7 a = b;
8 b = temp;
9}
10
11int main() {
12 int num1, num2;
13
14 cout << "Enter the first number: ";
15 cin >> num1;
16
17 cout << "Enter the second number: ";
18 cin >> num2;
19
20 cout << "Before swapping:" << endl;
21 cout << "First number: " << num1 << endl;
22 cout << "Second number: " << num2 << endl;
23
24 // Call the swapByReference function to swap the values
25 swapByReference(num1, num2);
26
27 cout << "After swapping:" << endl;
28 cout << "First number: " << num1 << endl;
29 cout << "Second number: " << num2 << endl;
30
31 return 0;
32}
- We define a function swapByReference that takes two integer parameters a and b by reference.
- Inside the swapByReference function, we use a temporary variable temp to swap the values of a and b.
- In the main function, we input two numbers from the user and display them before swapping.
- We then call the swapByReference function, passing num1 and num2 as arguments by reference, which means the function will directly modify its values.
- Finally, we display the values of the two numbers after swapping, showing that the values have been successfully swapped using call by reference.
Difference Between Call by Value and Call by Reference
Parameter Copies
- Call by value makes copies of the arguments for the function.
- Call by reference uses references to the original data.
Data Modification
- Call by value cannot modify the original data.
- Call by reference can modify the original data.
Data Integrity
- Call by value preserves the integrity of original data.
- Call by reference allows direct modification of data.
Function Signature
- Call by value functions lack the & symbol in parameter declarations.
- Call by reference functions include the & symbol in parameter declarations.
Call by Pointer in C++
- Call by pointer is another parameter passing mechanism in C++ similar to call by reference.
- However, instead of passing references to data, it involves passing pointers to data.
- In Call by pointer, function parameters receive pointers (memory addresses) to the original data.
- The function can access and modify the original data indirectly through these pointers. For example
1#include <iostream>
2using namespace std;
3
4// Function that swaps the values using call by pointer
5void swapByPointer(int *a, int *b) {
6 int temp = *a; // Dereference to access the value pointed to by 'a'
7 *a = *b; // Assign the value pointed to by 'b' to 'a'
8 *b = temp; // Assign the temporary value to 'b'
9}
10
11int main() {
12 int num1 = 5;
13 int num2 = 10;
14
15 cout << "Before swapping:" << endl;
16 cout << "First number: " << num1 << endl;
17 cout << "Second number: " << num2 << endl;
18
19 // Call the swapByPointer function and pass pointers to 'num1' and 'num2'
20 swapByPointer(&num1, &num2); // Pass memory addresses as arguments
21
22 cout << "After swapping:" << endl;
23 cout << "First number: " << num1 << endl;
24 cout << "Second number: " << num2 << endl;
25
26 return 0;
27}
- We define the swapByPointer function, which takes two integer pointers int *a and int *b as parameters.
- Inside the swapByPointer function, we dereference the pointers to access and modify the values pointed to by a and b, effectively swapping their values.
- In the main function, we have two integer variables num1 and num2.
- We call the swapByPointer function, passing the memory addresses of num1 and num2 using the & operator.
- After the function call, we print the values of num1 and num2 to observe that their values have been successfully swapped using call by pointer.
Call by value in C++ using Class
Let's understand with an example :
1#include <iostream>
2using namespace std;
3
4class Swap
5{
6
7public:
8 int a, b, swap;
9
10 void getData()
11 {
12 cout << "enter the value of a \n";
13 cin >> a;
14 cout << " \nenter the value of b \n";
15 cin >> b;
16 }
17
18 void SwapByValue()
19 {
20 swap = a;
21 a = b;
22 b = swap;
23 }
24
25 void DisplayData()
26 {
27 cout << "swaped value of a is "
28 << a;
29 cout << " swaped value of b is "
30 << b;
31 }
32};
33
34int main()
35{
36 Swap s;
37 s.getData();
38 s.SwapByValue();
39 s.DisplayData();
40}
- The getData member function is used to input values for a and b from the user.
- The SwapByValue member function swaps the values of a and b using a temporary variable swap.
- The DisplayData member function displays a and b.
- In the main function:
- An object s of the Swap class is created.
- The getData method is called to input values for a and b.
- The SwapByValue method is called to perform the swapping using "call by value" within the class.
- The DisplayData method is called to display the swapped values.
Call by Reference using Class
Let's understand with an example :
1// call by reference using class
2
3#include <iostream>
4using namespace std;
5class Swap
6{
7
8 // int a, b;
9
10 // priavte , protected
11public:
12 void swapReference(int &a, int &b)
13 {
14 int temp = a;
15 a = b;
16 b = temp;
17 }
18};
19int main()
20{
21
22 Swap s;
23 int x, y;
24
25 cout << " Please enter the value of x - ";
26 cin >> x;
27
28 cout << "\n Please enter the value of y - ";
29 cin >> y;
30
31 s.swapReference(x, y);
32 cout << " \n swapped value of x is "
33 << x;
34 cout << "\n swapped value of y is "
35 << y;
36
37 return 0;
38}
- The code defines a class Swap with a swapReference function.
- In main, it takes two integer inputs x and y.
- It calls the swapReference function of the Swap class to swap the values of x and y.
- Finally, it prints the swapped values of x and y.
Conclusion
Call by Value Involves passing copies of arguments to functions. Call by Reference Involves passing references to the original data to functions.