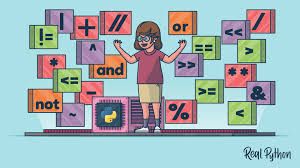
Operators in Python
Relational Operators in Python
- Relational operators in Python are used to compare two values or expressions and determine their relationship or relative order.
- These operators return a Boolean value (True or False) based on the comparison result.
- Relational operators help you make decisions and control the flow of your program by evaluating conditions.
- Here are the commonly used relational operators:
Greater Than (>): Checks if the value on the left is greater than the value on the right.
Example: x > y returns True if x is greater than y, otherwise, it returns False.
1x = 8
2y = 5
3result = x > y # This evaluates to True because 8 is greater than 5
4
Less Than (<): Check if the value on the left is less than the value on the right.
Example: x < y returns True if x is less than y, otherwise, it returns False.
1x = 3
2y = 7
3result = x < y # This evaluates to True because 3 is less than 7
4
Greater Than or Equal To (>=): Check if the value on the left is greater than or equal to the value on the right.
Example: x >= y returns True if x is greater than or equal to y, otherwise, it returns False.
1x = 5
2y = 5
3result = x >= y # This evaluates to True because 5 is equal to 5
4
5
Less Than or Equal To (<=): Check if the value on the left is less than or equal to the value on the right.
Example: x <= y returns True if x is less than or equal to y, otherwise, it returns False.
1x = 4
2y = 6
3result = x <= y # This evaluates to True because 4 is less than 6
4
Equal To (==): Checks if the values on both sides are equal.
Example: x == y returns True if x is equal to y, otherwise, it returns False.
1x = 5
2y = 5
3result = x == y # This evaluates to True because 5 is equal to 5
4
5
Not Equal To (!=): Checks if the values on both sides are not equal.
Example: x != y returns True if x is not equal to y, otherwise, it returns False.
1x = 3
2y = 7
3result = x != y # This evaluates to True because 3 is not equal to 7
4
These examples demonstrate how relational operators compare values and return either True or False based on the specified condition. You can use these comparisons to make decisions and control the flow of your Python programs.
Logical Operators in Python
Logical operators in Python are like decision-makers in your code. They help you make decisions based on conditions, and they work with either "True" or "False" values. Here's how they work in simple terms:
1. and
- The "and" operator checks if both conditions on its left and right are True.
- If both are True, the whole expression becomes True; otherwise, it's False.
- Example: If it's sunny and warm, then it's good weather.
1sunny = True
2warm = True
3
4if sunny and warm:
5 print("It's good weather for a picnic!")
6else:
7 print("Maybe another day.")
8
9
2. or
- The "or" operator checks if at least one of the conditions on its left or right is True.
- If either is True, the whole expression becomes True; if both are False, it's False.
- Example: You can have ice cream if it's hot or you're happy.
1hot = True
2happy = False
3
4if hot or happy:
5 print("Time for ice cream!")
6else:
7 print("No ice cream today.")
8
3. not
- The "not" operator flips the condition. If something is True, "not" makes it False, and vice versa.
- Example: If it's not raining, you can go out.
1raining = False
2
3if not raining:
4 print("Let's go for a walk!")
5else:
6 print("Stay indoors.")
7
Logical operators are handy for making choices and controlling the flow of your Python programs. They help your code make decisions, just like you do in real life.
Bitwise Operators in Python
- Bitwise operators in Python are used to perform operations at the binary level, which means they work with 0s and 1s (binary digits) of data.
- For beginners, think of them like switches that can turn bits (0 or 1) on or off within numbers.
- There are several bitwise operators, but the key idea is manipulating individual bits to achieve specific results in your code.
- Here's a simple example:
- Imagine you have two binary numbers: 1010 and 1100. Bitwise operators allow you to perform operations like AND (&), OR (|), XOR (^), NOT (~), and bit shifting (<< and >>) on each corresponding pair of bits.
- For instance, if you use the & operator on 1010 and 1100, you get 1000. This means it turned off (set to 0) the bits where both numbers had 1s.
- In everyday programming, bitwise operators are often used for tasks like data compression, cryptography, and low-level hardware control, making them essential for certain types of applications but less commonly used for typical beginner-level coding.
1# Bitwise AND (&)
2a = 10 # Binary: 1010
3b = 12 # Binary: 1100
4result_and = a & b # Binary result: 1000, Decimal result: 8
5print(result_and)
6
7
8# Bitwise OR (|)
9a = 10 # Binary: 1010
10b = 12 # Binary: 1100
11result_or = a | b # Binary result: 1110, Decimal result: 14
12print(result_or)
13
14
15# Bitwise XOR (^)
16a = 10 # Binary: 1010
17b = 12 # Binary: 1100
18result_xor = a ^ b # Binary result: 0110, Decimal result: 6
19print(result_xor)
20
21
22# Bitwise NOT (~)
23a = 10 # Binary: 1010
24result_not = ~a # Binary result: -1011 (in two's complement form)
25print(result_not)
26
27
28# Left Shift (<<)
29a = 5 # Binary: 0101
30shifted_left = a << 2 # Binary result: 10100, Decimal result: 20
31print(shifted_left)
32
33
34# Right Shift (>>)
35a = 20 # Binary: 10100
36shifted_right = a >> 2 # Binary result: 0010, Decimal result: 5
37print(shifted_right)
38
39
- In this code, we demonstrate the bitwise AND, OR, XOR, NOT, left shift, and right shift operators with binary and decimal results.
- These operators manipulate the individual bits of numbers, allowing you to perform various operations at the binary level.
Membership Operator in Python
- The membership operator in Python is like a detective tool that helps you find out if something is inside a group or a collection of things.
- It's all about checking whether a particular item is a member of a sequence, like a list or a string.
- Imagine you have a bag of fruits, and you want to know if there's an apple inside it.
- The membership operator is what you'd use. It answers the question, "Is this thing in here?" "Is this here?" It answers the question.
In Python, we use two membership operators:
in
- This operator checks if something is a sequence.
not in
- This operator checks if something is not inside a sequence.
1#Using in Operator:
2fruits = ["apple", "banana", "cherry"]
3if "banana" in fruits:
4 print("Yes, 'banana' is in the list of fruits.")- print("Yes, 'banana' is in the fruit list.")
5else:
6 print("No, 'banana' is not in the list of fruits.")
7
This code checks if "banana" is in the list of fruits and prints the result accordingly.
1#Using not in Operator:
2animals = ["lion", "tiger", "elephant"]
3if "giraffe" not in animals:
4 print("Yes, 'giraffe' is not in the list of animals.") print("Yes, 'giraffe' is not in the animal list.")
5else:
6 print("No, 'giraffe' is in the list of animals.") print("No, 'giraffe' is in the animal list.")
7
Here, we use the not-in operator to check if "giraffe" is not in the list of animals and print the result.
These simple examples illustrate how the membership operators can help you quickly check for the presence or absence of items in lists or sequences in Python.