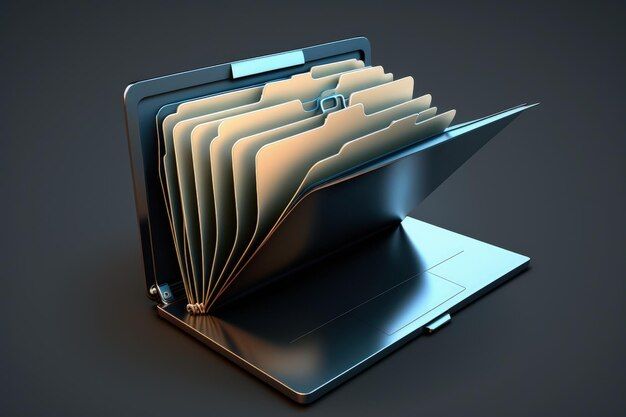
File Handling in Python
File Handling
- File handling in Python involves working with various types of files, including text files, binary files, and CSV files.
- You can perform a range of operations on files, such as creating, writing, appending, inserting, deleting, updating, and modifying data.
Types of Files
- Text File: Text files contain human-readable text and are typically encoded in plain text formats like ASCII or UTF-8.
- Binary Files: Binary files contain non-textual data and can be in various formats, such as images, audio, or any data that doesn't follow plain text encoding.
- CSV File: CSV (Comma-Separated Values) files store tabular data in plain text form, with values separated by commas.
Creating and Writing to a Text File in Python
- To create a new file, you can use the built-in open() function with the 'w' (write) mode for text files or 'wb' (write binary) mode for binary files.
- Here's an example of creating a text file and writing data:
1# Create and write to a text file
2with open('example.txt', 'w') as file:
3 file.write("Hello, this is a text file.\n")
4 file.write("You can write text to it.\n")
Appending Data to Files
- To append data to an existing file, you can use the 'a' (append) mode.
- Here's an example:
1# Append data to a text file
2with open('example.txt', 'a') as file:
3 file.write("This text is appended to the file.\n")
Insertion, Deletion, and Modification of Data
- Inserting, deleting, and modifying data in a file is more complex and typically involves reading the file, making the necessary changes in memory, and then writing the updated data back to the file.
- Here's an example of modifying a specific line in a text file:
1# Read the file into memory
2with open('example.txt', 'r') as file:
3 lines = file.readlines()
4
5# Modify a line
6lines[1] = "This line has been modified.\n"
7
8# Write the updated data back to the file
9with open('example.txt', 'w') as file:
10 file.writelines(lines)
For insertion and deletion, you would manipulate the lines list accordingly.
Conclusion
- File handling is an essential part of working with data in Python.
- You can create, write, append, insert, delete, update, and modify data in text and binary files.