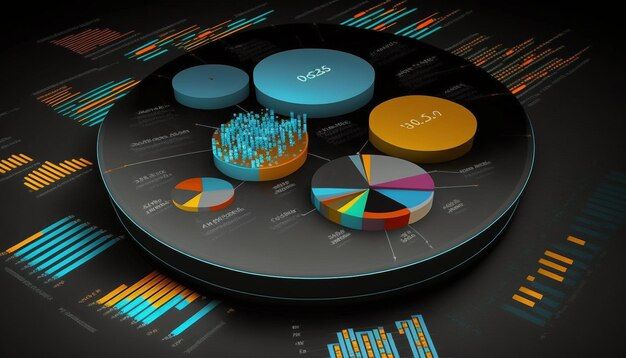
Introduction to Matplotlib (Bar graphs & Pie Charts)
What is Matplotlib?
- Matplotlib is a popular Python library for creating various types of data visualizations, including charts, plots, and graphs.
- It's widely used in data analysis and data visualization tasks.
- we'll explore how to create bar graphs and pie charts using Matplotlib.
Bar Graphs
- Bar graphs, also known as bar charts, are used to represent categorical data with rectangular bars.
- Each bar's length or height is proportional to the value it represents.
- Here's how to create a simple bar graph in Matplotlib:
1import matplotlib.pyplot as plt
2
3# Data for the bar graph
4categories = ['Category A', 'Category B', 'Category C', 'Category D']
5values = [10, 15, 7, 12]
6
7# Create a bar graph
8plt.bar(categories, values)
9
10# Add labels and a title
11plt.xlabel('Categories')
12plt.ylabel('Values')
13plt.title('Bar Graph Example')
14
15# Show the bar graph
16plt.show()
17plt.show()
- In this code, we use Matplotlib to create a bar graph with categories on the x-axis and corresponding values on the y-axis.
- You can customize the labels, titles, colors, and other aspects of the bar graph to suit your specific data.
Pie Charts
- Pie charts are used to represent data as a circular graph divided into slices, where each slice represents a category and its size is proportional to the data it represents.
- Here's an example of creating a pie chart in Matplotlib:
1import matplotlib.pyplot as plt
2
3# Data for the pie chart
4categories = ['Category A', 'Category B', 'Category C', 'Category D']
5sizes = [30, 15, 25, 30]
6
7# Create a pie chart
8plt.pie(sizes, labels=categories, autopct='%1.1f%%')
9
10# Add a title
11plt.title('Pie Chart Example')
12
13# Show the pie chart
14plt.show()
- In this code, we use Matplotlib to create a pie chart with categories, sizes, and percentage labels.
- You can customize the appearance, explode a slice for emphasis, and adjust other settings as needed.
Conclusion
- Matplotlib is a versatile library for creating various types of visualizations, and in this introduction, we've covered how to create bar graphs and pie charts.
- These visualizations are valuable for presenting and understanding data, making it easier to convey information and insights from your datasets.
- Matplotlib provides extensive customization options to tailor the appearance of your graphs and charts to your specific needs.