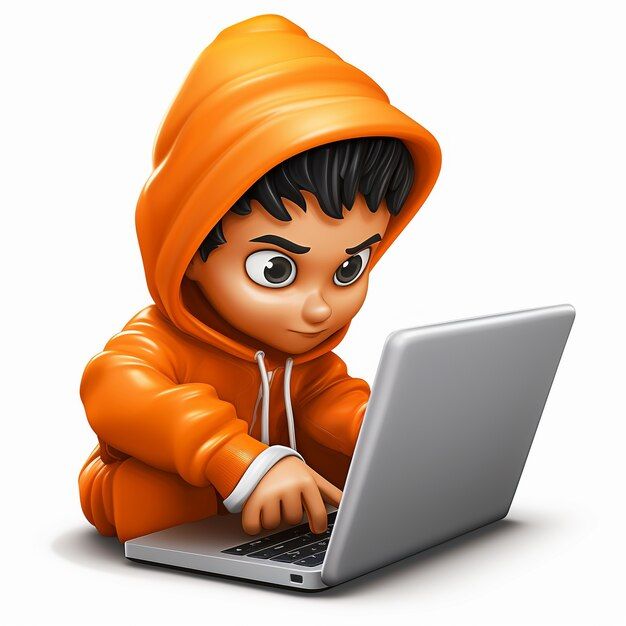
NumPy , Array Types and Array Operations in Python
what is NumPy?
- NumPy (Numerical Python) is a Python library.
- It provides support for multi-dimensional arrays, along with a collection of mathematical functions for arrays
Getting Started with NumPy
Before diving into working with NumPy, ensure that you have the NumPy library installed. If it's not already installed, you can do so using the pip package manager:
1pip install numpy
Importing NumPy
Next, you'll want to import the NumPy library into your Python script. You can achieve this by using a straightforward import statement:
1import numpy as np
This import statement creates an alias for NumPy, allowing you to use the shorthand "np" instead of the longer "numpy" in your code.
One-Dimensional Arrays
To create a one-dimensional array in NumPy, you can use the numpy.array() function. Here's an example:
1import numpy as np
2
3# Create a one-dimensional array
4arr = np.array([1, 2, 3, 4, 5])
5
6# Print the array
7print("One-Dimensional Array:")
8print(arr)
In this code, we import NumPy as np and create a one-dimensional array arr containing the elements 1 to 5. The print statement displays the array.
Re-shaping of an Array
- NumPy allows you to reshape arrays to change their dimensions.
- You can use the numpy.reshape() method or the .reshape() method of an existing array. Here's how:
1import numpy as np
2
3# Create an array
4arr = np.array([1, 2, 3, 4, 5, 6])
5
6# Reshape the array to a 2x3 matrix
7reshaped_arr = arr.reshape(2, 3)
8
9# Print the reshaped array
10print("Reshaped Array:")
11print(reshaped_arr)
In this example, we create an array with 6 elements and reshape it into a 2x3 matrix using the .reshape() method.
Creating 2-Dimensional Arrays
To create a 2-dimensional array, you can use a list of lists:
1import numpy as np
2
3my_2d_list = [[1, 2, 3], [4, 5, 6]]
4my_2d_array = np.array(my_2d_list)
5print(my_2d_array)
In this example, my_2d_array is a 2D array created from a list of lists. Each inner list represents a row in the array.
Elementwise Operations
- NumPy simplifies elementwise operations on arrays.
- You can apply mathematical operations to each element without explicit loops. Here's an example of adding two arrays elementwise:
1import numpy as np
2
3# Create two arrays
4arr1 = np.array([1, 2, 3])
5arr2 = np.array([4, 5, 6])
6
7# Perform elementwise addition
8result = arr1 + arr2
9
10# Print the result
11print("Elementwise Addition:")
12print(result)
In this code, we create two arrays and add them together element by element. The result will contain [5, 7, 9].
Aggregate Operations
- Aggregate operations in NumPy involve performing operations that combine multiple elements of an array to produce a single result.
- Common aggregate operations include calculating the sum, mean, maximum, or minimum of the elements in an array.
- Here's an example using NumPy:
1import numpy as np
2
3# Create an array
4arr = np.array([1, 2, 3, 4, 5])
5
6# Calculate the sum of all elements
7sum_result = np.sum(arr)
8
9# Calculate the mean of all elements
10mean_result = np.mean(arr)
11
12# Print the results
13print("Sum:", sum_result)
14print("Mean:", mean_result)
15
In this code, we use NumPy functions like np.sum() and np.mean() to perform aggregate operations on the array arr.
Array Indexing
- Array indexing in NumPy allows you to access individual elements within an array.
- NumPy arrays are zero-indexed, meaning the first element is accessed using index 0. Here's an example:
1import numpy as np
2
3# Create an array
4arr = np.array([1, 2, 3, 4, 5])
5
6# Access the second element (index 1)
7element = arr[1]
8
9# Print the element
10print("Element at index 1:", element)
11
In this code, we access the second element of the array arr using indexing.
Array Slicing
- Array slicing in NumPy allows you to extract a portion of an array, which is useful for working with subarrays.
- Here's an example:
1# Create an array
2arr = np.array([1, 2, 3, 4, 5])
3
4# Slice the array to get elements from index 1 to 3
5subarray = arr[1:4]
6
7# Print the subarray
8print("Sliced Subarray:", subarray)
9
In this code, we slice the array arr to extract elements from index 1 to 3, creating a subarray.
Insert Rows/Columns
You can insert rows or columns into a NumPy array using functions like numpy.insert(). Here's an example of inserting a row:
1import numpy as np
2# Create a 2D array
3arr = np.array([[1, 2, 3], [4, 5, 6]])
4
5# Row to insert
6new_row = np.array([7, 8, 9])
7
8# Insert the new row at index 1
9arr = np.insert(arr, 1, new_row, axis=0)
10
11# Print the updated array
12print("Updated Array with Inserted Row:")
13print(arr)
14
This code inserts a new row into the 2D array at index 1.
Append Rows/Columns
Appending rows or columns to a NumPy array can be done using functions like numpy.append(). Here's an example of appending a column:
1import numpy as np
2
3# Create a 2D array
4arr = np.array([[1, 2, 3], [4, 5, 6]])
5
6# Column to append
7new_column = np.array([7, 8])
8
9# Append the new column
10arr = np.append(arr, new_column[:, np.newaxis], axis=1)
11
12# Print the updated array
13print("Updated Array with Appended Column:")
14print(arr)
In this code, we append a new column to the 2D array.
Array Manipulation Operations
- Array manipulation operations in NumPy involve various functions for changing the shape, size, or structure of arrays.
- Functions like numpy.reshape(), numpy.transpose(), and numpy.vstack() can be used for these operations. Here's an example using reshape:
1import numpy as np
2
3# Create an array
4arr = np.array([1, 2, 3, 4, 5, 6])
5
6# Reshape the array to a 2x3 matrix
7reshaped_arr = arr.reshape(2, 3)
8
9# Print the reshaped array
10print("Reshaped Array:")
11print(reshaped_arr)
In this code, we use reshape to change the shape of the array from a 1D array to a 2x3 matrix.
Conclusion
- NumPy is a powerful library for numerical computing in Python, providing tools for creating arrays, reshaping them, and performing elementwise operations efficiently.
- These fundamental capabilities make it an essential tool for scientific and data analysis tasks.
- Remember, these are just the basics, and NumPy offers a wide range of advanced functionalities for more complex operations in scientific computing.