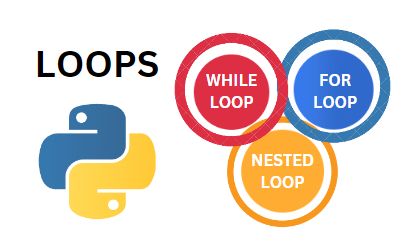
Loops in Python
Iteration (for Loop) in Python
Iteration is like doing something repeatedly, and in Python, we use a "for loop" to make this happen.
Imagine you have a list of chores, and you want to go through each one to complete them – that's what a for loop does in programming.
Here's how it works:
- List of Things: You start with a list of items you want to work with, like a list of numbers, names, or tasks.
- Go Through Each One: The for loop goes through each item in the list, one by one.
- Do Something: You can tell the loop to do something with each item in the list. It can be printing, calculating, or any task you need.
- Repeat Until Done: The loop keeps doing this for every item in the list until it reaches the end.
In this case, the for loop goes through each fruit in the list and prints it. So, you'll see "apple," "banana," and "cherry" printed one after the other.
1fruits = ["apple", "banana", "cherry"]
2
3for fruit in fruits:
4 print(fruit)
5
Iteration with for loops is a powerful way to work with data in your programs, especially when you need to perform the same task for multiple items.
While Loop in Python:
Iteration, in Python, is like a magic trick that lets your program repeat a set of instructions as long as a specific condition is true.
It's similar to doing something over and over again until you decide it's time to stop.
Think of it as eating your favorite snacks. You keep munching on chips until the bag is empty, right? That's a bit like how a while loop works.
Here's how it works in Python: Setting the Condition:
- First, you set a condition that your program will check.
- For example, you might want to eat chips while there are chips left in the bag.
Doing Something:
- Inside the loop, you specify what you want to do. This could be anything - printing a message, calculating numbers, or even eating chips.
Checking the Condition:
- After each time the loop does something, Python checks the condition.
- If the condition is still true, the loop continues. If it's false, the loop stops.
1chips_in_bag = 10
2
3while chips_in_bag > 0:
4 eat_chip()
5 chips_in_bag -= 1
6
7print("No more chips left!")
8
In this example, the while loop keeps running as long as there are chips in the bag (the condition chips_in_bag > 0 is true). It eats one chip in each iteration and decreases the count until there are no more chips left.
That's the magic of iteration with a while loop in Python – it lets your program repeat actions until a condition says it's time to stop.
Nested Loops in Python:
Imagine you have a set of boxes, and inside each box, there are more boxes. That's what nested loops are like in Python – loops inside loops!
Here's explanation:
1. What Are Nested Loops?
- Nested loops are loops placed inside other loops. This means you have a loop (like a 'while' or 'for' loop) inside another loop.
- • Nested loops are loops placed inside other loops. This means that it is a loop inside another loop (like a "while" or "for" loop).
- It's like having a treasure chest (outer loop) with smaller boxes (inner loop) inside it.
2. Why Use Nested Loops?
- Nested loops are used when you need to perform a task repeatedly for each item in a collection (like a list) and then repeat that process for multiple collections.
- Think of it as looking inside each box in the treasure chest and then checking each item inside those boxes.
3. Example: Printing a Grid
- One common use is to create grids or tables. You can use an outer loop to move through rows and an inner loop to move through columns.
1
2for row in range(3): # Outer loop for rows
3 for col in range(3): # Inner loop for columns
4 print("Row", row, "Column", col)
5
4. Keeping Things Organized
- Nested loops help you organize and work with complex data structures like 2D arrays or matrices.
- You can process elements row by row and then move on to the next row.
Example
1#Nested Loops with for Loop:
2
3for i in range(3): # Outer loop for rows
4 for j in range(3): # Inner loop for columns
5 print(f"Row {i}, Column {j}")
6
In this for loop example, we have an outer loop that runs three times, representing rows, and an inner loop that also runs three times for columns. It prints the row and column numbers in a 3x3 grid.
1#Nested Loops with while Loop:
2i = 0 # Initialize the row counter
3while i < 3: # Outer loop for rows
4 j = 0 # Initialize the column counter
5 while j < 3: # Inner loop for columns
6 print(f"Row {i}, Column {j}")
7 j += 1 # Increment the column counter
8 i += 1 # Increment the row counter
9
In this while loop example, we achieve the same result as the for loop.
We start with an outer loop that runs three times, and for each outer loop iteration, we have an inner loop that runs three times.
It also prints the row and column numbers in a 3x3 grid.
We use counters (i and j) to keep track of the row and column we're currently processing.
Both of these examples demonstrate how to use nested loops to work with rows and columns, which is a common use case for nested loops when dealing with grids, tables, or multi-dimensional data.
Break and Continue Statements in Python
Break and continue statements in Python are like special instructions you can give to your program while it's running.
They help control the flow of loops, like telling your computer to take a break or skip a step when certain conditions are met.
1. Break Statement
- The break statement is like an emergency stop button for loops.
- When used inside a loop, it immediately stops the loop from running, even if the loop's condition hasn't been met.
- Think of it as saying, "Stop everything and get out of here!"
- • Follow this with “Take everything and get out of here!” Think of it as.
Example:
1for number in range(1, 11):
2if number == 5:
3break# Stops the loop when number becomes 5
4print(number)
5
6
2. Continue Statement
- The continue statement is like a skip button for loops.
- When used inside a loop, it skips the current iteration and moves to the next one.
- It's like saying, "I'm not interested in this one; let's move on."
Example:
1for number in range(1, 11):
2if number == 5:
3continue# Skips number 5 and continues with the loop
4print(number)
These statements give you more control over your loops, allowing you to handle specific cases or avoid certain iterations. Think of them as helpful tools to make your code more flexible and efficient.