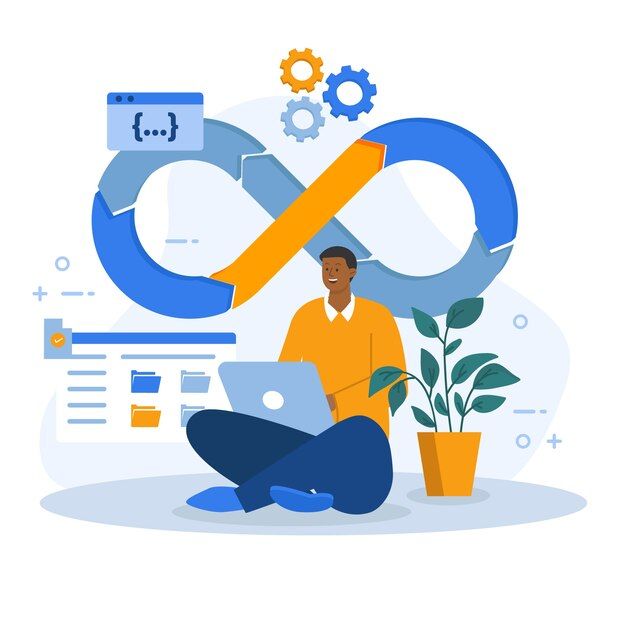
Control Structures in Python
Control structures are like the navigation tools in a Python program.
They help you decide what to do next based on certain conditions or criteria.
Imagine you're driving a car, and you have to choose whether to turn left, right, or go straight based on traffic signals – that's similar to what control structures do in programming.
Python if Condition Statements
- In Python, an if statement is like a decision-maker in your code.
- It helps your program make choices based on certain conditions.
- Imagine you're deciding whether to go outside: if it's sunny, you go out; if it's rainy, you stay inside.
- That's what if statements do in programming.
Here's how
- Condition Check: You start with a condition, which is like a question your program asks. Is it sunny?
- Decision: Then, based on the answer (True or False), your program decides what to do next. If it's sunny (True), go out; otherwise (False), stay inside.
In Python, if statements look like this:
if condition:
do_something()
- condition is the question your program asks.
- If the condition is True, the code inside the if block (indicated by the indentation) is executed.
- If the condition is False, the code inside the if block is skipped. • If the condition is False, the transfer of the code is prevented.
1temperature = 25 # Suppose the temperature is 25 degrees Celsius.
2
3if temperature > 30:
4 print("It's a hot day!") # This line will only run if the temperature is greater than 30.
5print("It's a hot day!") # This line only runs if the temperature is above 30 degrees.
6print("Enjoy your day!") # This line will always run.
7
In this code:
- We set the temperature variable to 25.
- The if statement checks if the temperature is greater than 30. If it is, it prints "It's a hot day!" because the condition is true.
- • Report if fever is above 30 degrees. If so, "It's a hot day!" in summer.
- The print("Enjoy your day!") statement will always run, regardless of the temperature, because it's not part of the if block.
So, if the temperature is greater than 30, you'll see "It's a hot day!" printed along with "Enjoy your day!" Otherwise, you'll only see "Enjoy your day!" because the if condition is not met.
If-Else Condition in Python:
- Imagine you have to decide whether to take an umbrella when you go outside.
- You look at the sky, and if it's cloudy, you take the umbrella; if it's not, you leave it behind.
- This decision-making process is a lot like what the "if-else" condition does in Python.
What is it?
- The "if-else" condition is like a traffic signal for your program. It helps your program make choices based on certain conditions.
- If a specific condition is true, your program does one thing (like taking an umbrella), and if it's false, it does something else (like not taking an umbrella).
How does it work?
- You start with an "if" statement, which checks if a condition is true.
- If the condition is true, the code inside the "if" block runs.• If the condition is true, run the code in the "if" block.
- If the condition is false, the code inside the "else" block runs.
1weather = "rainy"
2
3if weather == "rainy":
4 take_umbrella()
5else:
6 enjoy_sunny_day()
7
8
- In this example, the program checks if the weather is "rainy." If it is, it takes an umbrella; otherwise, it enjoys a sunny day.
- It's like giving instructions to your program based on what's happening, just like you decide whether to take an umbrella based on the weather.
The "if-elif-else" Condition in Python
- The "if-elif-else" condition in Python is like making choices in your program.
- It allows you to decide what to do based on multiple conditions.
- Think of it as a series of questions you ask, one after the other, to determine the right action.
Here's how it works:
if (If Statement):
- You start with an "if" statement to check a condition. If it's true, the code inside the "if" block runs.
Example:
if time < 12:
say("Good morning!")
elif (Else If Statement):
- If the "if" condition is false, you can use "elif" to check another condition. It's like asking a different question.
- You can have multiple "elif" blocks to check various conditions.
Example:
elif time < 18:
say("Good afternoon!")
else (Else Statement):
- If none of the previous conditions are true, the "else" block runs. It's like having a default action.
Example:
else:
say("Good evening!")
- With "if-elif-else," you can create decision trees in your program, making it flexible and responsive to different scenarios.
- It's like having a conversation with your program, where it chooses what to say based on the answers you provide.
1# Get the current time in 24-hour format (e.g., 13:45 for 1:45 PM)
2current_time = int(input("Enter the current time (in 24-hour format): "))
3current_time = int(input("Please enter the current time (24-hour format):"))
4
5# Check the time and greet accordingly
6if current_time < 12:
7 print("Good morning!")
8elif current_time < 18:
9 print("Good afternoon!")
10else:
11 print("Good evening!")
12
13
This code does the following:
- It asks the user to input the current time in 24-hour format.
- It uses the "if-elif-else" condition to check the time and print a greeting message based on the time of day.
- For example, if you input 9 as the current time, it will print "Good morning!"
- If you input 15, it will print "Good afternoon!" And if you input 20, it will print "If you enter 20, "Good night!" writer.
- Good evening!" This code demonstrates how you can use conditions to make decisions.