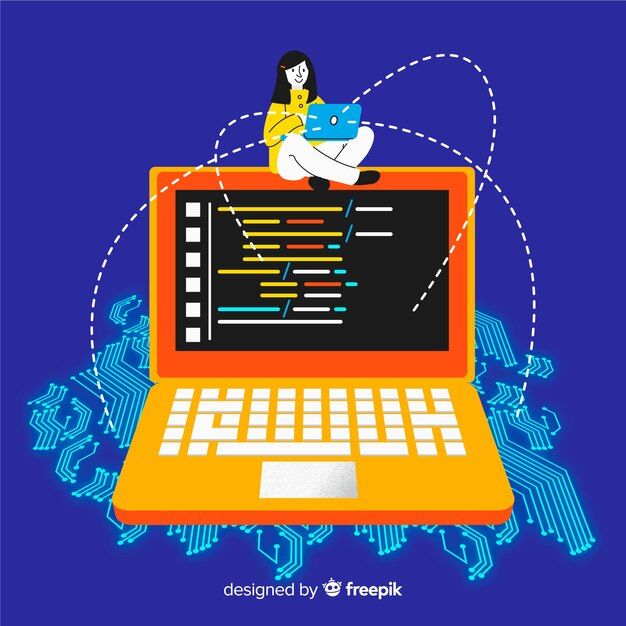
Modules and Packages in Python
Modules in Python
- Modules in Python are files containing Python code.
- They allow you to organize your code into reusable, separate units, making it easier to manage and maintain your programs.
Importing Modules
- You can import existing modules into your Python code using the import statement.
- For example, to use the math module, which provides mathematical functions and constants:
1import math
Once imported, you can access the functions and variables defined in the module using dot notation, such as math.sqrt(25).
import math Module
- The math module is a built-in Python module that provides various mathematical functions and constants.
- Some common functions include sqrt() for square root, sin() for sine, and pi for the value of π.
1import math
2
3print(math.sqrt(25)) # Computes the square root of 25
4print(math.sin(math.pi / 2)) # Calculates the sine of π/2 (which is 1.0)
5
Random Module
- The random module is another built-in Python module that allows you to generate random numbers and make random selections.
- It's often used for tasks like simulations, games, and statistical sampling.
1import random
2
3random_number = random.randint(1, 100) # Generates a random integer between 1 and 100
4random_choice = random.choice(["apple", "banana", "cherry"]) # Picks a random item from the list
5
Creating Your Own Modules
- Creating your own modules involves defining functions, variables, and classes in separate Python files.
- These files can then be imported and used in other Python scripts.
- Here's an example of creating a simple module named my_module.py:
1# my_module.py
2def greet(name):
3 return f"Hello, {name}!"
4
You can use this module in another script:
1import my_module
What are Packages?
- Packages are a way to organize related modules into a directory hierarchy.
- They help prevent naming conflicts and provide a structured approach to organizing your code.
- A package is essentially a directory containing a special file called __init__.py and one or more Python modules.
- For example, you can have a package structure like this:
1my_package/
2 __init__.py
3 module1.py
4 module2.py
5
To use a module within a package, you can import it like this:
1from my_package import module1
Packages are beneficial for large projects, as they help you organize and group related modules effectively.
Conclusion
- Modules and packages are fundamental to structuring Python code effectively.
- They allow you to reuse code and organize functionality.
- Understanding how to import modules, utilize built-in modules like math and random, and create your own modules and packages is essential for proficient Python development.