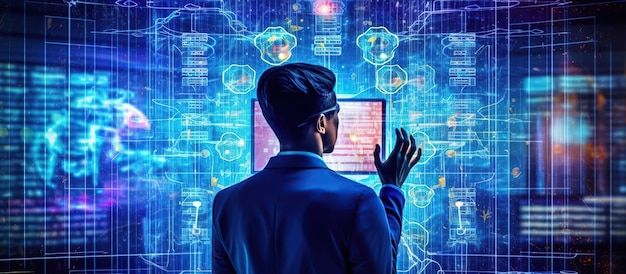
Introduction To Python
Introduction to Python
Variables
What Are Variables?
In Python, a variable is like a box or a container. You can put things (data) inside it. Just like you have a name, variables have names too. These names help you remember what's inside the box.
In Python, a variable is like a box or a container. You can put things (data) inside it. Just like you have a name, variables have names too. These names help you remember what's inside the box.
For example:
Imagine you have a box called my_number, and you put the number 5 inside it. Now, whenever you say my_number, you'll get 5.
Assignment Statements
An assignment statement is like a label you stick on a box to give it a name and put something inside it. In Python, you use the equal sign = for this.
For example:
1my_number = 5
2# Here, we put the number 5 inside the box called my_number.
So, when you later use my_number, Python knows you mean 5.
In short, variables and assignment statements are like giving names to boxes and putting things in them. This helps you work with data in your Python programs.
Operators
Operators in Python are special symbols or keywords used to perform operations on data or variables.
They are like tools that allow you to work with different types of values in your programs.Here are a few basic Python operator types:
Assignment Operator
In Python, the equal sign = is the fundamental assignment operator used to assign values to variables.
It's like giving a name to a box and placing something inside that box. Here's more about the assigment operator:
The equal sign = assigns a value to a variable.
For example,
1x = 10 #assigns the value 10 to the variable x.
Single Value Assignment
You can only assign one value to a variable at a time using the equal sign.
Trying to assign multiple values simultaneously will result in an error.
Variable Update
You can use the equal sign to update the value of a variable by assigning it a new value.
For instance, x = 5 sets x to 5, but x = 8 updates it to 8.
Right-to-Left Assignment
The variable on the left is given the value on the right by the equal sign.
So, in total = price, the value of price is assigned to total.
Clearing a Variable
You can also use the equal sign to clear a variable by assigning it a value like None.
For example,
name = None sets name to be empty or undefined.
The equal sign operator is the foundation of variable assignment in Python. It allows you to store and manipulate data by giving names to values, making it easier to work with information in your programs.
Unary operators
unary operators are special symbols used to perform operations on a single value or variable. They're like one-man bands - they don't need a partner; they can create a tune all by themselves. Here's what you need to know about unary operators:
What Are Unary Operators?
Unary operators work on just one value at a time.
They're like a magic wand that transforms a number or a variable on its own.
What Are Unary Operators?
Unary operators work on just one value at a time.
They're like a magic wand that transforms a number or a variable on its own.
Example:
1a = 10
2b = +a # b is still 10
Unary operators are handy when you want to flip the sign of a number or work with a value on its own. Just remember, they're the soloists of Python operators!
Binary Operators
In Python, binary operators are like math symbols that work with two values to produce a result. Think of them as tools you use when you need to combine or compare two things.
Binary operators are handy when you want to perform calculations, make comparisons, or manipulate data in your Python programs. They are an essential part of the language and allow you to do all sorts of interesting things with your data.
Binary operators are handy when you want to perform calculations, make comparisons, or manipulate data in your Python programs. They are an essential part of the language and allow you to do all sorts of interesting things with your data.
For Example:
1x = 5
2y = 3
3result = x + y
4print(result)
5
In this code:
We have two variables, x and y, which hold the values 5 and 3, respectively.
We use the + operator to add x and y together, and the result is stored in the result variable.
Finally, we print the result variable, which will display the sum of x and y, which is 8, on the screen.
So, the + operator, in this case, performs addition, allowing you to combine the values of x and y to get the result you want in your Python program.
Arthmetic Operators
Arithmetic operators in Python are like mathematical tools that help you perform basic calculations with numbers. They allow you to add, subtract, multiply, divide, and find remainders between numbers, making it easy to solve everyday math problems in your programs
Addition (+)
1product = 4 * 2 # Multiplies 4 and 2 to get the product 8
Subtraction (-): You can use it to find the difference between two numbers.
1difference = 8 - 3 # Subtracts 3 from 8 to get the difference 5
Multiplication (*): It's like adding a number repeatedly. If you have 4 * 2, you get 8.
1product = 4 * 2 # Multiplies 4 and 2 to get the product 8
Division (/): It's like splitting something into equal parts.
1quotient = 10 / 2 # Divides 10 by 2 to get the quotient 5.0 (float)
Modulus (%): This operator finds the remainder of a division.
1remainder = 7 % 3 # Finds the remainder when 7 is divided by 3, which is 1
Relational Operator :
Relational operators in Python are used to compare two values or expressions and determine their relationship or relative order. These operators return a Boolean value (True or False) based on the comparison result. Relational operators help you make decisions and control the flow of your program by evaluating conditions.The most typical relational operators are listed below:
Greater Than (>) : determines whether the value on the left exceeds the value on the right.
Less Than (=) : Checks if the value on the left is greater than or equal to the value on the right.