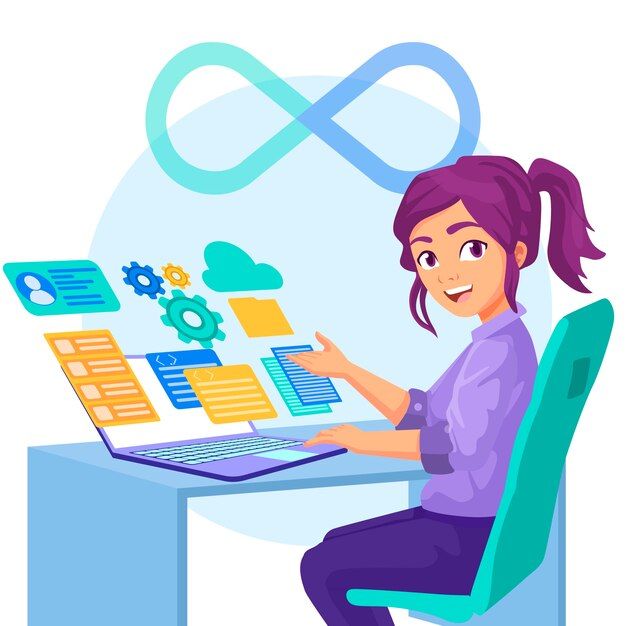
Functions in Python
- Functions in Python are blocks of reusable code that can perform a specific task or return a value.
- They help in organizing code and making it more modular.
Defining Functions
To define a function in Python, you use the def keyword, followed by the function name and parentheses, which can contain input parameters (also known as arguments).
The function body is indented. Here's an example:
1def greet(name):
2 return f"Hello, {name}!"
3
Calling Functions
Once a function is defined, you can call it by using its name followed by parentheses and, if needed, passing arguments. For example:
1message = greet("Alice")
Types of Functions in Python
- Built-in Functions
- User-Defined Functions
Built-in Functions
Python provides many built-in functions, like print(), len(), and input(), that you can use without defining them.
User-Defined Functions
These are functions you define yourself, like the greet( ) function we defined earlier.
Arguments
- Arguments are the values you pass to a function.
- They can be required or optional.
Return Values
Functions can return values using the return statement.
For example, the greet() function returns a greeting message.
Formal vs. Actual Arguments
Formal Arguments
Formal arguments are the parameters defined in a function's header, like name in greet(name).
Actual Arguments
Actual arguments are the values you provide when calling the function, like "Alice" in greet("Alice").
Scope
- Scope defines the visibility and accessibility of variables.
- Variables defined inside a function have local scope, while those defined outside have global scope.
Lifetime
- Lifetime refers to the duration a variable exists in memory.
- Local variables have a shorter lifetime compared to global variables.
Keyword Arguments
- You can pass arguments to a function using keyword arguments, specifying the parameter names explicitly.
- This can make your code more readable.
1greet(name="Bob")
Default Arguments
- You can set default values for function parameters, making them optional.
- If no value is provided, the default is used.
1def greet(name="Guest"):
2 return f"Hello, {name}!"
3
Recursion
- Recursion is a technique where a function calls itself.
- It's commonly used to solve problems that can be broken down into smaller, similar subproblems.
1def factorial(n):
2 if n == 0:
3 return 1
4 else:
5 return n * factorial(n-1)
6
- This function calculates the factorial of a number using recursion.
- Functions in Python are a fundamental concept that allows you to create organized, reusable code.
- Understanding how to define, call, and work with various aspects of functions is crucial for writing efficient and modular Python programs.
- If you have any questions or need further explanations, feel free to ask.
Conclusion
- In Python, functions are an integral part of writing clean, efficient, and organized code.
- They help structure your programs and enable code reuse.
- Functions in Python such as defining, calling, arguments, return values, scope, lifetime, and recursion, are crucial for proficient programming.