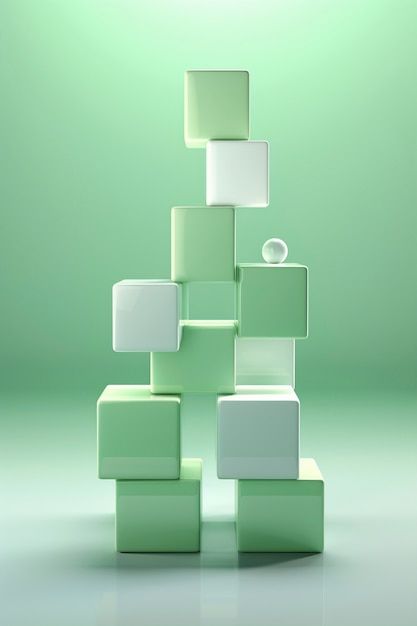
Stack Organization in Computer Organization
What is a Stack Organization?
Stack organization is a critical aspect of computer system architecture, and it plays a fundamental role in managing the execution of programs and handling function calls.
What is Stack?
- A stack is a fundamental data structure that follows the Last-In-First-Out (LIFO) principle.
- This means that the last item pushed onto the stack is the first to be popped off.
- Stacks are widely used in computer science and architecture, particularly for managing program execution and function calls.
- It is typically organized in the system's RAM (Random Access Memory).
- A stack typically consists of two primary operations: push, which adds an item to the top of the stack, and pop, which removes the top item.
Managing Function Calls
- When a program executes, it often involves the execution of multiple functions or procedures.
- Each time a function is called, information related to the function's execution, such as the return address and local variables, needs to be stored and managed.
- The stack is used to keep track of these function calls, making it possible to return to the calling function after the called function completes.
Example:
- Consider a simple program with two functions, functionA and functionB.
- When functionA calls functionB, the return address of functionA and local variables specific to functionA are pushed onto the stack.
- When functionB completes, it pops these values off the stack to return to the correct point in functionA.
Storing Local Variables
- Local variables are variables that are declared and used within a specific function.
- These variables are allocated on the stack, allowing each function to have its own separate memory space for local variables.
- This ensures that variables don't interfere with one another when multiple functions are in execution.
Example:
- In the context of a program, if functionA has a local variable x, and functionB also has a local variable x, they can coexist on the stack without conflicts.
- Each function accesses its own x variable stored in its respective stack frame.
Stack Frames
- A stack frame is a crucial concept in stack organization.
- It is a specific portion of the stack allocated for a particular function's execution.
- A stack frame contains information such as:
- Return address: This points to the instruction to be executed after the function call returns.
- Local variables: Space for local variables declared within the function.
- Parameters: If the function takes any parameters, they are stored in the stack frame.
- Control information: Additional information is required for function execution, like the previous stack frame's base pointer.
- Stack Pointer and Base Pointer: The stack pointer (SP) and base pointer (BP) are two essential registers used in stack organization.
Stack Pointer (SP)
- The SP points to the top of the stack. It is used to push and pop data onto and from the stack.
- When a value is pushed onto the stack, the SP is incremented, and when a value is popped, it is decremented.
Base Pointer (BP)
- The BP is used to reference the base of the current stack frame. It allows functions to access their parameters and local variables.
- When a function is called, the BP is typically set to the current SP value, creating a new frame.
Stack Operations
The stack primarily supports two fundamental operations:
1.) Push
- When a function is called, the information related to its execution is pushed onto the stack, creating a new stack frame.
- This includes the return address, parameters, and local variables.
2.) Pop
- When a function completes its execution, the stack frame is popped off, and the control returns to the calling function.
- This involves restoring the previous state, such as the return address and local variables.
Stack Overflow
- A stack overflow occurs when the stack's capacity is exceeded.
- This typically happens when there are too many function calls or excessive use of local variables, causing the stack to run out of space.
- This can lead to program crashes.
Stack Underflow
- A stack underflow occurs when an attempt is made to pop from an empty stack.
- This is generally an error condition and can also lead to program crashes.
Conclusion
- Stack organization is a fundamental concept in computer system architecture, playing a pivotal role in managing function calls and local variables.
- It ensures the orderly execution of programs and enables functions to coexist without interference.
- Understanding how the stack operates is crucial for programmers and computer architects, as it impacts the stability and efficiency of software systems.