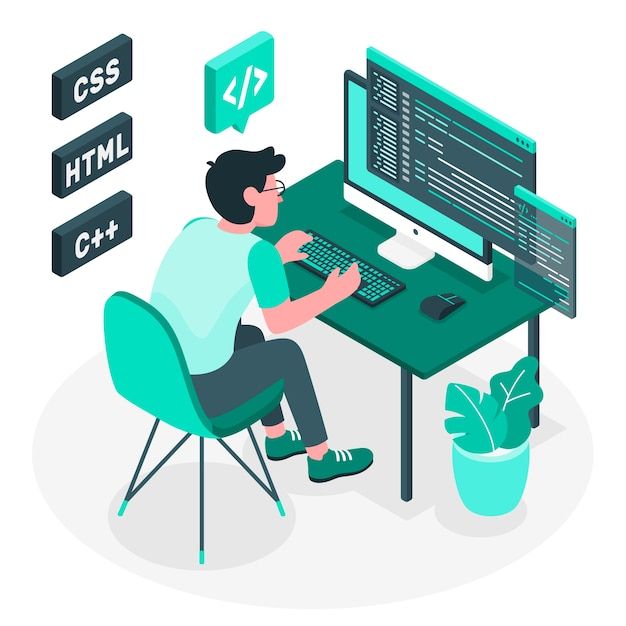
PHP Forms
- Working with forms and managing state is an integral part of web development.
- Forms allow users to input data, which can then be processed and stored using various methods.
HTML Forms: The Basics
Creating a Form
- To create a form in HTML, you use the <form> element.
- This element acts as a container for form controls such as text fields, buttons, and checkboxes. Here's a simple form example:
1<form action="process.php" method="post">
2 <!-- Form controls go here -->
3</form>
Form Controls
- Form controls are elements within the form that allow users to input data.
- Common form controls include <input>, <textarea>, <select>, and <button>.
- Each control has a specific purpose and can be customized with attributes.
The Get Method in PHP
- In PHP, the GET method is one of the HTTP request methods used to send data from a web page to a server.
- It is commonly used to retrieve or get back data from the server, such as submitting search queries.
- When a form is submitted using Get, the data is appended or attached, to the URL as query parameters.
1<form action="process.php" method="get">
2 <!-- Form controls -->
3 <input type="text" name="username" />
4 <input type="submit" value="Submit" />
5</form>
6
The Post Method in PHP
- The Post method is another HTTP method for submitting form data.
- Unlike Get, Post sends data in the request body, making it more suitable for sensitive or large data.
- It is commonly used for submitting form data, sending sensitive information such as
- login credentials or payment details, and transferring large amounts of data securely.
Here's an example:
1<form action="process.php" method="post">
2 <!-- Form controls -->
3 <input type="password" name="password" />
4 <input type="submit" value="Login" />
5</form>
6
When to use GET?
- Retrieving Data from the Server: GET is suitable for fetching data from the server, such as search results,
- where the data is not sensitive and only needs to be read.
- Sending Small Amounts of Non-Sensitive Data: GET is efficient for transmitting small amounts of data, such as parameters in a URL, where security is not a major concern.
- Caching Responses: GET requests can be cached by browsers and servers, improving performance for frequently accessed resources.
- Stateless Operations: GET requests are typically stateless, meaning each request is independent and does not rely on previous requests.
When to use POST?
- Sending Large Amounts of Data: POST is more suitable for transferring large amounts of data, such as file uploads or form submissions with multiple fields.
- Ensuring Data Privacy: POST requests provide better data privacy since the data is sent in the request body rather than in the URL.
- Avoiding URL Length Limitations: POST requests do not have URL length limitations like GET requests.
- Implementing Secure Operations: POST requests can be used for implementing secure operations like form submissions where data integrity and confidentiality are crucial.
State Management in Web Applications
- State management in web applications refers to the process of managing and maintaining the state or condition of a user's interactions and data within the application.
- State management is crucial in web applications to maintain user data and application state between requests.
Example:
- Shopping Cart in E-Commerce: When a user adds items to their shopping cart on an e-commerce website, the state of their cart needs to be managed.
- The state of the cart remains save or persist as the user navigates through different pages until they complete the checkout process or remove items from the cart.
We'll explore three essential techniques: Cookies, Session, and Query String.
Cookies in PHP
- Cookies are small portions or fractions of data stored on the client's machine.
- They can be used to remember user preferences or store session information.
- They can store information such as user preferences, session IDs, or shopping cart items.
- Cookies enable personalized experiences on websites by remembering user settings, language preferences, and previously viewed content.
- To set a cookie in PHP:
1setcookie("username", "john_doe", time() + 3600, "/");
Retrieving Cookies in PHP
You can retrieve cookies in PHP using the $_COOKIE superglobal:Example:
1$username = $_COOKIE["username"];
2
Session in PHP
- In web applications, a session refers to a period of interaction between a user and a website within a single
- Sessions in PHP allow you to store data on the server side that is associated with a specific user.
- for example:
- Example: Imagine a user logs into an e-commerce website to browse and shop for products.
- Once they log in, a session is initiated, and the website or Web app remembers their login state and other preferences during that browsing session.
- To start a session, you use the session_start() function.
- Once started, you can store and retrieve data in the $_SESSION superglobal.
Example:
1<?php
2// Start the session
3session_start();
4
5// Check if the user is already logged in
6if (isset($_SESSION['username'])) {
7 echo "Welcome back, " . $_SESSION['username'] . "!<br>";
8 echo '<a href="logout.php">Logout</a>';
9} else {
10 // Display the login form if the user is not logged in
11 echo '<form action="login.php" method="post">
12 <label for="username">Username:</label>
13 <input type="text" id="username" name="username">
14 <label for="password">Password:</label>
15 <input type="password" id="password" name="password">
16 <button type="submit">Login</button>
17 </form>';
18}
19?>
Storing and Retrieving Session Data
- You can store and retrieve data within a session by assigning values to keys in the $_SESSION array.
- This data will persist or saves across multiple requests as long as the session is active.
Example:
1$_SESSION["user_id"] = 123;
2$user_id = $_SESSION["user_id"];
3
Query String
- A query string is a way to pass data in URLs.
- It consists of key-value pairs separated by "&" symbols.
- For example, ?user=john&age=30 is a query string. You can append this to a URL to send data to the server.
Example:
1<a href="profile.php?user=john&age=30">View Profile</a>
2
Extracting Query Parameters in PHP
- In PHP, you can access the query parameters from the URL using the $_GET superglobal.
- This allows you to retrieve data sent via the query string and use it in your application.
Example:
1$user = $_GET["user"];
2
Adding Hidden Fields to a Form
- Hidden fields are special form controls that are not visible to users.
- They are often used to store data that needs to be sent with a form submission but should not be editable by the user.
Example:
1<input type="hidden" name="token" value="abc123" />
2
- In this example, a hidden field named "token" with the value "abc123" is included in the form.
- Users won't see it, but it will be sent with the form data when submitted.
Retrieving Hidden Field Values
- In PHP, you can access the values of hidden fields submitted with a form using the $_POST superglobal.
- This allows you to retrieve and use hidden field values in your server-side code.
Example:
1$token = $_POST["token"];
2
Simple Web Form in PHP
Let's create a simple login form that demonstrates the concepts we've covered so far.
HTML Form (index.php)
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>Simple Web Form</title>
7</head>
8<body>
9 <h1>Contact Us</h1>
10 <form action="submit_form.php" method="post">
11 <label for="name">Name:</label>
12 <input type="text" id="name" name="name" required><br><br>
13
14 <label for="email">Email:</label>
15 <input type="email" id="email" name="email" required><br><br>
16
17 <label for="message">Message:</label><br>
18 <textarea id="message" name="message" rows="4" cols="50" required></textarea><br><br>
19
20 <button type="submit">Submit</button>
21 </form>
22</body>
23</html>
24
- In this HTML form (index.php), we have input fields for name, email, and a textarea for the message.
- The form action is set to submit_form.php, and the method is set to post,
- indicating that the form data will be sent to the PHP script submit_form.php using the POST method.
Process Form Data (submit_form.php)
1<?php
2if ($_SERVER["REQUEST_METHOD"] == "POST") {
3 $name = htmlspecialchars($_POST['name']);
4 $email = htmlspecialchars($_POST['email']);
5 $message = htmlspecialchars($_POST['message']);
6
7 // Here, you can process the form data, such as sending an email, saving to a database, etc.
8
9 echo "<h2>Thank you, $name!</h2>";
10 echo "<p>Your message has been received. We will get back to you at $email shortly.</p>";
11} else {
12 // If the form is accessed directly without submitting, redirect to index.php
13 header('Location: index.php');
14 exit();
15}
16?>
- In the PHP script submit_form.php, we check if the form data was submitted using the POST method ($_SERVER["REQUEST_METHOD"] == "POST").
- We then retrieve the form data using $_POST['name'], $_POST['email'], and $_POST['message'].
- We use htmlspecialchars to sanitize the data and prevent XSS attacks.
Conclusion
We covered HTML forms, the Get and Post methods, and state management techniques like Cookies, Session, Query String, and Hidden Fields.