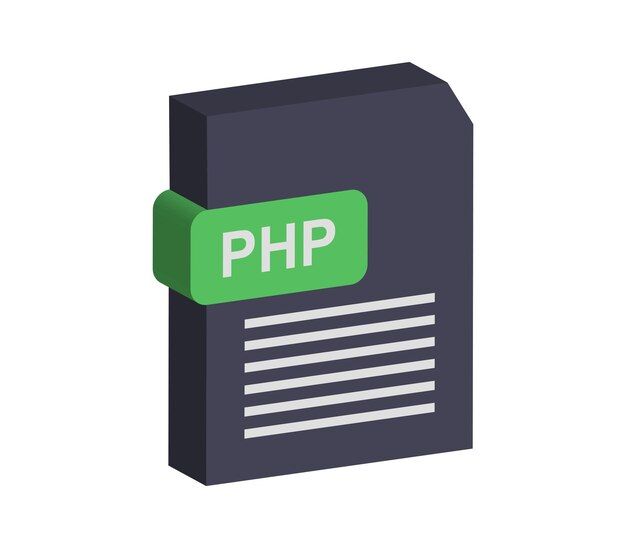
OOPS concepts in PHP with examples
Object-oriented programming (OOP)
Object-oriented programming is like building a program by breaking it down into smaller, reusable parts (objects) that have both data and abilities. It makes it easier to understand and manage complex code.
What are Classes and Objects?
In PHP, classes are like plans. Objects, on the other hand, are instances of classes. Think of a class as a template and an object as the product created from that template. for instance:
1// Define a class
2class Car {
3 // Properties (variables)
4 public $brand;
5 public $model;
6
7 // Methods (functions)
8 public function startEngine() {
9 echo "Engine started!";
10 }
11}
12
13// Creating object of class
14$myCar = new Car();
15$myCar->brand = "Toyota";
16$myCar->model = "Camry";
17$myCar->startEngine(); // Output: Engine started!
Understanding Access Modifiers
Public, Private, and Protected
Access modifiers define the visibility of class properties and methods. In PHP, there are three main access modifiers:
- Public: Properties and methods declared as public can be accessed from anywhere, both within and outside the class.
- Private: Private properties and methods can only be accessed from within the class itself.
- Protected: Protected properties and methods can be accessed within the class and its subclasses (inheritance).
1. Public
The public access modifier is the least restrictive and provides the broadest level of visibility. Properties and methods declared as public can be accessed from anywhere within your code, both within and outside the class in which they are defined. Here's how it works:
1class MyClass {
2 public $publicVar = "I'm public!";
3
4 public function publicMethod8() {
5 return "I am also public .";
6 }
7}
8
9$obj = new MyClass();
10
11// Accessing public property and method
12echo $obj->publicVar; // Output: I'm public!
13echo $obj->publicMethod8();
In this example, we have a class named MyClass with a public property (public) and a public method (public method8).
You can see that we can access both the property and the method from outside the class using an object of that class.
2. Private
The private access modifier is the most restrictive. Properties and methods declared as private can only be accessed from within the class where they are defined. They are not accessible from outside the class. Here's how it works:
1class MyClass {
2 private $privateVar = "I'm private!";
3
4 private function privateMethod() {
5 return "This is a private method.";
6 }
7
8 public function accessPrivate() {
9 return $this->privateVar . " " . $this->privateMethod();
10 }
11}
12
13$obj = new MyClass();
14
15// trying to access private property
16
17// Results in an error
18echo $obj->privateVar; // Error: Cannot access private property MyClass::$privateVar
19echo $obj->privateMethod(); // Error: Call to private method MyClass::privateMethod() from context ''
20
21// Accessing via a public method
22echo $obj->accessPrivate();
23// Output: I'm private!
24
In this example, we create a class named MyClass with private properties (privateVar) and a private method (privateMethod).
If you try to access them directly from outside the class, you'll encounter errors. However, we've also included a public method (accessPrivate) that can access and combine the private property and method, demonstrating that private members can be used within the class.
3. Protected
The protected access modifier provides intermediate visibility. Properties and methods declared as protected can be accessed from within the class where they are defined and within its subclasses (inheritance). They are not accessible from outside the class or its subclasses. Here's how it works:
1class ParentClass {
2 protected $message = "Chandryaan 3 is successful";
3
4 protected function showMessage() {
5 echo $this->message;
6 }
7}
8
9class ChildClass extends ParentClass {
10 public function displayMessage() {
11 $this->showMessage();
12 }
13}
14
15// Create an object of ChildClass
16$child = new ChildClass();
17
18// Access the protected method from ChildClass
19$child->displayMessage(); // Output: This is a protected message.
20
21
22echo $child->message; // Error: Cannot access protected property
23
In this example, we have a ParentClass with protected properties (protectedVar) and a protected method (protectedMethod).
Then, we create a ChildClass that extends ParentClass. The ChildClass can access the protected members of its parent class, but attempting to access them directly from outside the class hierarchy results in errors.
Achieving Reusability
Inheritance in PHP
One of the core principles of OOP is reusability. PHP allows you to create new classes by inheriting properties and methods from existing ones. This is achieved through the extends keyword. Consider this example:
1class Animal {
2 public $species;
3
4 public function speak() {
5 echo "Animal speaks!";
6 }
7}
8
9class Dog extends Animal {
10 public function speak() {
11 echo "Dog barks!";
12 }
13}
14
15$myDog = new Dog();
16$myDog->species = "Canine";
17$myDog->speak(); // Output: Dog barks!
In this code, the Dog class extends the Animal class, inheriting the species property and overriding the speak method to provide a specific behavior for dogs.
Constructors and Destructors in PHP
Initializing and Cleaning Up
In PHP, constructors and destructors are special methods that help with object initialization and cleanup. The constructor is called when an object is created, and the destructor is called when the object is destroyed.
1class Book {
2 public $title;
3
4 public function __construct($title) {
5 $this->title = $title;
6 echo "Book created: {$this->title}";
7 }
8
9 public function __destruct() {
10 echo "Book destroyed: {$this->title}";
11 }
12}
13
14$myBook = new Book("The Great Gatsby");
15unset($myBook); // Destroying the object
In this example, we create a Book class with a constructor (__construct) that initializes the title property when an object is created. We also have a destructor (__destruct) that displays a message when the object is destroyed.
Conclusion
In PHP, Object-Oriented Programming (OOP) is a coding approach that encourages the organization of code into reusable units called "objects." Each object has data (properties) and actions (methods).