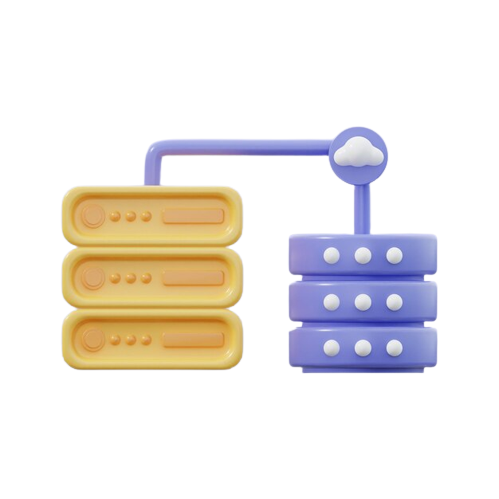
Arrays in PHP
What are Arrays in PHP?
- Arrays in PHP provide a convenient way to store multiple values under a single variable.
- Arrays allow us to access and manipulate elements based on their index position.
- Arrays can hold various types of data, including numbers, strings, objects, and even other arrays.
Types of Arrays in PHP
- Indexed Array
- Associate Array
- Multi-dimensional Array
PHP Indexed Array
- An indexed array, also known as a numeric array, is the simplest and most common type of array.
- In an indexed array, each element is assigned a numeric index starting from zero.
- Let's consider an example of an indexed array representing a list of fruits:
1<?php
2// Define the array of fruits
3$fruits = array("apple", "banana", "orange");
4
5// Print the array
6print_r($fruits);
7?>
8
- we create an indexed array named $fruits containing three elements.
- To access individual elements, we use their corresponding index. For instance, to retrieve the second element, we would use $fruits[1].
PHP Associate Array
- An associate array, also referred to as a key-value pair array, allows us to associate values with specific keys or names.
- Unlike indexed arrays, the elements in an associate array are not accessed by numeric indexes but rather by their assigned keys.
- To better understand this, let's look at an illustration:
1<?php
2// Define an associative array with student information
3$student = array(
4 "name" => "Sehaj Bindra",
5 "age" => 20,
6 "university" => "ABC University"
7);
8
9// Access and print specific elements of the array
10echo "Student Name: " . $student["name"] . "<br>";
11echo "Student Age: " . $student["age"] . "<br>";
12echo "University: " . $student["university"];
13?>
PHP Multi-dimensional Array
- A multi-dimensional array is an array that contains other arrays as its elements.
- It allows us to represent complex data structures such as matrices, tables, and grids.
- Let's consider an example of a two-dimensional array representing a tic-tac-toe board:
1<?php
2// Define a 2D array representing the tic-tac-toe board
3$board = array(
4 array("X", "O", "X"),
5 array("O", "X", "O"),
6 array("X", "O", "X")
7);
8
9// Loop through the rows and columns to print the board
10for ($i = 0; $i < 3; $i++) {
11 for ($j = 0; $j < 3; $j++) {
12 echo $board[$i][$j] . " ";
13 }
14 echo "<br>";
15}
16?>
When you run this PHP program, it will print the tic-tac-toe board as follows:
1X O X
2O X O
3X O X
4
This code initializes the $board array with the game state and then uses nested loops to iterate through the rows and columns, printing each symbol on the board.
Array Predefined Functions
- count ()
- array_push()
- array_merge()
- array_pop()
count()
- The count() function gives the array's total number of elements.
- When we need to determine the size of an array dynamically, it is especially helpful. Here's an illustration:
1$numbers = array (1, 2, 3, 4, 5);
2$count = count($numbers);
3echo "The array's size is given as: " . $count;
array_push()
- The array_push() function allows us to add one or more elements to the end of an array.
- It is commonly used to append new items to an existing array. Consider the following code snippet:
1$fruits = array("apple", "banana");
2array_push($fruits, "orange", "mango");
3print_r($fruits);
array_merge()
- A single array is created by combining two or more arrays using the array_merge() function.
- It is convenient when we are required to combine the contents of several arrays. Here's an illustration:
1<?php
2// Define the first array
3$first = array("apple", "banana");
4
5// Define the second array
6$second = array("orange", "mango");
7
8// Merge the two arrays
9$merged = array_merge($first, $second);
10
11// Print the merged array
12print_r($merged);
13?>
array_pop()
- The array_pop() function eliminates the final element of an array and returns it.
- It is frequently used for the last-in, first-out (LIFO) sequence of element extraction and processing. Here's an illustration:
1$fruits = array("apple", "banana", "orange");
2$lastFruit = array_pop($fruits);
3echo "The last fruit is: " . $lastFruit;
Output:
1The last fruit is: orange
These are just a few examples of the array of predefined functions available in most programming languages. By utilizing these functions effectively, you can perform a wide range of array operations efficiently.
Conclusion
Arrays are a fundamental tool in programming, allowing us to store and manipulate collections of data efficiently.
In this article, we explored three types of arrays: indexed array, associate array, and multi-dimensional array. We also discussed essential array predefined functions that aid in array manipulation.