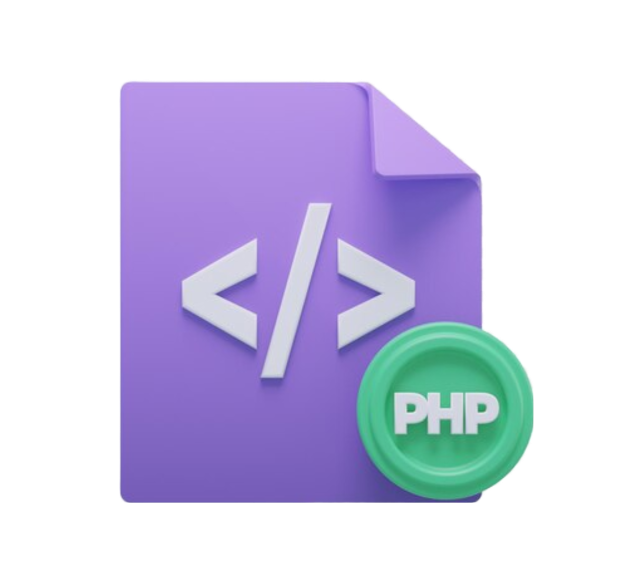
PHP full form | What is PHP ?
What is PHP Full Form?
PHP Full form is (Hypertext Preprocessor). PHP is a server-side scripting language, PHP is open-source software and PHP is compatible with various operating systems.
What is PHP used for?
- PHP is a commonly used programming language for building interactive web pages and web applications.
- PHP runs on the web server, which means it is responsible for handling server-side logic. For example user authentication.
- PHP is commonly used in the development of e-commerce platforms and online shopping carts.
- PHP can connect to various databases, including MySQL, PostgreSQL, and SQLite, to store and manipulate data.
What are PHP Data Types?
- Data types define the kind of data that can be stored and manipulated in a programming language.
- In PHP, there are several built-in data types, including:
- Integer: Represents whole numbers without decimal points.
- Float: Represents numbers with decimal points.
- String: Represents a sequence of characters.
- Boolean: Represents either true or false.
- Array: Represents an ordered collection of values.
- Object: Represents an instance of a class.
- Null: Represents a variable with no value assigned.
1// Integer
2$age = 25;
3
4// Float
5$price = 9.99;
6
7// String
8$name = "John Doe";
9
10// Boolean
11$isStudent = true;
12
13// Array
14$fruits = array("apple", "banana", "orange");
15
16// Object
17class Person {
18 public $name;
19 public $age;
20}
21
22$person = new Person();
23$person->name = "Jane Smith";
24$person->age = 30;
25
26// Null
27$noValue = null;
PHP Operators
Operators are used to perform various operations on data, such as arithmetic, assignment, comparison, logical, etc.
PHP supports a wide range of operators, including:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- String Operators
- Increment and Decrement Operators
1// Arithmetic Operators
2$a = 10;
3$b = 5;
4
5$sum = $a + $b;
6$difference = $a - $b;
7$product = $a * $b;
8$quotient = $a / $b;
9$remainder = $a % $b;
10
11// Assignment Operators
12$c = 20;
13$c += 5; // Equivalent to: $c = $c + 5;
14$c -= 3; // Equivalent to: $c = $c - 3;
15$c *= 2; // Equivalent to: $c = $c * 2;
16$c /= 4; // Equivalent to: $c = $c / 4;
17
18// Comparison Operators
19$x = 10;
20$y = 15;
21
22$isEqual = ($x == $y);
23$isNotEqual = ($x != $y);
24$isLessThan = ($x < $y);
25$isGreaterThan = ($x > $y);
26$isLessThanOrEqual = ($x <= $y);
27$isGreaterThanOrEqual = ($x >= $y);
28
29// Logical Operators
30$p = true;
31$q = false;
32
33$logicalAnd = ($p && $q);
34$logicalOr = ($p || $q);
35$logicalNot = !$p;
36
37// String Operators
38$name1 = "John";
39$name2 = "Doe";
40
41$fullName = $name1 . " " . $name2;
42
43// Increment/Decrement Operators
44$counter = 0;
45
46$counter++; // $counter = $counter + 1;
47$counter--; // $counter = $counter - 1;
- In this example, we have variables representing different operators in PHP.
- We perform arithmetic operations using arithmetic operators (+, -, *, /, %).
- assign values using assignment operators (=, +=, -=, *=, /=).
- compare values using comparison operators (==, !=, <, >, <=, >=).
- combine conditions using logical operators (&&, ||, !).
- concatenate strings using the string operator (.).
- increment or decrement values using increment/decrement operators (++ and --).
What are Variables in PHP?
- In PHP, variables are used to store and manage data.
- The variable name and dollar sign ($) are used to declare variables.
- It can store a variety of data types, including numbers, strings, arrays, objects, and more.
- Here is an illustration of declaring a variable and giving it a value:
1$name = "John Doe";
2$age = 25;
- In this example, the variable $name is assigned the value "John Doe" and the variable $age is assigned the value 25.
- Variables in PHP are case-sensitive, meaning $name and $Name would be treated as two different variables.
Super Global Variables in PHP
- Super global variables are predefined variables that are accessible from anywhere in a PHP script.
- They provide information about the server, user, and other parameters.
- Some commonly used super global variables in PHP include:
- The $_SERVER variable preserves details about the server and its execution environment.
- $_FILES: Contains information about uploaded files.
PHP Constants
- Constants are similar to variables, but their values cannot be changed once they are defined.
- They are useful for storing values that remain constant throughout the execution of a PHP script.
- Constants are defined using the define() function and are typically written in uppercase. Here's an example:
1define("PI", 3.14);
2define("SITE_NAME", "My Website");
- In this example, the constant PI is assigned the value 3.14, and the constant SITE_NAME is assigned the value "My Website".
- Constants provide a way to define and use fixed values that don't need to be modified during the execution of a script.
PHP Comments
- Comments are essential for documenting code and making it more understandable for developers.
- There are two types of comments in PHP as follows: Single-line comments start with //, while multi-line comments are enclosed within /* and */. Here's an example:
1// This is a single-line comment
2
3/*
4This is a multi-line comment.
5It can span multiple lines.
6*/
- Comments are not executed as part of the code and are ignored by the PHP interpreter.
- They serve as notes for the developers and help in improving code readability and maintainability.
PHP Regular Expressions
- Regular expressions provide a powerful way to match and manipulate text in PHP.
- They are patterns that can be used to search, extract, and replace specific parts of a string.
- PHP provides built-in functions, such as preg_match() and preg_replace(), for working with regular expressions. Here's an example:
1$string = "Hello, World!";
2if (preg_match("/Hello/", $string)) {
3 echo "Match found!";
4}
- In this example, the preg_match() function is used to check if the string contains the word "Hello".
- If a match is found, the message "Match found!" is displayed.
PHP Conditional Statements
- You can decide what to do and how to execute different code blocks using conditional statements.
- There are various categories of conditional statements in PHP:
if else Statement
The if-else statement allows you to execute a block of code if a certain condition is true, and a different block of code if the condition is false. Here's an example:
1$age = 25;
2if ($age >= 18) {
3 echo "You are eligible to vote!";
4} else {
5 echo "You are not eligible to vote yet.";
6}
- In this example, if the value of the variable $age is greater than or equal to 18, the message "You are eligible to vote!" will be displayed.
- Otherwise, the message "You are not eligible to vote yet." will be displayed.
if elseif else Statement
The if elseif else statement allows you to check multiple conditions and execute different blocks of code based on those conditions. Here's an example:
1$grade = 85;
2if ($grade >= 90) {
3 echo "Excellent!";
4} elseif ($grade >= 80) {
5 echo "Good!";
6} elseif ($grade >= 70) {
7 echo "Average!";
8} else {
9 echo "Needs Improvement.";
10}
In this example, the code checks the value of the variable $grade and displays a corresponding message based on the grade range.
Nested if Statement
An if statement inside another if statement is referred to as a nested if statement. Decision-making under more complex situations is attainable. Here's an instance:
1$age = 25;
2$isStudent = true;
3
4if ($age >= 18) {
5 if ($isStudent) {
6 echo "You will score good !";
7 } else {
8 echo "You qualify for a standard discount!";
9 }
10} else {
11 echo "you will not score well";
12}
- In this example, if the value of the variable $age is greater than or equal to 18, the code checks if the variable $isStudent is true or false.
- Depending on the result, it displays a different message.
Switch Case Statement
- You can carry out various operations depending on the various values of a variable or expression by using the switch case statement.
- It offers a clear way to write numerous if-else statements. Here's an instance:
1$day = "Monday";
2switch ($day) {
3 case "Monday":
4 echo "Today is Monday.";
5 break;
6 case "Tuesday":
7 echo "Today is Tuesday.";
8 break;
9 case "Wednesday":
10 echo "Today is Wednesday.";
11 break;
12 default:
13 echo "Today is not a weekday.";
14}
- In this illustration, the code executes the appropriate case after checking the value of the variable $day.
- The message "Today is Monday." will be shown if the value is Monday."
PHP Loops
for Loop
The for loop allows you to execute a block of code a specific number of times.
It consists of an initialization, condition, and increment/decrement section. Here's an example:
1for ($i =1; $i <= 5; $i++) {
2 echo "The value of i is: $i <br>";
3}
In this example, the loop will execute five times, displaying the value of $i each time.
PHP while Loop
The while loop allows you to execute a block of code as long as a certain condition is true. Here's an example:
1$i = 1;
2while ($i <= 5) {
3 echo "The value of i is: $i <br>";
4 $i++;
5}
In this example, the loop will execute as long as the value of $
i
is less than or equal to 5, displaying the value of $i each time.PHP Do while Loop
- Similar to the while loop, the do-while loop checks the condition at the end of the loop.
- As a result, the code block will always run at least once. Here's an illustration:
1$i = 1;
2do {
3 echo "The value of i is: $i <br>";
4 $i++;
5} while ($i <= 5);
In this example, the loop will execute once and then check the condition. If the condition is true, it will continue to execute.
PHP For each loop
When iterating through arrays or objects, the for-each loop is used. You can loop through each element without using an index thanks to this. Here's an illustration:
1$fruits = ["apple", "banana", "orange"];
2foreach ($fruits as $fruit) {
3 echo "I like $fruit <br>";
4}
- In this example, the loop will iterate over each element of the $fruits array and display the corresponding message.
- These loop structures provide flexible ways to repeat code execution based on specific conditions or iterations.
Conclusion
We have explored concepts of PHP, including PHP data types, PHP variables, PHP super global variables, PHP constants, PHP comments, PHP operators and regular expressions, the advantages of PHP, and control statements such as PHP conditional statements and PHP loops.