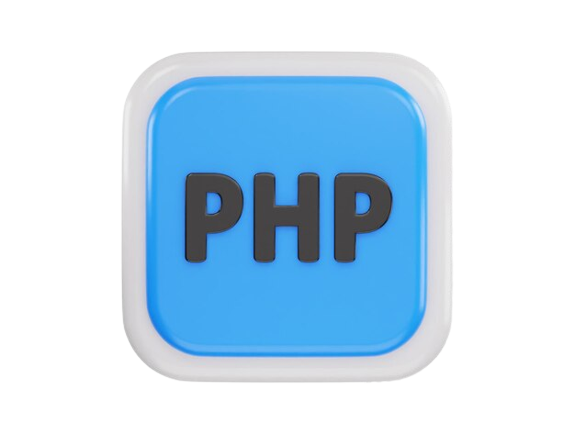
PHP Functions with Examples
What are PHP Functions?
- PHP functions are blocks of reusable code that perform specific tasks.
- Functions help organize code and make it easier to maintain and debug PHP Web applications.
Advantages of PHP Functions
- PHP Functions break down code into manageable parts, improving organization and readability.
- PHP has a vast standard library of built-in functions that cover a wide range of tasks, such as array operations, file handling, database interactions, and more.
- PHP Functions promote code reusability.
PHP Function Syntax
The syntax for the PHP function is as follows:
1function functionName($parameter1, $parameter2, ...) {
2 // Function code here
3 // It can use parameters and perform operations
4 return $result; // Optional: Return a value
5}
- function: This keyword is used to declare a function.
- functionName change this with the preferred name for your function.
- ($parameter1, $parameter2, ...): You can define input parameters (arguments) within the parentheses.
- You have the flexibility to include parameters in your function, and you can have none or several.
- If you have multiple parameters, make sure to separate them using commas.
- {}: The curly braces contain the function's code block.
- return $result; This optional line allows the function to return a value to the caller.
Defining Functions in PHP
Defining a function in PHP involves specifying its name, parameters (if any), and the code block that gets executed when the function is called.
1function greet() {
2 echo "Hello, world!";
3}
In the example above, we define a function called greet that has no parameters.
Calling Functions in PHP
Calling a function is as simple as writing its name followed by parentheses. Let's call or reinvoke the above-mentioned greet function we defined:
1greet();
When we execute the above code, it will output "Hello, world!" to the browser or command line, depending on where the PHP script runs.
Types of PHP Functions
There are two types of PHP functions, each serving a specific purpose:
- PHP Build-in Functions
- PHP User-Defined Functions
PHP Built-in Functions
- These are provided by PHP and cover a wide range of tasks, such as string manipulation, array handling, file operations, and database interactions.
- Examples include strlen(), array_push(), and mysqli_query().
PHP User-Defined Functions
- These are custom functions created by developers to perform specific tasks in their PHP web applications.
- Developers define the function name, parameters, and functionality. They are reusable within the application.
PHP Functions Arguments
- PHP function arguments, also known as parameters, are values that you can pass into a function when you call it.
- These arguments are used within the function to perform specific operations. Here are some key points about PHP function arguments:
Multiple Parameters: You can define multiple parameters by separating them with commas.
1function add($num1, $num2) {
2 $sum = $num1 + $num2;
3 echo "The sum is $sum";
4}
Order Matters: The order in which you pass arguments should match the order in which the parameters are defined in the function.
1add(5, 3); // Calling the add function with 5 and 3 as arguments.
PHP Call by Value
- In call by value, the function receives a copy of the original data when arguments are passed.
- Any changes made to the parameter within the function do not affect the original data outside the function.
- PHP uses call by value as the default parameter passing method.
1function increment($num) {
2 $num++;
3}
4
5$value = 5;
6increment($value);
7echo $value; // Output: 5 (original value remains unchanged)
PHP Call By Reference
- In call by reference, the function receives a reference or pointer to the original data when arguments are passed.
- Changes made to the parameter within the function directly affect the original data outside the function.
- To explicitly pass an argument by reference, you use the & symbol in the function parameter definition and the function call.
1function increment(&$num) {
2 $num++;
3}
4
5$value = 5;
6increment($value);
7echo $value; // Output: 6 (original value is modified)
PHP Variable Scope
- Variable scope refers to the accessibility and lifetime of variables within a program.
- In PHP, variables can have different scopes, such as global scope, function scope, and block scope.
- When a variable is defined inside a function, it's only accessible within that function unless explicitly declared as global or static.
- On the other hand, variables defined outside any function have a global scope and can be accessed from anywhere in the script. Let's see an example:
1$globalVariable = "I'm a global variable";
2
3function testScope() {
4$localVariable = "I'm a local variable";
5
6$x = 10; // Variable with block scope
7
8if ($x > 5) {
9
10$y = 20; // Variable with block scope
11
12echo $y; // Output: 20}
13
14echo $localVariable;
15echo $globalVariable; // Error: undefined variable
16}
17
18testScope();
19echo $globalVariable;
20echo $localVariable; // Error: undefined variable
- In the above example, the $globalVariable is accessible inside the testScope function without any issues.
- However, the $localVariable defined inside the function can't be accessed outside of it.
PHP Mail Function
- The mail function in PHP allows you to send emails from your web application.
- It takes several parameters, such as the recipient's email address, subject, message, and additional headers.
- Here's an example of sending an email using the mail function:
1$to = "recipient@example.com";
2$subject = "Hello from PHP";
3$message = "This is a test email.";
4$headers = "From: sender@example.com";
5
6if (mail($to, $subject, $message, $headers)) {
7 echo "Email sent successfully!";
8} else {
9 echo "Failed to send email.";
10}
- The above code sends an email to the specified recipient with the given subject, message, and headers.
- It checks if the email was sent successfully and provides appropriate feedback.
Handling PHP Errors
- PHP provides error-handling mechanisms to help developers identify and resolve issues in their code.
- Errors can occur due to syntax mistakes, undefined variables, or other runtime issues.
- By default, PHP displays error messages on the screen, which is not desirable in a production environment.
- To handle errors more effectively, we can configure PHP to log errors to a file or handle them programmatically using error-handling functions. Here's an example:
Enable Error Reporting
- During development, enable error reporting to see error messages.
- In your PHP script, add the following line at the beginning to display errors on the screen:
1error_reporting(E_ALL);
2ini_set('display_errors', 1);
Use Descriptive Error Messages
- When writing your code, include descriptive error messages when errors occur.
1if ($result === false) {
2 die("Error: Unable to perform the operation.");
3}
Conclusion
PHP functions are essential for organizing and enhancing code reusability in web applications. They can be defined with parameters and used to perform specific tasks.