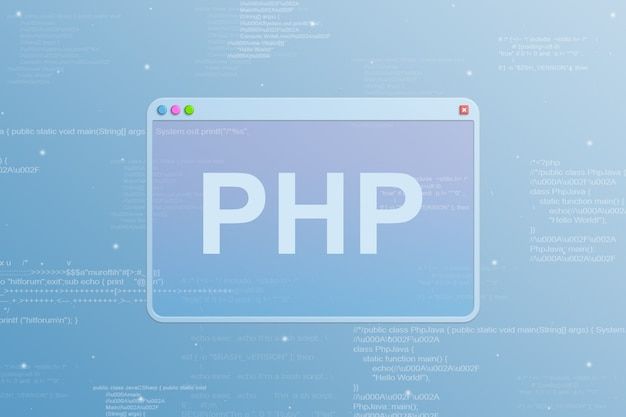
File Handling in PHP with Example
What is file in PHP?
- In PHP, a "file" typically refers to a digital document or a resource that is stored on a computer's file system.
- Files in PHP can come in various formats, such as text files, binary files, and more.
What is file handling in PHP?
File handling in PHP refers to working with files, such as creating files, reading files, writing files, and manipulating the files within the PHP.
PHP Open File - fopen()
- You can open a file with the fopen() function.
- It takes two arguments: the filename and the mode in which you want to open the file (read, write, append, etc.).
1$file = fopen("example.txt", "r");
- example.txt is the name of the file we want to open.
- "r" indicates that we are opening the file in read-only mode.
PHP Close File - fclose()
It's essential to close a file once you're done with it. Use the fclose() function to do this.
1fclose($file); // Closes the file
PHP Read File - fread()
- Reading a file is a common task, and PHP makes it straightforward with fread().
- This function allows you to read the content of a file.
Let's look at a basic example:
1$file = fopen("example.txt", "r");
2$data = fread($file, filesize("example.txt"));
3fclose($file);
- filesize("example.txt") calculates the size of the "example.txt" file in bytes, which is then used as the number of bytes to read.
So, this line of code reads the content of the "example.txt" file, which is stored in the variable $data. After this line executes, $data will contain the entire content of the "example.txt" file.
PHP Write File - fwrite()
- Writing to a file is useful when you want to store data or configuration changes.
- Here's how you can write content to a file using fwrite():
1$file = fopen("example.txt", "w"); // Open in write mode
2$content = "file handling in php";
3fwrite($file, $content);
4fclose($file);
PHP Delete File - unlink()
- Deleting a file is as simple as it gets.
- The unlink() function removes a file from the server or local directory.
1unlink("example.txt"); // Deletes example.txt
Creating a Directory in PHP
- To create a directory in PHP, you can use the mkdir() function.
- Accepts a list as an argument and creates the list in the current working directory.
1mkdir("new_directory");
let's see PHP programs for each of the PHP file-handling functions
PHP Open File
1<?php
2// Open a file in read-only mode
3$file = fopen("example.txt", "r");
4
5if ($file) {
6 echo "File opened successfully.";
7 fclose($file); // Close the file when you're done
8} else {
9 echo "Failed to open the file.";
10}
11?>
12
PHP Close File
1<?php
2$file = fopen("example.txt", "r"); // Open a file in read-only mode
3
4if ($file) {
5 // Do some operations with the file
6
7 // Close the file when you're done
8 fclose($file);
9 echo "File closed successfully.";
10} else {
11 echo "Failed to open the file.";
12}
13?>
PHP Read File
1<?php
2$file = fopen("example.txt", "r"); // Open in read-only mode
3
4if ($file) {
5 // Read the entire file
6 $content = fread($file, filesize("example.txt"));
7 fclose($file); // Close the file
8
9 // Display the file content
10 echo $content;
11} else {
12 echo "Failed to open the file.";
13}
14?>
PHP Delete file
1<?php
2$fileToDelete = "example.txt";
3
4if (unlink($fileToDelete)) {
5 echo "File '$fileToDelete' has been deleted.";
6} else {
7 echo "Failed to delete '$fileToDelete'.";
8}
9?>
10
PHP Write file
1<?php
2$file = fopen("example.txt", "w"); // Open in write mode
3
4if ($file) {
5 // Content to write to the file
6 $content = "This is some text to be written to the file.";
7
8 // Write content to the file
9 fwrite($file, $content);
10
11 // Close the file when you're done
12 fclose($file);
13 echo "Content has been written to the file.";
14} else {
15 echo "Failed to open the file.";
16}
17?>
18
What is File Inclusion in PHP?
- File Inclusion is a powerful feature in PHP that allows you to include the contents of one PHP file within another.
- Think of it as building blocks; you can create modular code and reuse common elements, saving time and effort.
Including Files in PHP
To include a file in PHP, you have two options: include and require statements. These commands pull in the content of another PHP file into your current one.
Difference between Include and Require
Include Statement:
- The include statement is used to include a file in your PHP script.
- If the included file is not found or there's an error, PHP will generate a warning but continue executing the script.
- In other words, if the included file is missing or has an issue, it won't stop your entire script from running. It's more lenient.
- It's commonly used for including files that are not crucial for the script's functionality, such as optional components or templates.
Require Statement:
- The require statement is also used to include a file in your PHP script.
- However, if the included file is not found or there's an error, PHP will generate a fatal error, and the script execution will stop immediately.
- In essence, if the required file is missing or problematic, it will halt the entire script. It's more strict and ensures that essential files are present and functioning.
- It's typically used for including files that are critical for the script's functionality, such as configuration files or important libraries.
In summary, the key difference lies in how they handle errors:
- include generating a warning for errors and continuing script execution.
- require generates a fatal error for errors and stops script execution.
Handling File Uploads
File uploads and downloads are common tasks in web applications. PHP simplifies these processes, making them beginner-friendly.
Uploading Files
- To allow users to upload files to your web application, create an HTML form with an input field of type "file."
- PHP can then handle the uploaded files using the $_FILES superglobal.
1<form action="upload.php" method="post" enctype="multipart/form-data">
2 <input type="file" name="fileToUpload">
3 <input type="submit" value="Upload File">
4</form>
Enabling File Downloads
To let users download files from your website, you must set appropriate headers and provide a way to read and serve the file's content.
1$file = "example.pdf";
2header("Content-Disposition: attachment; filename=" . basename($file));
3readfile($file);
Getting Information about Files
Understanding your files is crucial for effective file management in your application. PHP offers various functions to retrieve file information.
File Information Functions
- file_exists(): Checks if a file exists.
- is_file(): Determines if a path is a regular file.
- filemtime(): Retrieves the last modification time of a file.
1$filename = "example.txt";
2if (file_exists($filename)) {
3 echo "File $filename exists.";
4}
Conclusion
In conclusion, mastering file handling in PHP is a vital skill for web developers. It enables you to manage data efficiently, create dynamic web experiences, and build robust applications.