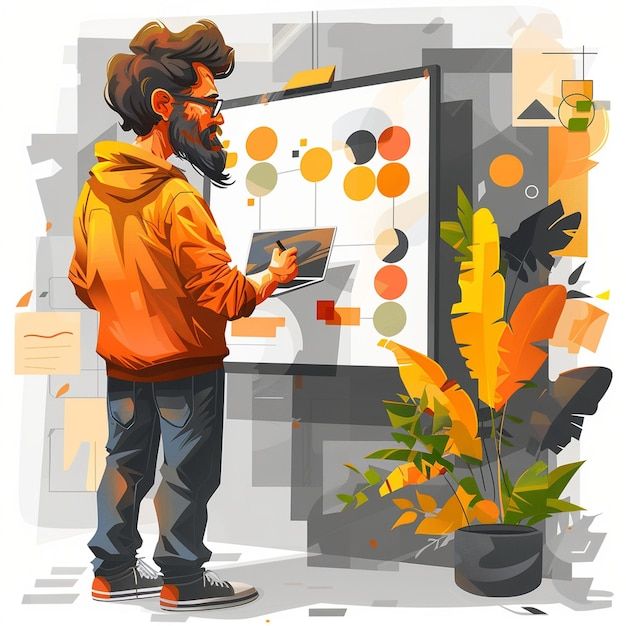
Painter's Algorithm in Computer Graphics
- The Painter's Algorithm is a fundamental technique in computer graphics used to render 3D scenes by determining the correct order for drawing objects.
- The method is named after the way a painter would approach a canvas: painting distant objects first and layering nearer objects on top to create depth.
- This technique ensures that objects closer to the viewer obscure those farther away, providing the illusion of three-dimensionality on a two-dimensional surface.
- Despite its simplicity, the Painter's Algorithm is an important concept in the history of computer graphics, though it has limitations in handling complex scenes.
How the Painter's Algorithm Works
- The Painter's Algorithm operates by sorting the polygons (or other 3D shapes) in a scene based on their distance from the viewer.
- Once sorted, polygons are drawn in order from the farthest to the nearest.
- This layering effect creates the appearance of depth, ensuring that closer objects obscure those behind them.
Step 1: Depth Sorting
- Depth Calculation: Each object’s depth is typically calculated by finding the distance from the viewer to a reference point on the object, often the centroid or center.
- Sorting Order: Objects are then sorted based on this depth value, with the farthest objects placed first in the drawing order.
Step 2: Rendering from Back to Front
- Drawing Order: Once sorted, the objects are rendered starting from the back of the scene and working towards the front.
- Layering Effect: This ensures that nearer objects are painted over objects that are farther away, creating the illusion of depth and perspective.
Key Considerations in Painter's Algorithm
While the Painter’s Algorithm is easy to implement, it has certain limitations, particularly when dealing with complex scenes or overlapping objects.
Overlapping and Intersecting Polygons
- Problem: When polygons intersect or overlap, sorting by depth alone can produce incorrect results because there may not be a single, clear back-to-front order.
- Example: Consider two walls meeting at a right angle. From certain angles, one wall may appear in front of the other, but from other perspectives, the order might reverse. This ambiguity can confuse the sorting process.
Cyclic Overlap (The Painter’s Algorithm Problem)
- Problem: Cyclic overlap arises when three or more objects overlap in a way that prevents a clear depth order from being established.
- Example: If Object A overlaps Object B, Object B overlaps Object C, and Object C overlaps Object A, there’s no straightforward way to sort the objects in a back-to-front order that works from all angles.
Performance and Complexity
- Efficiency: The sorting operation has a time complexity of O(n log n), where n is the number of polygons. Sorting can become time-consuming for scenes with many objects.
- Optimization: To improve performance, spatial data structures like BSP trees can be used to minimize the number of objects that need sorting.
Painter's Algorithm Example
Let's break down a simple example to see how the Painter’s Algorithm is applied.
Scene Setup
Imagine a 3D scene with three objects:
- Object A: A distant mountain.
- Object B: A house, located closer to the viewer.
- Object C: A tree, located even closer.
Sorting by Depth: The objects are sorted from farthest to nearest, producing the order: A, B, C.
Rendering Order: The objects are drawn in this sorted order:
- First, Object A (mountain) is drawn.
- Next, Object B (house) is drawn, partially covering Object A.
- Finally, Object C (tree) is drawn, obscuring parts of both Object A and Object B.
This process ensures that the frontmost objects obscure the ones behind them, creating a layered, depth-filled scene.
Advantages and Limitations of Painter's Algorithm
While the Painter’s Algorithm is simple and effective in many cases, it has both strengths and weaknesses.
Advantages of Painter's Algorithm
- Simplicity: The algorithm is easy to understand and implement. It primarily involves sorting and sequentially drawing polygons, making it an accessible technique for basic 3D rendering.
- Flexibility: It works well in scenes where objects don’t intersect or overlap in complex ways, making it useful in simpler 3D environments.
Limitations of Painter's Algorithm
- Handling Overlaps: The algorithm struggles with intersecting or cyclically overlapping objects. When objects partially overlap in an unusual way, the sorting based on depth alone may not produce the correct result.
- Performance for Complex Scenes: Sorting a large number of objects can be computationally expensive, especially in scenes with many polygons.
- Inefficiency with Transparency: The algorithm is not well-suited for handling transparent objects, as it does not correctly layer objects with varying transparency.
Alternative Approaches and Solutions
To overcome the limitations of the Painter’s Algorithm, other depth management techniques are often used.
Depth Buffer (Z-Buffer) Technique
- The Z-buffer algorithm stores the depth of every pixel in a buffer. When a new object is drawn, its pixel depth is compared with the stored value, and only the closest pixels are drawn.
- Advantage: The Z-buffer handles overlapping objects without requiring sorting, and it works at the pixel level, which is more accurate than sorting entire objects.
- Comparison: Unlike the Painter’s Algorithm, which relies on sorting the entire scene,
- the Z-buffer operates on individual pixels, making it more effective for complex scenes.
Conclusion
The Painter’s Algorithm is a straightforward and historically significant technique for rendering 3D scenes by sorting objects based on their depth and drawing them in a back-to-front order.
While simple, it has limitations, particularly in handling complex overlapping objects or large scenes.