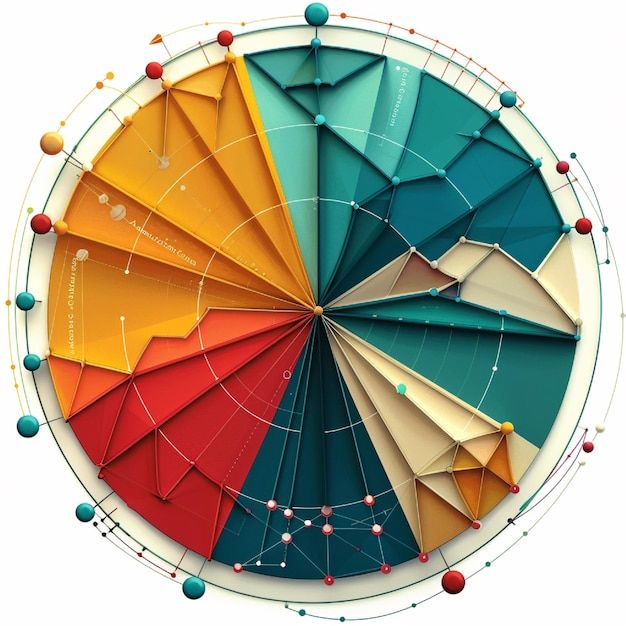
Bresenham’s Circle Drawing Algorithm in Computer Graphics
Bresenham's Circle Drawing Algorithm is a scan conversion technique based on incremental calculations, derived from Bresenham's Line Drawing Algorithm first introduced by Jack E. Bresenham in 1962.
This circle algorithm, like its predecessor, is optimized for integer-only arithmetic, making it ideal for computer graphics where floating-point operations are less efficient.
Principles of the Algorithm
- The algorithm relies on the symmetry of a circle, where each point ((x, y)) adheres to the equation:
- [ x^2 + y^2 = r^2 ]
- where ( r ) is the radius. By calculating points for one-eighth of the circle, the algorithm mirrors these across the other octants, enabling the complete circle to be drawn efficiently.
- It uses a decision parameter to select the pixel closest to the actual circle at each step, minimizing error.
- Starting from the top of the circle, the algorithm moves downward toward the x-axis, plotting points in each octant as it progresses.
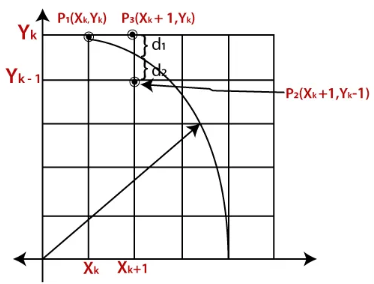
Step-by-Step Process
Initialization
- Assume the circle's center is at the origin ((0,0)) for simplicity. For a circle centered at ((h, k)), adjust the points accordingly.
- Define the radius ( r ).
- Begin with the initial point at ((0, r)).
- Initialize the decision parameter ( p = 1 - r ).
Plotting Points
For each point ((x, y)) starting from ((0, r)):
- Plot the point in all eight octants
- Update the coordinates based on the decision parameter:
- If ( p < 0 ), the next point is ((x + 1, y)), and ( p ) is updated to ( p = p + 2x + 1 ).
- If ( p \geq 0 ), the next point is ((x + 1, y - 1)), and ( p ) is updated to ( p = p + 2x - 2y + 1 ).
Utilizing Symmetry
Reflect the points plotted in the first octant across the other seven octants.
Advantages of Bresenham's Circle Drawing Algorithm
- Efficiency: The use of only integer arithmetic (addition and subtraction) avoids computationally expensive floating-point calculations.
- Accuracy: It accurately selects the nearest pixel to the theoretical circle path.
- Simplicity: The algorithm is straightforward and easy to implement.
Conclusion
Bresenham’s Circle Drawing Algorithm is a highly efficient and precise method for drawing circles in computer graphics.