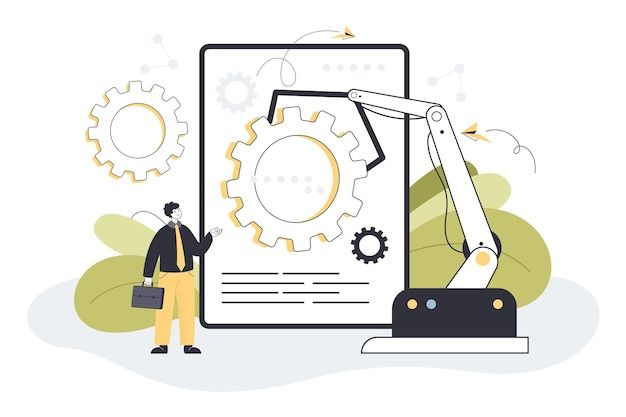
DDA (Digital Differential Analyzer) Algorithm Computer Graphics
- The Digital Differential Analyzer (DDA) algorithm is a straightforward method for scan-converting lines in computer graphics.
- It calculates the intermediate points between two given points (endpoints of a line) and plots them to draw the line.
let's understand the Digital Differential Analyzer (DDA) algorithm for scan-converting lines with illustration as follows:-
Example: Drawing a Line from (1, 1) to (5, 4)
1. Calculate Differences
- Determine the change in x (Δ x) and y (Δ y) between the endpoints (1, 1) and (5, 4).
- Δx (change in x) = X2-X1 i.e 5 - 1 = 4
- Δy (change in y) = Y2-Y1 i.e 4 - 1 = 3
2. Determine Steps
- Find the maximum of the absolute values of Δx and Δy to determine the number of steps needed for the algorithm.
- Steps = max(|Δx|, |Δy|) = max(4, 3) = 4
3. Calculate Increment Values
- Divide Δ x and Δ y by the number of steps to calculate the increments along the x and y directions, respectively.
- X-Increment = Δx / Steps = 4 / 4 = 1
- Y-Increment = Δy / Steps = 3 / 4 = 0.75
4. Initialize Current Points
- Start at (1, 1)
5. Scan Convert:
- Plot the point (1, 1).
- Update the current point:
1Initialize Current Points:
2Start at (1, 1)
3
4Iteration 1:
5 x_new = x_old + X-Increment = 1 + 1 = 2
6 y_new = y_old + Y-Increment = 1 + 0.75 = 1.75
7 Current Point: (2, 1.75)
8
9Iteration 2:
10 x_new = x_old + X-Increment = 2 + 1 = 3
11 y_new = y_old + Y-Increment = 1.75 + 0.75 = 2.5
12 Current Point: (3, 2.5)
13
14Iteration 3:
15 x_new = x_old + X-Increment = 3 + 1 = 4
16 y_new = y_old + Y-Increment = 2.5 + 0.75 = 3.25
17 Current Point: (4, 3.25)
18
19Iteration 4:
20 x_new = x_old + X-Increment = 4 + 1 = 5
21 y_new = y_old + Y-Increment = 3.25 + 0.75 = 4
22 Current Point: (5, 4)
23
24Iteration 5:
25 x_new = x_old + X-Increment = 5 + 1 = 6
26 y_new = y_old + Y-Increment = 4 + 0.75 = 4.75
27 Current Point: (6, 4.75)
28
- Repeat the process until reaching the endpoint (5, 4).
Visualization:
- (1, 1) -> (2, 1.75) -> (3, 2.5) -> (4, 3.25) -> (5, 4)
- The DDA algorithm essentially takes steps along the line, plotting points at each step.
- It calculates the increments needed in the x and y directions to distribute pixels along the line evenly.
- In this example, it draws a line from (1, 1) to (5, 4) by incrementing both x and y values in a smooth manner.
Conclusion
- The DDA algorithm efficiently calculates and plots intermediate points along a line, ensuring a smooth distribution of pixels.
- In the example above, it draws a line from (1, 1) to (5, 4) by continuously incrementing both x and y values.