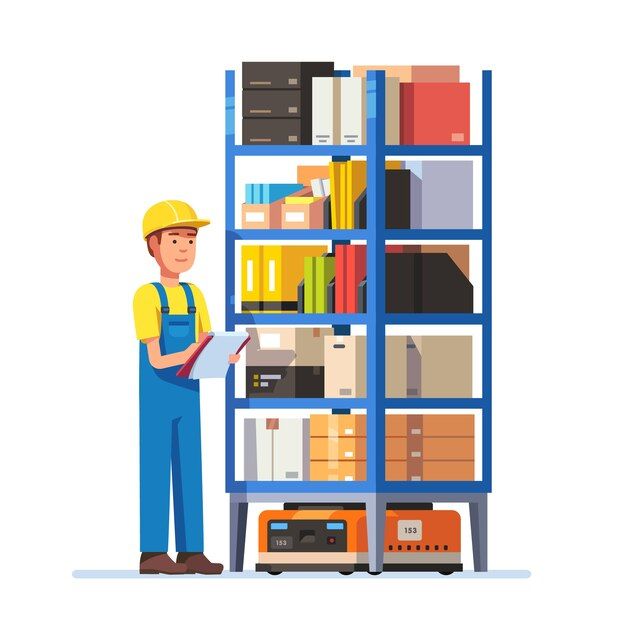
Storage Classes in C
What are Storage Classes in C?
- In C programming, stored classes determine the lifetime, scope and visibility of variables.
- There are four main storage classes: automatic, external (also known as global), static, and register.
Automatic Storage Class
- The automatic storage class, often referred to as the default storage class, is used for variables declared within a function.
- These variables are created when the function is called and destroyed when the function exits.
1#include <stdio.h>
2
3void displayMessage() {
4 int count = 10; // Automatic variable
5 printf("Count: %d\n", count);
6}
7
8int main() {
9 displayMessage();
10 // printf("%d\n", count); // This would result in an error
11 return 0;
12}
13
- In the code above, the variable count is an automatic variable.
- It is created when the displayMessage function is called and destroyed when the function exits.
- Attempting to access it outside the function would result in an error.
External (Global) Storage Class
- The external or global storage class is used for variables declared outside of any function.
- These variables have a longer lifetime and are accessible from any part of the program.
1#include <stdio.h>
2
3int globalVar = 20; // Global variable
4
5void displayMessage() {
6 printf("GlobalVar: %d\n", globalVar);
7}
8
9int main() {
10 displayMessage();
11 printf("GlobalVar: %d\n", globalVar);
12 return 0;
13}
14
- In this code, globalVar is a global variable.
- It can be accessed from both the display message function and the main function.
Static Storage Class
- The static storage class is used for variables with an extended lifetime.
- Static variables are initialized only once, and their values persist between function calls.
1#include <stdio.h>
2
3int globalVar = 20; // Global variable
4
5void displayMessage() {
6 printf("GlobalVar: %d\n", globalVar);
7}
8
9int main() {
10 displayMessage();
11 printf("GlobalVar: %d\n", globalVar);
12 return 0;
13}
14
- In this code, the variable count is a static variable.
- It is initialized only once and retains its value between calls to the displayCount function.
- This allows us to keep track of the count across function calls.
Register Storage Class
- The register storage class is used to suggest to the compiler that a variable should be stored in a CPU register for faster access.
- However, modern compilers often optimize variable storage, and this keyword's usage has become less relevant.
1#include <stdio.h>
2
3int main() {
4 register int x = 10; // Register variable (not guaranteed to be in a register)
5 int y = 20;
6
7 int sum = x + y;
8 printf("Sum: %d\n", sum);
9 return 0;
10}
11
Conclusion
- In C programming, the four main storage classes (automatic, external, static, and register) control the scope, lifetime, and accessibility of variables.
- These storage classes are crucial for efficient memory management and variable behavior in C programs.