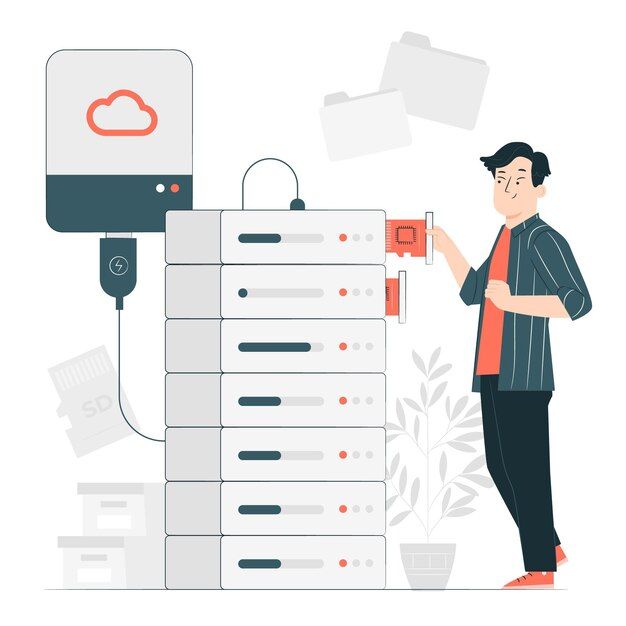
Structures and Union in C
Structures in C
- A structure is a composite data type in C that allows you to group together variables of different data types under a single name.
- Each variable in a structure is called a "member."
Structure Syntax:
1union union_name {
2 data_type member1;
3 data_type member2;
4 // ... additional members
5};
- struct: This keyword is used to define a structure.
- structure_name: Replace this with the name of the structure you want to define.
- { }: The curly braces enclose the members of the structure.
- data_type: Specifies the data type of each member.
- member1, member2, and so on: These are the names of the structure members.
Example
1#include <stdio.h>
2
3// Define a structure named "Person"
4struct Person {
5 char name[50];
6 int age;
7};
8
9int main() {
10 // Declare a variable of the "Person" structure type
11 struct Person person1;
12
13 // Initialize the structure members
14 strcpy(person1.name, "John");
15 person1.age = 30;
16
17 // Access and print the structure members
18 printf("Name: %s\n", person1.name);
19 printf("Age: %d\n", person1.age);
20
21 return 0;
22}
23
Unions
- A union is similar to a structure, but it only allocates memory for one member at a time.
- Unions are useful when you want to store different types of data in the same memory location.
Union Syntax:
1union union_name {
2 data_type member1;
3 data_type member2;
4 // ... additional members
5};
- union: This keyword is used to define a union.
- union_name: Replace this with the name of the union you want to define.
- { }: The curly braces enclose the members of the union.
- data_type: Specifies the data type of each member.
- member1, member2, and so on: These are the names of the union members.
Example
1#include <stdio.h>
2
3// Define a union named "Data"
4union Data {
5 int i;
6 float f;
7 char str[20];
8};
9
10int main() {
11 union Data data;
12
13 data.i = 10;
14 printf("Data as int: %d\n", data.i);
15
16 data.f = 3.14;
17 printf("Data as float: %f\n", data.f);
18
19 strcpy(data.str, "Hello, C!");
20 printf("Data as string: %s\n", data.str);
21
22 return 0;
23}
24
Enumerations
- An enumeration is a user-defined data type used to assign names to integral constants, making the code more readable.
Enumeration Syntax:
1enum enum_name {
2 enumerator1,
3 enumerator2,
4 // ... additional enumerators
5};
- enum: This keyword is used to define an enumeration.
- enum_name: Replace this with the name of the enumeration you want to define.
- { }: The curly braces enclose the list of enumerators.
- enumerator1, enumerator2, and so on: These are the names of the enumerators, which represent integral constants.
- By default, the first enumerator is assigned the value 0, and subsequent enumerators are incremented by 1.
For Example:
1#include <stdio.h>
2
3// Define an enumeration named "Day"
4enum Day {
5 Sunday, // 0
6 Monday, // 1
7 Tuesday, // 2
8 Wednesday, // 3
9 Thursday, // 4
10 Friday, // 5
11 Saturday // 6
12};
13
14int main() {
15 enum Day today = Wednesday;
16 printf("Today is %d\n", today);
17
18 return 0;
19}
20
Passing Structure to Functions
- You can pass structures as function parameters to manipulate and work with their data.
1#include <stdio.h>
2#include <string.h>
3
4// Define the "Person" structure
5struct Person {
6 char name[50];
7 int age;
8};
9
10// Function that takes a structure as a parameter
11void displayPerson(struct Person p) {
12 printf("Name: %s\n", p.name);
13 printf("Age: %d\n", p.age);
14}
15
16int main() {
17 struct Person person1;
18 strcpy(person1.name, "Alice");
19 person1.age = 25;
20
21 displayPerson(person1); // Pass the structure to the function
22
23 return 0;
24}
25
Arrays and Structures
- You can create arrays of structures to store multiple instances of structured data.
1include <stdio.h>
2#include <string.h>
3
4// Define the "Person" structure
5struct Person {
6 char name[50];
7 int age;
8};
9
10int main() {
11 // Create an array of "Person" structures
12 struct Person people[3];
13
14 // Initialize the array elements
15 strcpy(people[0].name, "John");
16 people[0].age = 30;
17
18 strcpy(people[1].name, "Alice");
19 people[1].age = 25;
20
21 strcpy(people[2].name, "Bob");
22 people[2].age = 22;
23
24 // Access and print array elements
25 for (int i = 0; i < 3; i++) {
26 printf("Name: %s, Age: %d\n", people[i].name, people[i].age);
27 }
28
29 return 0;
30}
31
Difference between Structure and Union
- The main difference is that structures allocate memory for all members
- while unions allocate memory for only one member at a time. Structures are used when you want to store data.
- simultaneously, whereas unions are used when you want to store different types of data in the same memory location.
Self-Referential Structure
- A self-referential structure contains a member that is a pointer
1#include <stdio.h>
2
3// Define a self-referential structure
4struct Node {
5 int data;
6 struct Node* next;
7};
8
9int main() {
10 // Creating nodes of a linked list
11 struct Node node1, node2, node3;
12
13 node1.data = 10;
14 node2.data = 20;
15 node3.data = 30;
16
17 node1.next = &node2;
18 node2.next = &node3;
19 node3.next = NULL;
20
21 // Traversing and printing the linked list
22 struct Node* current = &node1;
23 while (current != NULL) {
24 printf("Data: %d\n", current->data);
25 current = current->next;
26 }
27
28 return 0;
29}
30
Bit Fields
- Bit fields are used to allocate specific numbers of bits to each member of a structure, enabling efficient memory usage.
1#include <stdio.h>
2
3// Define a structure with bit fields
4struct Flags {
5 unsigned int flag1 : 1; // 1 bit for flag1
6 unsigned int flag2 : 2; // 2 bits for flag2
7 unsigned int flag3 : 3; // 3 bits for flag3
8};
9
10int main() {
11 struct Flags flags;
12
13 flags.flag1 = 1;
14 flags.flag2 = 2;
15 flags.flag3 = 3;
16
17 printf("flag1: %u\n", flags.flag1);
18 printf("flag2: %u\n", flags.flag2);
19 printf("flag3: %u\n", flags.flag3);
20
21 return 0;
22}
23
Conclusion
- In C programming, you can define structures and unions to group variables with different data types under a common name, with structures allocating memory for all members and unions allocating memory for one member at a time.
- Enumerations are used to create symbolic names for integral constants, enhancing code readability and manageability.