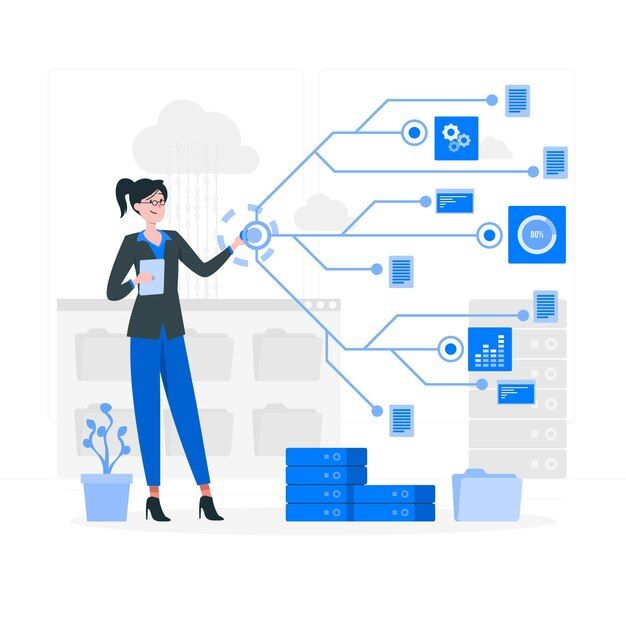
Functions in C
Why Functions are important?
- In C programming, functions are blocks of code that perform a specific task or a set of related tasks.
- Functions are essential for breaking down complex programs into smaller, more manageable parts, making the code more organized, modular, and easier to understand.
Function Declaration
- A function declaration tells the compiler about the function's name, return type, and parameters.
- It provides enough information to the compiler to allow it to check the function's usage in the program. Here's how you declare a function:
1// Function declaration
2int add(int a, int b);
3
In this example, we declare a function named add that takes two integer parameters and returns an integer.
Function Definition
- Function definition is where you provide the actual implementation of the function.
- It includes the code that gets executed when the function is called.
1// Function definition
2int add(int a, int b) {
3return a + b;
4
5}
In this code, we define the add function to take two integers as parameters and return their sum.
Function Scope
- Function scope refers to the area of the code where a variable is accessible.
- In C, variables declared within a function have local scope, meaning they are only accessible within that function.
1int globalVar = 10; // Global variable
2
3int main() {
4 int localVar = 5; // Local variable
5 // globalVar is accessible here
6 // localVar is accessible here
7 return 0;
8}
9
10void anotherFunction() {
11 // globalVar is accessible here
12 // localVar is NOT accessible here
13}
14
In the example above, globalVar is accessible within both main and another Function, but localVar is only accessible within main.
Recursion
- Recursion is a programming technique in which a function calls itself to solve a problem.
- Here is an example of a recursive function that calculates the factorial of a number:
1int factorial(int n) {
2 if (n <= 1) {
3 return 1;
4 } else {
5 return n * factorial(n - 1);
6 }
7}
8
The factorial function calls itself until n becomes 1, and then it returns the result.
Call by Value
- In C, function parameters are passed by value by default, which means a copy of the argument is passed to the function.
1void modify(int x) {
2 x = x * 2;
3}
4
5int main() {
6 int num = 5;
7 modify(num);
8 // num is still 5, not 10
9 return 0;
10}
11
The modify function modifies a copy of num, and the original num remains unchanged.
Call by Reference
- To modify the original values of variables within a function, you can use pointers.
- Here's an example of call by reference using pointers:
1void modify(int *x) {
2 *x = *x * 2;
3}
4
5int main() {
6 int num = 5;
7 modify(&num);
8 // num is now 10
9 return 0;
10}
11
In this case, we pass a pointer to num to the modify function, allowing it to change the original value.
Preprocessor Directives
- Preprocessor directives are commands that are executed by the C preprocessor before the code is compiled.
- They are indicated by # and are used for various purposes, including defining macros and conditional compilation.
#define
- The #define directive is used to create a macro.
- which is a way to replace a code snippet with a defined value or expression. Here's an example:
1#define MAX_VALUE 100
2
3int main() {
4 int number = 120;
5 if (number > MAX_VALUE) {
6 printf("Number is greater than %d\n", MAX_VALUE);
7 }
8 return 0;
9}
10
In this code, MAX_VALUE is defined as 100, and it is used in the if statement.
Macros with Arguments
- You can create macros that take arguments by using #define.
- Here's an example of a macro that calculates the square of a number:
1#define SQUARE(x) (x * x)
2
3int main() {
4 int result = SQUARE(5); // result is 25
5 return 0;
6}
7
The SQUARE macro takes an argument x and returns its square.
Nested Macros
- You can also nest macros within other macros.
Here's an example:
1#define SQUARE(x) (x * x)
2#define CUBE(x) (x * SQUARE(x))
3
4int main() {
5 int result = CUBE(3); // result is 27
6 return 0;
7}
8
In this code, the CUBE macro uses the SQUARE macro within its definition.
## Operator
- The ## operator in macros is used for token concatenation, allowing you to create new identifiers or values by combining tokens.
Here's an example:
1#define MAKE_IDENTIFIER(name) var_##name
2
3int main() {
4 int var_x = 10;
5 int MAKE_IDENTIFIER(y) = 20; // Creates a variable named var_y
6 return 0;
7}
8
In this code, the MAKE_IDENTIFIER macro combines "var_" with the argument to create a new variable name.
Conditional Compilation
- Conditional compilation allows you to include or exclude parts of code based on preprocessor directives.
For example:
1#define DEBUG
2
3int main() {
4#ifdef DEBUG
5 printf("Debug mode is enabled\n");
6#endif
7 return 0;
8}
9
Conclusion
- In C programming, functions are vital for code organization and modularity, simplifying complex tasks.
- Preprocessor directives and macros enhance flexibility and maintainability, ensuring efficient and adaptable code.