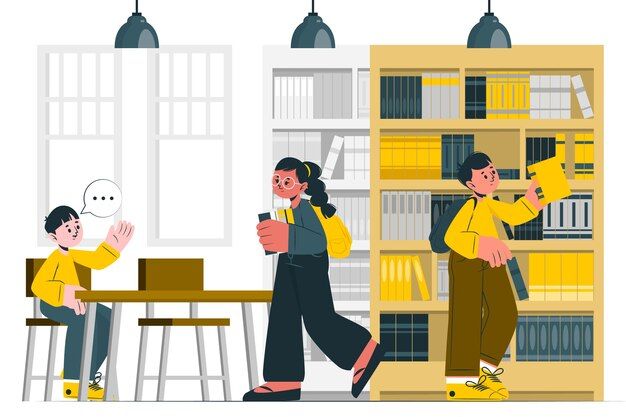
C Standard Library Functions
- C is a popular programming language that comes with a standard library that provides various functions for performing common tasks.
- These functions are included in various header files, each serving a specific purpose.
Standard Library Functions in C
- stdio.h
- stdlib.h
- conio.h
- ctype.h
- math.h
- string.h
<stdio.h> - Input/Output Functions
The stdio.h header file provides functions for standard input and output operations.
Example: Printing to the Console
1#include <stdio.h>
2
3int main() {
4 printf("Hello, World!\n");
5 return 0;
6}
7
Explanation:
- #include <stdio.h>: This line includes the standard I/O header.
- printf(): This function is used to print text to the console.
- "\n": Represents a newline character for formatting.
<stdlib.h> - Standard Library Functions
The stdlib.h header file provides functions for general-purpose tasks.
Example: Using rand() to Generate Random Numbers
1#include <stdio.h>
2#include <stdlib.h>
3#include <time.h>
4
5int main() {
6 srand(time(NULL)); // Seed the random number generator
7 int random_number = rand() % 100; // Generate a random number between 0 and 99
8 printf("Random Number: %d\n", random_number);
9 return 0;
10}
11
Explanation:
- srand(time(NULL)): Seeds the random number generator with the current time, ensuring different sequences of random numbers each time you run the program.
- rand(): Generates a random numbers
- % 100: Limits the range to 0-99.
<conio.h> - Console Input/Output Functions (DOS/Windows)
- Note: <conio.h> is not a part of the C standard library and is platform-specific.
- It's mainly used for older DOS/Windows environments. Modern compilers may not support it.
<ctype.h> - Character Handling Functions
- The ctype.h header file provides functions for character handling.
Example: Checking if a Character is a Digit
1#include <stdio.h>
2#include <ctype.h>
3
4int main() {
5 char ch = '7';
6 if (isdigit(ch)) {
7 printf("'%c' is a digit.\n", ch);
8 } else {
9 printf("'%c' is not a digit.\n", ch);
10 }
11 return 0;
12}
13
Explanation:
- isdigit (ch): Checks if the character is a digit.
<math.h> - Mathematical Functions
- The math.h header file provides mathematical functions.
Example: Calculating the Square Root
1#include <stdio.h>
2#include <math.h>
3
4int main() {
5 double number = 25.0;
6 double sqrt_result = sqrt(number);
7 printf("Square root of %lf is %lf\n", number, sqrt_result);
8 return 0;
9}
10
Explanation:
- sqrt(number): Computes the square root of a number.
<string.h> - String Functions
- The string.h header file provides functions for string manipulation.
Example: Concatenating Strings
1Example: Concatenating Strings
2#include <stdio.h>
3#include <string.h>
4
5int main() {
6 char str1[20] = "Hello, ";
7 char str2[] = "World!";
8 strcat(str1, str2); // Concatenate str2 to str1
9 printf("%s\n", str1);
10 return 0;
11}
12
Explanation:
- strcat(str1, str2): Concatenates str2 to the end of str1.
Command Line Arguments
- Command line arguments are parameters passed to a program when it's run in the terminal.
Example: Reading Command Line Arguments
1#include <stdio.h>
2
3int main(int argc, char *argv[]) {
4 if (argc < 2) {
5 printf("Usage: %s <your_argument>\n", argv[0]);
6 return 1;
7 }
8 printf("You provided: %s\n", argv[1]);
9 return 0;
10}
11
Conclusion
Now you have a basic understanding of some common standard library functions in C and how to use them, including working with command line arguments.