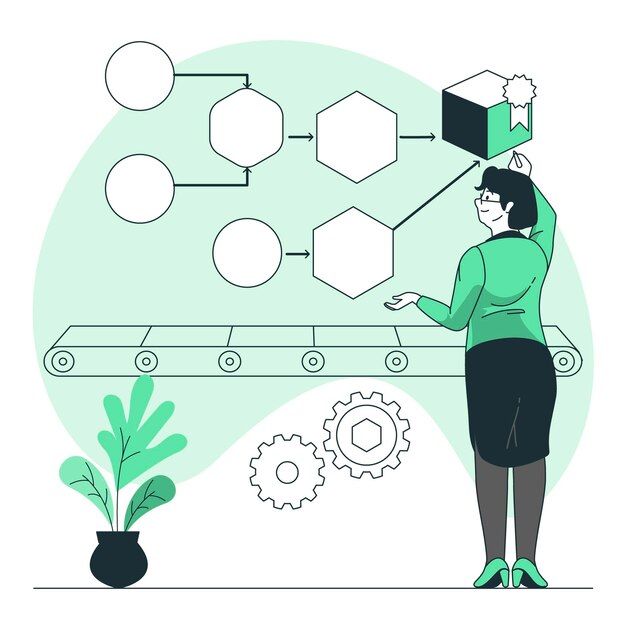
C Control Structures
- In C programming, control structures are used to control the flow of a program.
- They includes if statements, if...else statements, else if ladders.
- while loops, do...while loops, for loops, switch statements, nested control structures.
- break, labeled break, continue, labeled continue statements, exit statements, and goto statements.
1
The if statement is used to execute a block of code if a specified condition is true.
1#include <stdio.h>
2
3int main() {
4 int num = 5;
5
6 if (num > 0) {
7 printf("The number is positive.\n");
8 }
9
10 return 0;
11
In this code, the program checks if the value of num is greater than 0. If it is true, it prints "The number is positive."
2. if...else Statement
The if...else statement is used to execute one block of code if a condition is true and another block if the condition is false.
1#include <stdio.h>
2
3int main() {
4 int num = -2;
5
6 if (num > 0) {
7 printf("The number is positive.\n");
8 } else {
9 printf("The number is not positive.\n");
10 }
11
12 return 0;
13
Here, the program checks if num is greater than 0. If it is, it prints "The number is positive," otherwise, it prints "The number is not positive."
3. else if Ladder
An else if ladder is used to check multiple conditions in sequence.
1#include <stdio.h>
2
3int main() {
4 int num = 0;
5
6 if (num > 0) {
7 printf("The number is positive.\n");
8 } else if (num < 0) {
9 printf("The number is negative.\n");
10 } else {
11 printf("The number is zero.\n");
12 }
13
14 return 0;
15
In this example, the program checks if num is positive, negative, or zero and prints the appropriate message.
4. while Loop
The while loop is used to repeatedly execute a block of code as long as a condition is true.
1#include <stdio.h>
2
3int main() {
4 int i = 1;
5
6 while (i <= 5) {
7 printf("%d\n", i);
8 i++;
9 }
10
11 return 0;
12}
13
This code prints numbers from 1 to 5 by repeatedly executing the printf statement as long as i is less than or equal to 5.
5. do...while Loop
The do...while loop is similar to the while loop, but it always executes the block of code at least once before checking the condition.
1#include <stdio.h>
2int main() {
3int i = 1;
4do {
5printf("%d\n", i);
6i++;
7
8}
9while (i <= 5);
10return 0;
11
12}
13
14
In this example, the program prints numbers from 1 to 5, and even if the condition is initially false, it executes the loop once.
6. for loop
The for loop is used to iterate over a range of values with an initialization, condition, and increment expression.
1#include <stdio.h>
2
3int main() {
4 for (int i = 1; i <= 5; i++) {
5 printf("%d\n", i);
6 }
7
8 return 0;
9
This code accomplishes the same task as the previous examples by using a for loop. It iterates from 1 to 5 and prints the values.
7. switch Statement
The switch statement is used to select one of many code blocks to be executed based on a given expression.
1#include <stdio.h>
2
3int main() {
4 int choice = 2;
5
6 switch (choice) {
7 case 1:
8 printf("You chose option 1.\n");
9 break;
10 case 2:
11 printf("You chose option 2.\n");
12 break;
13 case 3:
14 printf("You chose option 3.\n");
15 break;
16 default:
17 printf("Invalid choice.\n");
18 }
19
20 return 0;
21}
In this example, the program prints a message based on the value of choice.
8. Nested Control Structures
Nested control structures are control structures within other control structures. For example, you can have an if statement inside a for loop.
1#include <stdio.h>
2
3int main() {
4 for (int i = 1; i <= 5; i++) {
5 if (i % 2 == 0) {
6 printf("%d is even.\n", i);
7 } else {
8 printf("%d is odd.\n", i);
9 }
10 }
11
12 return 0;
13}
14
This code uses a nested if statement inside a for loop to print whether a number is even or odd.
9. break Statement
The break statement is used to exit a loop prematurely.
1#include <stdio.h>
2
3int main() {
4 for (int i = 1; i <= 5; i++) {
5 if (i == 3) {
6 break;
7 }
8 printf("%d\n", i);
9 }
10
11 return 0;
12}
13
In this code, the for loop will terminate when i reaches 3 due to the statement.
10. Labeled break and continue Statements
You can label loops to use break and continue with specific loops in nested structures.
1#include <stdio.h>
2
3int main() {
4 for (int i = 1; i <= 3; i++) {
5 for (int j = 1; j <= 3; j++) {
6 if (j == 2) {
7 break; // This will break the inner loop.
8 }
9 printf("%d %d\n", i, j);
10 }
11 }
12
13 return 0;
14}
15
11. goto Statement
The goto statement is used to transfer control to a labeled statement.
1#include <stdio.h>
2
3int main() {
4 int i = 0;
5start:
6 if (i >= 5) {
7 goto end;
8 }
9
10 printf("%d\n", i);
11 i++;
12
13 goto start;
14
15end:
16 return 0;
17}
18
19
12. exit Statement
The exit statement is used to terminate a program.
1#include <stdio.h>
2#include <stdlib.h>
3
4int main() {
5 int num = 7;
6 if (num < 10) {
7 printf("Number is less than 10.\n");
8 exit(0); // Terminate the program.
9 }
10 printf("This will not be executed.\n");
11 return 0;
12}
13
Conclusion
In C programming, control structures such as if, for, while, switch and more enable developers to create organized and efficient code, facilitating conditional execution, looping, and decision-making in their programs.