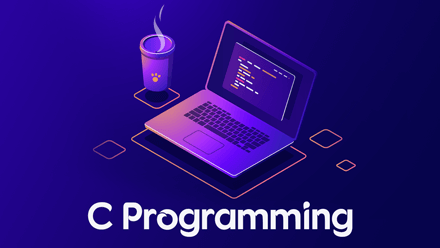
C Basics
- C is a general-purpose computer programming language.
- It was created in the 1970s by Dennis Ritchie, and remains very widely used and influential.
Key Points
- Structured Language: C is a structured programming language, allowing developers to write clear, organized, and efficient code.
- Low-Level and High-Level: It provides both low-level memory manipulation and high-level abstraction for creating a wide range of software.
- Portable: Code written in C is highly portable, meaning it can run on different platforms with minimal modification.
- Extensive Standard Library: C comes with a rich standard library that simplifies common programming tasks.
Structure of a Basic C Program
A basic C program consists of a main function, which is the entry point of the program.
Here's a simple structure.
1#include <stdio.h>
2intmain() {
3// Program logic goes here
4return 0;
5}
Writing and Executing the First C Program
Here's a basic "Hello, World!" program:
1#include <stdio.h>
2intmain() {
3printf("Hello, World!\n");
4return 0;
5}
- To execute the program, save it to a file with a .c extension (e.g., hello.c), and then compile and run it using a C compiler like gcc:gcc hello.c -o hello./hello
- This code prints "Hello, World!" to the console.
C Character Set
- In C, a character set is the collection of characters that are recognized by the language.
- The basic character set in C includes letters (both uppercase and lowercase), digits, and a few special characters like punctuation symbols. Here's a simple example that uses characters:
1#include <stdio.h>
2
3int main() {
4 char firstLetter = 'A';
5 char secondLetter = 'B';
6
7 printf("First letter: %c\n", firstLetter);
8 printf("Second letter: %c\n", secondLetter);
9
10 return 0;
11}
12
In this example, we've used the characters 'A' and 'B' to initialize two variables and then printed them using the %c format specifier.
Identifiers and Keywords
- Identifiers in C are names given to various program elements such as variables, functions, and arrays.
- They must start with a letter or an underscore and can be followed by letters, digits, or underscores. C also has a set of keywords, which are reserved words with predefined meanings.
Here's an example that demonstrates identifiers and keywords:
1include <stdio.h>
2
3int main() {
4 int number = 42; // 'number' is an identifier
5 if (number > 0) {
6 printf("The number is positive.\n"); // 'if' is a keyword
7 } else {
8 printf("The number is non-positive.\n"); // 'else' is a keyword
9 }
10
11 return 0;
12}
13
Data Types
- Data types in C specify the type of data a variable can hold.
- Common data types include int, char, float, and double. Here's an example using different data types:
1#include <stdio.h>
2
3int main()
4{
5 int age = 25;
6 char grade = 'A';
7 float price = 12.99;
8 double pi = 3.14159265359;
9
10 printf("Age: %d\n", age);
11 printf("Grade: %c\n", grade);
12 printf("Price: %.2f\n", price);
13 printf("Pi: %.10lf\n", pi);
14
15 return 0;
16}
17
Constants
- Constants are fixed values in C.
- They can be of various types, including integer constants, floating-point constants, and character constants.
- Here's an example with integer and character constants:
1#include <stdio.h>
2
3int main()
4{
5 const int numberOfMonths = 12; // Integer constant
6 const char firstLetter = 'A'; // Character constant
7
8 printf("Number of months in the year: %d\n", numberOfMonths);
9printf("First letter of the alphabet: %c\n", FirstLetter);
10 return 0;
11}
12
Symbolic Constants
- Symbolic constants are user-defined constants represented by identifiers.
- They are typically created using the #define preprocessor directive.
Here's an example:
1#include <stdio.h>
2
3#define PI 3.14159265359
4
5int main()
6{
7 double radius = 5.0;
8 double area = PI * radius * radius;
9
10 printf("The area of the circle: %.2lf\n", area);
11
12 return 0;
13}
14
In this example, we've defined a symbolic constant PI and used it to calculate the area of a circle.
Variable Declarations
- Variable declarations specify the data type and name of a variable.
Here's an example:
1#include <stdio.h>
2
3int main() {
4 int x; // Variable declaration
5
6 x = 10; // Variable initialization
7
8 printf("The value of x: %d\n", x);
9
10 return 0;
11}
12
In this example, we declare and initialize an integer variable x.
#include Preprocessor Directive
- The #include directive is used to include header files in a C program.
- It allows you to use functions and features defined in those header files. For example:
1#include <stdio.h>
2
3intmain() {
4// Code that uses functions from the 'stdio.h' header
5return 0;
6}
7
8
In this example, we include the stdio.h header to use functions like printf.
Expression Statements
Expression statements are statements that consist of expressions followed by a semicolon.
They perform some computation and may have side effects. Here's an example:
1#include <stdio.h>
2
3intmain()
4{
5int a = 5;
6int b = 3;
7int sum = a + b; // Expression statement
8printf("The sum of %d and %d is %d\n", a, b, sum);
9return 0;
10}
In this example, the expression a + b calculates the sum of two numbers.
Compound Statements
- Compound statements, also known as blocks, are enclosed within curly braces {} and can contain multiple statements.
- They are often used for grouping statements together.
1#include <stdio.h>
2int main()
3{
4int x = 10;
5int y = 5;
6if (x > y)
7{
8printf("x is greater than y.\n");
9}
10
11else
12{
13printf("x is not greater than y.\n");
14}
15
16return 0;
17}
Conclusion
C is a foundational, versatile programming language that combines low-level control with high-level capabilities, making it vital for systems programming and providing a strong foundation for developers.