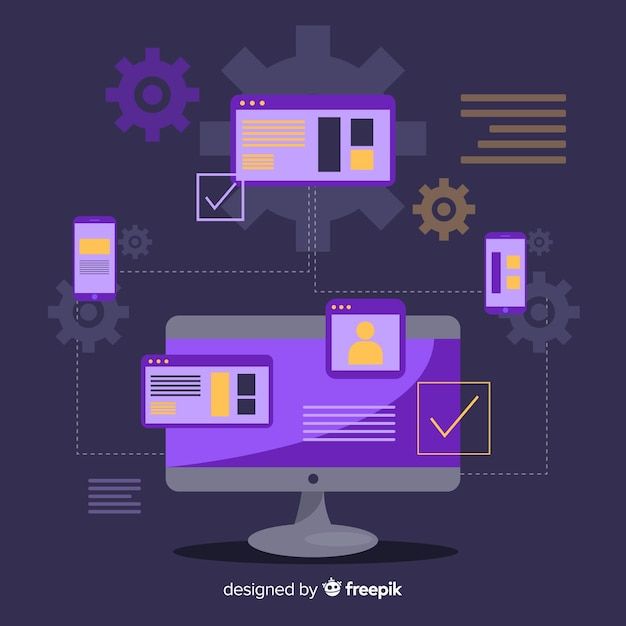
Semaphores in Os (Operating Systems) with example
What are Semaphores in Os?
- Semaphores are a synchronization mechanism introduced by Edsger Dijkstra in the late 1960s.
- Semaphores provide a way to achieve mutual exclusion and coordinate access to shared resources.
- Semaphores come in two main types: binary semaphores and counting semaphores.
What is Wait Operation in Semaphores?
- The "wait" operation, often denoted as P, is used by a process to request entry into a critical section or to acquire a resource.
- The steps involved in the wait operation are as follows:
1wait(M)
2{
3 while (M <= 0);
4 M--;
5}
- It checks if the semaphore value M is greater than 0.
- If M is not greater than 0, the process enters a loop, continuously checking the condition until it becomes true.
- Once the condition is true (i.e., M becomes greater than 0), the process decrements M and proceeds, indicating that it has acquired the semaphore.
If the Semaphore Value is Greater than 0:
- The process is allowed to proceed into the critical section or access the resource.
- The semaphore value is decremented by 1, indicating that one instance of the resource is now in use.
If the Semaphore Value is 0:
- The process is blocked, indicating that another process currently uses the resource.
- The process is placed in a waiting queue until the semaphore value becomes greater than 0.
What is Signal Operation in Semaphores?
The signal operation often denoted as V (from the Dutch word "verhogen," meaning "to increment" ), is used by a process to release a critical section or free a resource.
1signal(M)
2{
3 M++;
4}
- The signal operation increments the semaphore value M.
- Signal operation is typically used to release the semaphore, indicating that the shared resource or critical section is now available for other processes to access.
- If there are processes waiting for the semaphore (blocked by the wait operation), the increment allows one of them to proceed.
- The steps involved in the signal operation are as follows:
Release the Resource: The process leaves the critical section or releases the resource it was using. - Increment Semaphore Value: The semaphore value is incremented by 1, indicating that the resource is now available for other processes.
- Unblock a Waiting Process (if any): If there are processes waiting in the queue (blocked by the wait operation), one of them is unblocked, allowing it to proceed.
Binary Semaphores
- Binary semaphores are a type of semaphore that can take on only two values: 0 and 1.
- They are primarily used for managing mutual exclusion, where the objective is to control access to a critical section of code.
- The binary semaphore acts as a simple lock that can be either locked (1) or unlocked (0).
Key Characteristics: - Values:
- Binary semaphores can only have values 0 or 1.
- 0 typically signifies that the resource is unavailable or the critical section is occupied,
- while 1 indicates that the resource is available or the critical section is free.
- Mutual Exclusion: Binary semaphores are well-suited for scenarios where only one process should access a critical section at any given time.
- They help prevent race conditions by allowing or denying access to the critical section based on the current value of the semaphore.
- Operations: The standard operations associated with binary semaphores are "wait" (P operation) and "signal" (V operation).
Counting Semaphores
- Counting semaphores can take on non-negative integer values.
- They are used for managing resources where multiple instances can be available simultaneously.
- Counting semaphores provides a way to control access to a pool of resources rather than a single resource.
Key Characteristics: - Values: Counting semaphores can have values greater than 1, allowing multiple processes to access a resource simultaneously.
- Resource Management: Counting semaphores are suitable for scenarios where a fixed number of identical resources need to be shared among multiple processes.
- Operations: Similar to binary semaphores, counting semaphores use "wait" (P operation) and "signal" (V operation) operations.
Advantages of Semaphores
1. Synchronization
Semaphores help in controlling access to shared resources, preventing data corruption and race conditions.
2. Mutual Exclusion
Binary semaphores are particularly effective in achieving mutual exclusion, ensuring that only one process can access a critical section at a time.
3. Flexibility
Counting semaphores offers flexibility by allowing a specified number of processes to access a resource simultaneously.
4. Efficiency
Semaphores are efficient and can be implemented with minimal overhead, making them suitable for a wide range of applications.
5. Deadlock Prevention
Properly implemented, semaphores can help prevent deadlock situations by controlling the order in which processes access resources.
Disadvantages of Semaphores
1. Complexity
Working with semaphores can introduce complexity to code, potentially leading to harder-to-understand and maintain programs.
2. Programming Errors
Misuse of semaphores, such as failing to release a semaphore after use, can lead to subtle bugs that are difficult to detect.
3. Priority Inversion
Semaphores may contribute to priority inversion, where a low-priority task holds a semaphore needed by a high-priority task, causing delays.
4. Overhead
While generally efficient, semaphores introduce some overhead in terms of processing time and memory usage.
5. Resource Contention
In high-contention scenarios, the use of semaphores might lead to increased contention for resources, potentially impacting performance.