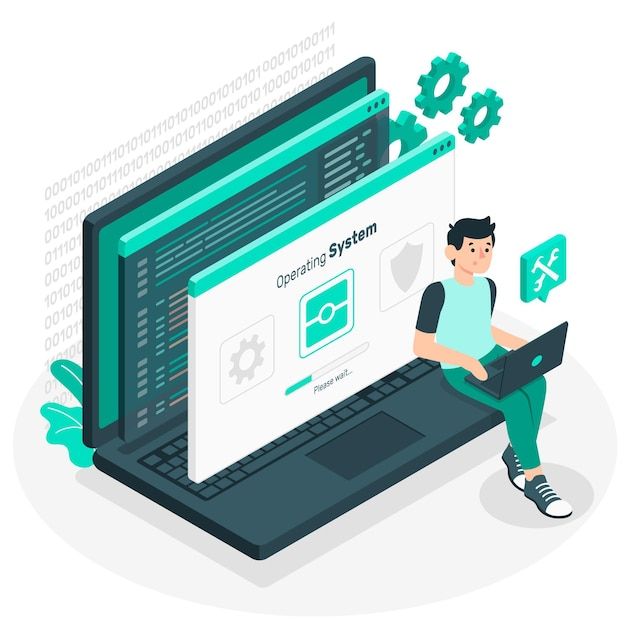
Linux File Management and File Security
What is Information Management in Linux?
- Information management in Linux programming involves organizing, storing, and retrieving data efficiently.
- One fundamental aspect is file management, where data is stored in files.
Creating Files in Linux
- In Linux, everything is treated as a file.
- A file is a collection of data stored under a particular name and a directory is a file that contains information about other files.
- Let's create a simple text file and display its content:
1echo "Hello, World!" > myfile.txt
Displaying File Content
1cat myfile.txt
File Access Methods in Os
File access methods in operating systems define how files are read, written, and manipulated by programs and users.
1. Sequential Access Method:
- In sequential access, files are processed in a linear or sequential manner from the beginning to the end.
- Access Pattern: Reading or writing operations are performed sequentially,
- and accessing data out of order may require scanning from the beginning.
- Use Cases: Suitable for situations where data is processed in a predictable order, such as reading log files or processing records.
Example:
11. Read record 1
2
32. Read record 2
4
53. Read record 3
6
7...
8
2. Direct Access Method (Random Access):
- Direct access allows direct and random access to any block of data within a file.
- Access Pattern: Data can be read or written directly at any position without the need to traverse the entire file sequentially.
- Use Cases: Useful for applications where data retrieval or modification is not sequential, such as databases.
Example:
11. Read block 5
2
32. Write data to block 10
4
53. Read block 3
3. Indexed Sequential Access Method (ISAM):
Overview:
- ISAM combines sequential and direct access by using an index to locate records in a file.
- Access Pattern: Sequential access within indexed segments, allowing for both sequential and direct access.
- Use Cases: Often used in database systems where the data is organized in index-sequential files.
Example:
11. Search index for key X
2
32. Retrieve record with key X
4
53. Move sequentially within the segment if needed
4. Hashed File Access Method:
- Hashing involves using a hash function to map keys to locations in a file, enabling rapid access.
- Access Pattern: Direct access based on a hash value generated from the key.
- Use Cases: Efficient for large databases where quick access to records based on a unique identifier is crucial.
Example:
11. Calculate hash value for key Y
2
32. Directly access the location based on the hash value
4
53. Handle collisions if necessary
Directory Structure in Os
Single-level Directory Structure
- The single-level directory structure is the most basic form, where all files reside in a single directory.
- This simple organization makes it easy to implement and understand.
- However, it has limitations when the system has multiple users or a growing number of files due to the requirement for unique file names.
Advantages:
- Fast Searching for Small Files: In cases where files are smaller in size, searching is faster due to the absence of nested directories.
Disadvantages:
- Search Time for Large Directories: As the directory grows, searching becomes time-consuming, affecting efficiency.
1/ (root directory)
2
3|
4
5├── file1
6
7├── file2
8
9├── file3
In this example, all files exist in the root directory without any subdirectories.
Two-level Directory Structure
- To address the limitations of the single-level structure, the two-level directory structure is introduced.
- It assigns each user their own directory, preventing conflicts in file names among different users.
Advantages
- Name Uniqueness: Users can have files with the same name without conflicts, improving organization.
- User-Specific Security: Security is enhanced as users can't access each other's files, ensuring data privacy.
- Efficient Searching:
- Searching for files becomes more efficient in this structured setup.
Disadvantages
- No Subdirectory Creation for Users: Users lack the ability to create subdirectories for further organization.
Example:
Consider a two-level directory structure:
1/ (root directory)
2
3|
4
5├── user1
6
7| ├── file1
8
9| ├── file2
10
11|
12
13├── user2
14
15├── file3
16
17├── file4
Here, each user has their own directory containing files.
Tree/Hierarchical Directory Structure
- The tree directory structure introduces a hierarchical organization, allowing users to create subdirectories and organize files more flexibly.
- This structure resembles an upside-down tree, with the root directory at the top.
Advantages
- Subdirectory Support: Users can create subdirectories, facilitating better organization of files.
- Improved Searching: Searching is easier due to the hierarchical arrangement of directories.
Disadvantages
- Limited File Sharing: Users are restricted from accessing other user's directories, limiting file sharing.
- Root Directory Access Limitation: Users cannot modify the root directory data.
Example:
Consider a tree directory structure:
1/ (root directory)
2
3|
4
5├── user1
6
7| ├── subfolder1
8
9| | ├── file1
10
11| | ├── file2
12
13| |
14
15| ├── file3
16
17|
18
19├── user2
20
21├── file4
22
23├── file5
In this example, users have their directories with subdirectories and files.
Acyclic Graph Directory Structure
- The acyclic graph directory structure allows file sharing between multiple users through links,
- addressing the limitation of previous structures where a file could only be accessed from the directory it was present in.
Advantages
- File and Directory Sharing: Allows sharing of files and directories among multiple users.
- Easy Searching: Searching becomes more efficient with shared resources.
Disadvantages
- File Deletion Complexity: Deleting a file requires removing all references, making permanent deletion complex.
Example:
Consider an acyclic graph directory structure:
1/ (root directory)
2
3|
4
5├── user1
6
7| ├── sharedfile
8
9|
10
11├── user2
12
13| ├── sharedfile (linked to user1/sharedfile)
14
15|
16
17├── user3
18
19├── sharedfile (linked to user1/sharedfile)
20
In this example, multiple users share the same file through links, allowing collaboration.
Navigating and Listing Contents
1mydirectory ls
2
This creates a directory named mydirectory and displays its contents.
File Protection in Linux Programming
- File protection involves setting permissions to control who can access or modify a file.
- In Linux, permissions are represented by three groups: owner, group, and others. Let's demonstrate setting file protection:
Displaying Current Permissions
1ls -l myfile.txt
Changing File Owner
1chown newowner myfile.txt
Here, newowner is the new owner of the file.
What is Chown?
The term "chown" stands for "change owner," and the primary purpose of this command is to change the owner of a file or directory.
The basic syntax of the chown command is as follows:
1chown [options] new_owner:new_group file(s)
- Options: Additional settings or flags that modify the behavior of the chown command.
- new_owner: The new owner to be assigned to the file or directory.
- new_group: The new group to assign to the file or directory.
- file(s): The name(s) of the file(s) or directory(ies) whose ownership you want to change.
Restricting Permissions
1chmod go-rwx myfile.txt
This removes read, write, and execute permissions for the group and others.
Linux File Security
Permission Types
In Linux, file security is governed by permissions, which control who can read, write, or execute a file. There are three main permission types:
- Read (r): Allows reading the contents of the file.
- Write (w): Permits modifying or deleting the file.
- Execute (x): Grants the ability to execute the file if it's a script or program.
Permissions are assigned to three entities:
- User (Owner): The person who owns the file.
- Group: A collection of users, often used to manage access among related users.
- Others: Anyone who doesn't fall into the user or group categories.
Examining Permissions
To examine the permissions of a file, you can use the ls command with the -l option. Let's take an example:
Displaying File Permissions
1ls -l myfile.txt
The output will show something like:
1-rw-r--r-- 1 user group 1024 Dec 14 12:00 myfile.txt
Changing Permissions - Symbolic Method
The chmod command is used to change permissions. The symbolic method allows modifying permissions using symbols.
Adding Write Permission for Group
1chmod g+w myfile.txt
This command adds write (w) permission for the group (g) in the file myfile.txt.
Removing Execute Permission for Others
1chmod o-x myfile.txt
This command removes execute (x) permission for others (o) in the file myfile.txt.
Changing Permissions - Numeric Method
The numeric method uses a three-digit octal number to represent permissions.
Each digit corresponds to the sum of read, write, and execute permissions:
- Read (r): 4
- Write (w): 2
- Execute (x): 1
Setting Read, Write, and Execute for Owner, Read for Group, None for Others
1chmod 741 myfile.txt
Here, 7 is for the owner (rwx), 4 is for the group (r--), and 1 is for others (--x).
Conclusion
We have Learned Linux file management: create, protect, and navigate files with chmod and chown and how to Efficiently organize and secure data with practical examples.