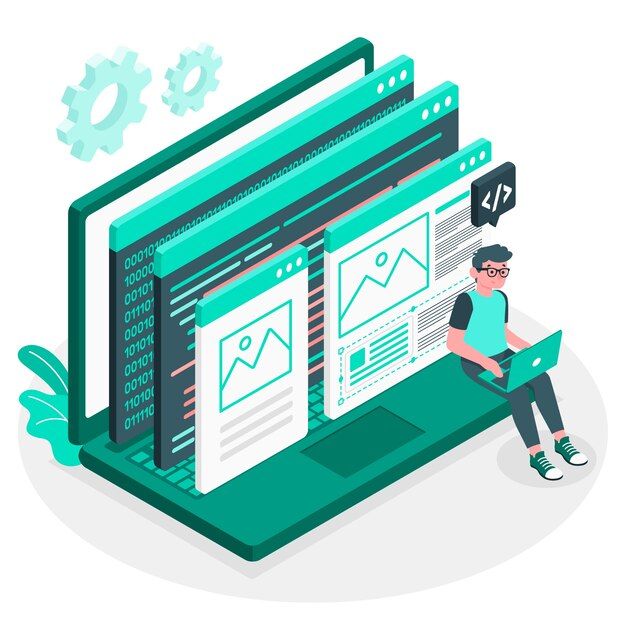
Process Synchronization in Operating Systems (Os) with examples
Process Synchronization involves ensuring that multiple processes can work together in a coordinated and orderly manner to avoid conflicts in the (OS) operating system.
How does Process Synchronization in OS work?
Let's understand how process synchronization works with examples as follows:-
- Example: Sharing a Printer
- Imagine you have two processes, Process A and Process B, both trying to print documents on a shared printer.
- To prevent conflicts and ensure orderly printing, process synchronization mechanisms come into play.
Need for Process Synchronization
- If there is no synchronization, both processes might attempt to send print jobs to the printer simultaneously.
- This can lead to race conditions, where the order of execution is unpredictable, and the printer may not handle the print jobs correctly from the above example.
- To address these issues, various synchronization mechanisms and algorithms have been developed.
What is Race Condition in the Operating System?
- In operating systems, a race condition is a situation where the behavior of a program depends on the timing or sequence of events, and the outcome is not deterministic.
- It occurs when multiple processes access shared resources concurrently, leading to unpredictable and often undesirable results.
Example: Sharing a Bank Account
- Consider a scenario where two processes, Process A and Process B, both have access to a shared bank account.
- Both processes can perform deposit and withdrawal operations on the account.
- A race condition may occur in the following way:
Race Condition Scenario:
- Process A checks the account balance, which is $100.
- Process B also checks the account balance simultaneously, still seeing $100.
- Process A decides to withdraw $50.
- Process B decides to withdraw $30.
- Both processes execute their withdrawal operations at the same time.
Undesired Result:
- Since both processes read the account balance before any withdrawals, they both believe there is enough money.
- Process A subtracts $50, making the new balance $50.
- Simultaneously, Process B subtracts $30, thinking the new balance is $70.
- In reality, the new balance should be $50 - $30 = $20, but due to the race condition, the final balance becomes $70.
In this example, the race condition arises because the processes did not properly coordinate their access to the shared resource (the bank account).
What is a Critical Section Problem?
- The Critical-Section Problem is a classic synchronization problem.
- It involves multiple processes or threads that need to access a shared resource, known as a critical section.
- The goal is to ensure that only one process can execute within the critical section at any given time, preventing conflicts and maintaining data integrity.
What are the Rules of Critical Sections?
To solve the Critical-Section Problem, we must meet the following rules:
- Mutual Exclusion: Only one process can be inside the critical section at a time.
- Progress: If no process is in the critical section and some processes wish to enter, only those processes not in the remainder section can participate in deciding which will enter next.
- Bounded Waiting: There is a limit on how long a process can be kept waiting before it enters the critical section.
Solutions to the Critical Section Problem:-
Peterson's Solution
- Peterson Solution was developed by Gary L. Peterson in 1981 and provides a way for two processes to safely access a critical section without the risk of race conditions.
- It uses two shared variables, turn and flag, to coordinate access to the critical section.
Key Components of Peterson's Solution
Flags
Two flags, often referred to as flag[0] and flag[1], are used to indicate whether a process is ready to enter its critical section.
Turn Variable
- A variable called turn determines which process gets to enter its critical section.
- If the turn is 0, Process 0 gets to enter, and if it's 1, Process 1 gets to enter.
Example:
- Let's consider two processes, Process 0 and Process 1, that want to enter a critical section.
- Peterson's Solution ensures that only one process enters the critical section at a time.
Initialization:
- flag[0] = flag[1] = 0 (both processes are not initially ready).
- turn = 0 (Process 0 is given the initial turn).
Process 0 Attempts to Enter Critical Section:
- Process 0 sets its flag[0] to 1, indicating its intention to enter the critical section.
- Process 0 sets turn to 1, signaling that it is Process 1's turn.
- Process 0 waits until either Process 1 is not ready.
- Once inside the critical section, Process 0 completes its task.
- Before leaving the critical section, Process 0 sets flag[0] to 0, indicating it is done.
Process 1 Attempts to Enter Critical Section:
- Process 1 sets its flag[1] to 1, indicating its intention to enter the critical section.
- Process 1 sets turn to 0, signaling that it is Process 0's turn.
- Process 1 waits until either Process 0 is not ready
- Once inside the critical section, Process 1 completes its task.
- Before leaving the critical section, Process 1 sets flag[1] to 0, indicating it is done.
Now let's understand with code example
1// Initialization
2int flag[2] = {0, 0}; // Flags for indicating process intention
3int turn = 0; // Variable to determine which process gets to enter
4
5// Process 0 Attempts to Enter Critical Section:
6flag[0] = 1; // Process 0 indicates its intention to enter the critical section
7turn = 1; // Process 0 signals that it's Process 1's turn
8while (flag[1] == 1 && turn == 1);
9// Critical Section (Process 0) - Perform tasks inside the critical section
10flag[0] = 0; // Process 0 is done with the critical section
11
12// Process 1 Attempts to Enter Critical Section:
13flag[1] = 1; // Process 1 indicates its intention to enter the critical section
14turn = 0; // Process 1 signals that it's Process 0's turn
15while (flag[0] == 1 && turn == 0);
16// Critical Section (Process 1) - Perform tasks inside the critical section
17flag[1] = 0; // Process 1 is done with the critical section
Note:
- However, Peterson's Solution is limited to two processes and may not be suitable for scenarios with more than two.
- In such cases, other synchronization mechanisms like semaphores are typically used.
Advantages of Peterson's Solution
- Simplicity: The algorithm is relatively simple and easy to understand. It uses only a few variables, making it accessible to programmers.
- Mutual Exclusion: It guarantees mutual exclusion, ensuring that only one process can access the critical section at a time. This property is crucial for preventing race conditions.
Disadvantages of Peterson's Solution
- Limited to Two Processes: The most significant limitation of Peterson's Solution is that it is designed for only two processes.
- It cannot be directly applied to scenarios with more than two processes.
- Non-Scalable: it does not scale well to situations involving multiple processes.
- Performance Impact: In scenarios where contention for the critical section is high, Peterson's Solution can introduce performance overhead due to the waiting and signaling mechanisms.
Conclusion
- Process synchronization is vital for orderly collaboration in operating systems.
- While Peterson's Solution ensures mutual exclusion for two processes, its simplicity comes with limitations, making it less suitable for scenarios with more processes.