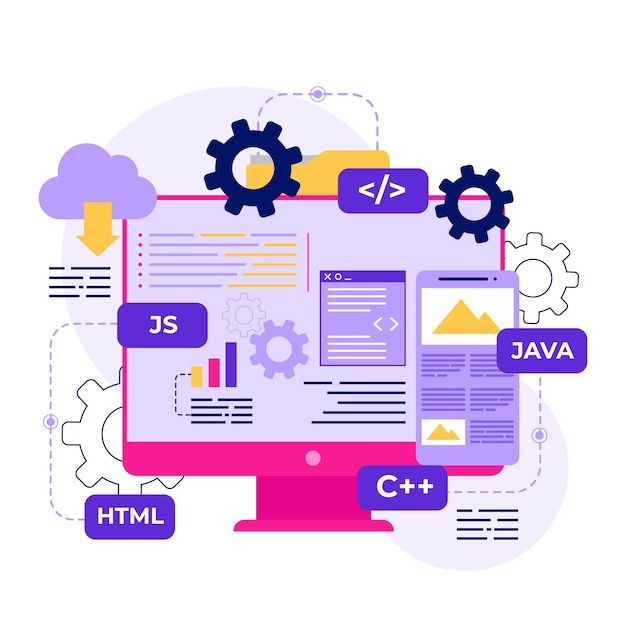
Swings Fundamentals: Building User Interfaces with Java Swing
1. What is Java Swing?
- Java Swing is a part of the Java Foundation Classes (JFC) and provides a platform-independent way to create GUIs for Java applications.
- It offers a wide range of components, layout managers, and event-handling mechanisms to build interactive and visually appealing user interfaces.
2. Swing Components
2.1. JLabel and ImageIcon
JLabel is a simple component used to display a non-editable text or image. ImageIcon allows you to display images easily within your Swing application.
1// Code example for displaying an image using JLabel and ImageIcon
2import javax.swing.*;
3
4public class ImageDisplayExample {
5 public static void main(String[] args) {
6 JFrame frame = new JFrame("Image Display");
7 ImageIcon icon = new ImageIcon("path/to/your/image.jpg");
8 JLabel label = new JLabel(icon);
9 frame.add(label);
10 frame.pack();
11 frame.setVisible(true);
12 }
13}
14
2.2. Using Swing Buttons
Swing provides various types of buttons like JButton, JToggleButton, JCheckBox, and JRadioButton, allowing users to interact with your application.
1// Code example for creating a JButton
2import javax.swing.*;
3
4public class JButtonExample {
5 public static void main(String[] args) {
6 JFrame frame = new JFrame("JButton Example");
7 JButton button = new JButton("Click Me!");
8 frame.add(button);
9 frame.pack();
10 frame.setVisible(true);
11 }
12}
13
2.3. JTextField
JTextField is used to create a single-line input field where users can enter text.
1// Code example for creating a JTextField
2import javax.swing.*;
3
4public class JTextFieldExample {
5 public static void main(String[] args) {
6 JFrame frame = new JFrame("JTextField Example");
7 JTextField textField = new JTextField(20);
8 frame.add(textField);
9 frame.pack();
10 frame.setVisible(true);
11 }
12}
13
2.4. JScrollPane
JScrollPane provides a scrollable view of a component that may be too large to fit in its container.
1// Code example for creating a JScrollPane
2import javax.swing.*;
3
4public class JScrollPaneExample {
5 public static void main(String[] args) {
6 JFrame frame = new JFrame("JScrollPane Example");
7 JTextArea textArea = new JTextArea(10, 20);
8 JScrollPane scrollPane = new JScrollPane(textArea);
9 frame.add(scrollPane);
10 frame.pack();
11 frame.setVisible(true);
12 }
13}
14
2.5. JList
JList is used to display a list of items from which users can make selections.
1// Code example for creating a JList
2import javax.swing.*;
3
4public class JListExample {
5 public static void main(String[] args) {
6 JFrame frame = new JFrame("JList Example");
7 String[] data = { "Item 1", "Item 2", "Item 3" };
8 JList<String> list = new JList<>(data);
9 frame.add(list);
10 frame.pack();
11 frame.setVisible(true);
12 }
13}
14
2.6. JComboBox
JComboBox is a drop-down list that allows users to select an item from a predefined set of choices.
1// Code example for creating a JComboBox
2import javax.swing.*;
3
4public class JComboBoxExample {
5 public static void main(String[] args) {
6 JFrame frame = new JFrame("JComboBox Example");
7 String[] data = { "Option 1", "Option 2", "Option 3" };
8 JComboBox<String> comboBox = new JComboBox<>(data);
9 frame.add(comboBox);
10 frame.pack();
11 frame.setVisible(true);
12 }
13}
14
3. Swing Containers and Layout Managers
Swing containers, like JFrame, JPanel, and JDialog, are used to hold and organize Swing components. Layout managers help in defining how components are arranged within a container.
4. Event Delegation Model
The Event Delegation Model in Swing enables efficient event handling by allowing components to delegate event processing to other objects.
5. Event Handling in Swing
5.1. Event Sources
In Swing, event sources are objects that generate events, such as buttons, checkboxes, and text fields.
5.2. Event Listeners
Event listeners are objects that listen to specific types of events and respond to them.
5.3. Event Classes and Interfaces
Swing provides various event classes and interfaces to handle different types of events.
5.4. Adapter Classes
Adapter classes are used to simplify event listener implementations by providing default implementations for all listener methods.
6. JDBC (Java Database Connectivity)
JDBC allows Java applications to interact with relational databases, enabling data retrieval, manipulation, and storage.
6.1. JDBC Architecture
JDBC follows a layered architecture, consisting of the JDBC API, DriverManager, Driver, and Database.
6.2. JDBC Drivers
JDBC supports different types of drivers to connect to various databases, such as Type 1, Type 2, Type 3, and Type 4 drivers.
6.3. Establishing a Connection
To connect to a database, we use the DriverManager.getConnection() method.
1// Code example for establishing a JDBC connection
2import java.sql.*;
3
4public class JdbcConnectionExample {
5 public static void main(String[] args) {
6 String jdbcUrl = "jdbc:mysql://localhost:3306/mydatabase";
7 String username = "myuser";
8 String password = "mypassword";
9
10 try {
11 Connection connection = DriverManager.getConnection(jdbcUrl, username, password);
12 System.out.println("Connection established successfully!");
13 connection.close();
14 } catch (SQLException e) {
15 e.printStackTrace();
16 }
17 }
18}
19
6.4. Executing Statements
The Statement and PreparedStatement interfaces are used to execute SQL queries and updates.
1// Code example for executing a SQL query using PreparedStatement
2import java.sql.*;
3
4public class JdbcPreparedStatementExample {
5 public static void main(String[] args) {
6 String jdbcUrl = "jdbc:mysql://localhost:3306/mydatabase";
7 String username = "myuser";
8 String password = "mypassword";
9
10 try {
11 Connection connection = DriverManager.getConnection(jdbcUrl, username, password);
12 String sqlQuery = "SELECT * FROM employees WHERE department = ?";
13 PreparedStatement preparedStatement = connection.prepareStatement(sqlQuery);
14 preparedStatement.setString(1, "IT");
15
16 ResultSet resultSet = preparedStatement.executeQuery();
17 // Process the result set
18 resultSet.close();
19 preparedStatement.close();
20 connection.close();
21 } catch (SQLException e) {
22 e.printStackTrace();
23 }
24 }
25}
26
6.5. Handling Results
The Result Set interface is used to handle query results.
7. Putting It All Together: Building a Simple Java Swing Application
Now, let's build a simple Java Swing application that interacts with a MySQL database using JDBC. In this application, we'll create a form where users can input their information, and the data will be saved in the database.
1// Code example for building a simple Java Swing application with JDBC integration
2import javax.swing.*;
3import java.awt.*;
4import java.awt.event.*;
5import java.sql.*;
6
7public class SimpleSwingJdbcApp extends JFrame {
8 private JTextField nameField;
9 private JTextField emailField;
10 private JButton saveButton;
11
12 public SimpleSwingJdbcApp() {
13 super("Simple Swing JDBC Application");
14
15 nameField = new JTextField(20);
16 emailField = new JTextField(20);
17 saveButton = new JButton("Save");
18 saveButton.addActionListener(new SaveButtonListener());
19
20 JPanel panel = new JPanel();
21 panel.setLayout(new GridLayout(3, 2));
22 panel.add(new JLabel("Name:"));
23 panel.add(nameField);
24 panel.add(new JLabel("Email:"));
25 panel.add(emailField);
26 panel.add(saveButton);
27
28 add(panel);
29 pack();
30 setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
31 setVisible(true);
32 }
33
34 private class SaveButtonListener implements ActionListener {
35 @Override
36 public void actionPerformed(ActionEvent e) {
37 String name = nameField.getText();
38 String email = emailField.getText();
39
40 try {
41 Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "myuser", "mypassword");
42 String sqlQuery = "INSERT INTO users (name, email) VALUES (?, ?)";
43 PreparedStatement preparedStatement = connection.prepareStatement(sqlQuery);
44 preparedStatement.setString(1, name);
45 preparedStatement.setString(2, email);
46
47 int rowsInserted = preparedStatement.executeUpdate();
48 if (rowsInserted > 0) {
49 JOptionPane.showMessageDialog(null, "User saved successfully!");
50 }
51
52 preparedStatement.close();
53 connection.close();
54 } catch (SQLException ex) {
55 ex.printStackTrace();
56 }
57 }
58 }
59
60 public static void main(String[] args) {
61 new SimpleSwingJdbcApp();
62 }
63}
64
8. Conclusion
we covered the fundamentals of Java Swing, including various Swing components, layout managers, event handling, and JDBC integration.