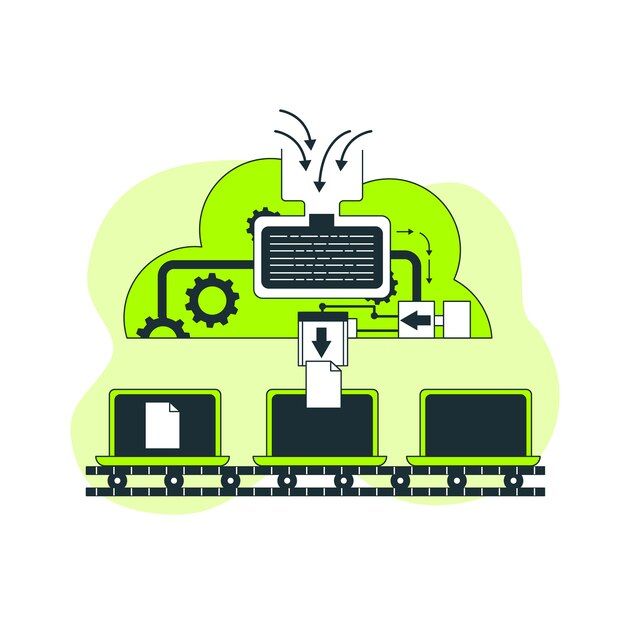
Elementary Concepts of Input/Output and Multithreading
Using I/O: Elementary Concepts of Input/Output
In this article, we will delve into the fundamental concepts of Input/Output (I/O) in programming. Specifically, we will explore the usage of byte streams and character-based streams for reading and writing data. Additionally, we'll cover multi-threaded programming and discuss multithreading fundamentals, the Thread class, the Runnable interface, thread life cycles, thread creation, and synchronization.
Byte Streams - Reading and Writing
When working with I/O in programming, understanding byte streams is crucial. A byte stream represents a sequence of bytes and is often used to read from or write to binary data. In Java, two main classes handle byte stream I/O: InputStream and OutputStream.
To read data from a file using byte streams, you can use the File Input Stream class. For example:
1try (FileInputStream fis = new FileInputStream("example.txt")) {
2 int byteData;
3 while ((byteData = fis.read()) != -1) {
4 // Process the byte data
5 }
6} catch (IOException e) {
7 // Handle the exception
8}
9
Likewise, to write data to a file using byte streams, you can use the FileOutputStream class:
1try (FileOutputStream fos = new FileOutputStream("output.txt")) {
2 byte[] data = "Hello, world!".getBytes();
3 fos.write(data);
4} catch (IOException e) {
5 // Handle the exception
6}
Automatic File Closure
In the examples above, you might have noticed the use of the try-with-resources statement. This feature, introduced in Java 7, automatically closes the resources (in this case, the file streams) after they are no longer needed. It ensures that resources are properly released, even in the event of an exception, thus promoting cleaner and more efficient code.
Character-Based Streams
While byte streams are suitable for binary data, character-based streams are used for handling character data, such as text files. In Java, the two main classes responsible for character-based I/O are Reader and Writer.
To read character data from a file, you can use the 'FileReader' class:
1try (FileReader reader = new FileReader("example.txt")) {
2 int charData;
3 while ((charData = reader.read()) != -1) {
4 // Process the character data
5 }
6} catch (IOException e) {
7 // Handle the exception
8}
9
For writing character data to a file, you can use the 'FileWriter' class:
1try (FileWriter writer = new FileWriter("output.txt")) {
2 writer.write("Hello, world!");
3} catch (IOException e) {
4 // Handle the exception
5}
6
File I/O using Character Streams
When it comes to reading and writing character data, character streams offer benefits such as ease of use and better handling of character encoding. The FileReader and FileWriter classes automatically handle character encoding, making it simpler to work with text-based files.
1import java.io.*;
2
3public class FileIOExample {
4 public static void main(String[] args) {
5 // Reading from a file using FileReader and BufferedReader
6 try (FileReader fr = new FileReader("example.txt");
7 BufferedReader br = new BufferedReader(fr)) {
8 String line;
9 while ((line = br.readLine()) != null) {
10 // Process each line of text
11 }
12 } catch (IOException e) {
13 // Handle the exception
14 }
15
16 // Writing to a file using FileWriter and BufferedWriter
17 try (FileWriter fw = new FileWriter("output.txt");
18 BufferedWriter bw = new BufferedWriter(fw)) {
19 String data = "Hello, world!";
20 bw.write(data);
21 } catch (IOException e) {
22 // Handle the exception
23 }
24 }
25}
26
Multi-threaded Programming: Introduction to Multithreading
A program can run multiple threads at once thanks to the potent technique of multithreaded programming. Threads are lightweight processes within a program, each performing specific tasks independently. Java provides built-in support for multithreading using the Thread class and the Runnable interface.
The Thread Class and Runnable Interface
The Thread class represents an individual thread of execution. To create a new thread, you can either extend the Thread class or implement the Runnable interface. Implementing the Runnable interface is generally preferred, as it allows you to separate the thread's behavior from its identity.
1public class MyRunnable implements Runnable {
2 @Override
3 public void run() {
4 // Thread behavior goes here
5 }
6}
7
8public class ThreadExample {
9 public static void main(String[] args) {
10 MyRunnable myRunnable = new MyRunnable();
11 Thread thread = new Thread(myRunnable);
12 thread.start();
13 }
14}
15
Thread Life Cycle and Thread Methods
A thread undergoes several states during its life cycle, including NEW, RUNNABLE, BLOCKED, WAITING, TIMED_WAITING, and TERMINATED. The Thread class provides various methods to interact with threads, such as start(), sleep(), join(), yield(), and interrupt().
Synchronization
Synchronization is essential when multiple threads access shared resources concurrently. In Java, synchronization can be achieved using synchronized methods or synchronized statements.
1public class SynchronizedCounter {
2 private int count = 0;
3
4 public synchronized void increment() {
5 count++;
6 }
7
8 // Other methods and code...
9}
10
Thread Life Cycle and Thread Methods
In multi-threaded programming, understanding the life cycle of a thread and the available thread methods is crucial for effectively managing threads. Let's explore the thread life cycle and the common thread methods with beginner-friendly code examples and proper explanations.
Thread Life Cycle
A thread in Java undergoes various states during its life cycle, each representing a different stage of its execution. The main states of a thread are as follows:
NEW: The topic is in "NEW" state when created. At this point, the thread object has been instantiated, but the thread's 'start()' method has not yet been called.
RUNNABLE: After calling the 'start()' method on the thread object, it enters the "RUNNABLE" state. In this state, the thread is ready to run but may or may not be currently executing, as it depends on the scheduler.
BLOCKED: A thread may enter the "BLOCKED" state while waiting to be locked. This happens when a synchronized block or method is being executed by another thread, and the current thread needs to wait for the lock to be released.
WAITING: Threads enter the "WAITING" state when they explicitly wait for another thread to notify them. This can happen when a thread calls the 'wait()' method on an object and waits for another thread to call the 'notify()' or 'notifyAll()' methods.
TIMED_WAITING: Similar to the "WAITING" state, threads enter the "TIMED_WAITING" state when they wait for a specified amount of time. For example, when a thread calls 'Thread.sleep()' or waits for a specific period using 'wait()'.
TERMINATED: Finally, when a thread completes its execution or is terminated due to an exception, it enters the "TERMINATED" state.
Thread Methods
Java provides several methods to interact with threads and control their behavior. Here are some of the common thread methods:
1.
The start() method is used to start the thread's execution. It schedules the thread to run, and the actual execution of the 'run()' method associated with the thread happens concurrently.
1public class MyThread extends Thread {
2 @Override
3 public void run() {
4 // Thread behavior goes here
5 }
6}
7
8public class Main {
9 public static void main(String[] args) {
10 MyThread myThread = new MyThread();
11 myThread.start(); // Start the thread's execution
12 }
13}
14
2.
The sleep() method allows a thread to pause its execution for a specified amount of time. This can be helpful for introducing delays or timing in your program.
1public class MyThread extends Thread {
2 @Override
3 public void run() {
4 try {
5 Thread.sleep(1000); // Pause the thread for 1 second (1000 milliseconds)
6 } catch (InterruptedException e) {
7 // Handle the exception
8 }
9 // Continue with thread behavior after the pause
10 }
11}
12
3.
The join() method is used to make one thread wait for another thread to complete its execution. This is useful when you want to ensure that a certain thread finishes before proceeding with the rest of the program.
1public class MyThread extends Thread {
2 @Override
3 public void run() {
4 // Some time-consuming task
5 }
6}
7
8public class Main {
9 public static void main(String[] args) {
10 MyThread myThread = new MyThread();
11 myThread.start();
12 try {
13 myThread.join(); // Wait for myThread to complete before proceeding
14 } catch (InterruptedException e) {
15 // Handle the exception
16 }
17 // Continue with the rest of the program
18 }
19}
20
4. yield()
The yield() method is used to give the scheduler a hint that the current thread is willing to yield its current time slice. This allows other threads to run, but there is no guarantee that the scheduler will honor the yield request.
1public class MyThread extends Thread {
2 @Override
3 public void run() {
4 // Some task
5 Thread.yield(); // Yield the current time slice to other threads
6 // Continue with the thread behavior after the yield
7 }
8}
9
5. interrupt()
The interrupt() method is used to interrupt a sleeping or waiting thread. It sets the interrupted status of the thread, allowing it to exit from sleep or wait for state.
1public class MyThread extends Thread {
2 @Override
3 public void run() {
4 try {
5 while (!Thread.currentThread().isInterrupted()) {
6 // Do some work
7 }
8 } catch (InterruptedException e) {
9 // Handle the exception or cleanup if necessary
10 }
11 }
12}
13
14public class Main {
15 public static void main(String[] args) {
16 MyThread myThread = new MyThread();
17 myThread.start();
18 // Some time later, interrupt the thread
19 myThread.interrupt();
20 }
21}
22
Conclusion
In this section, we learned about the life cycle of a thread and the common thread methods in Java. Understanding how threads transition through different states and how to control their behavior is essential for writing efficient multi-threaded programs.
By using these thread methods wisely, you can ensure proper synchronization, handle delays, and manage thread execution effectively. Properly managing threads will lead to more responsive and efficient programs, making your applications more reliable and user-friendly.
By using these thread methods wisely, you can ensure proper synchronization, handle delays, and manage thread execution effectively. Properly managing threads will lead to more responsive and efficient programs, making your applications more reliable and user-friendly.