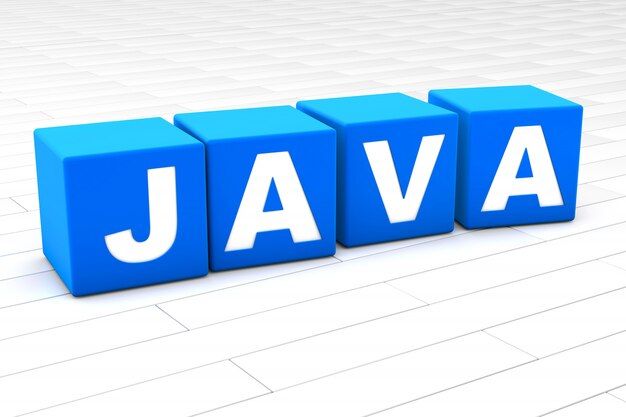
what is Java Programming?
What is Java?
Java is a versatile and widely used programming language. Java is known for its platform independence, as code written in Java can run on various operating systems and devices with the help of the Java Virtual Machine.
History of Java
Java Origins: Developed by James Gosling and his team at Sun Microsystems during the early 1990s, Java originally targeted TV interviews.
However, it evolved into a versatile, platform-friendly programming language known for its "write once, run anywhere" (WORA) philosophy. Java was publicly released in 1995 and has become one of the most popular and widely used languages globally.
Features of Java
Java's success can be attributed to its significant features, including:
1. Platform Independence
Java programs are compiled into bytecode, and executed by the Java Virtual Machine (JVM), allowing them to run on any device with a JVM. This makes Java programs inherently platform-independent.
2. Object-Oriented Approach
Java adheres to the Object-Oriented Programming (OOP) paradigm, empowering developers to create modular, reusable, and extensible systems using classes and objects.
3. Robustness and Security
Java's robustness comes from strong type checking, automatic memory management via garbage collection, and robust exception handling. It also incorporates built-in security mechanisms, making it suitable for secure application development.
4. Multi-Threading Support
Java supports multithreading, allowing developers to create applications that execute multiple tasks concurrently, enhancing performance and responsiveness.
5. Rich Standard Library
Java includes an extensive library of predefined classes and methods that simplify common programming tasks.
6. High Performance
The introduction of the Just-In-Time (JIT) compiler has improved Java's performance over time, making it a preferred choice for performance-critical tasks.
Difference between Java and C++
Java and C++ are powerful programming languages, but they have differences:
1. Platform Independence
Java achieves platform independence through bytecode and the JVM, while C++ programs are compiled into platform-specific machine code.
2. Memory Management
Java features automatic memory management via garbage collection, while C++ requires manual memory management using "new" and "delete" operators.
3. Pointers
C++ supports pointers for direct memory manipulation, introducing memory errors and vulnerabilities. Java eliminates direct memory manipulation for a safer environment.
4. Multiple Inheritance
C++ allows multiple inheritance, where a class inherits from multiple base classes. Java supports single inheritance and uses interfaces for achieving similar behavior.
Java Architecture
To understand Java's functioning, one must grasp its architecture, comprising three main components: Java Development Kit (JDK), Java Virtual Machine (JVM), and Java Runtime Environment (JRE).
1. Java Development Kit (JDK)
JDK is essential for coding, compiling, and executing Java programs. It includes tools like Java compiler (javac) and Java debugger (jdb).
2. Java Virtual Machine (JVM)
JVM is a core component that executes Java bytecode and converts it into platform-specific machine code.
3. Java Runtime Environment (JRE)
JRE contains essential libraries and resources required to run the JVM and Java programs.
Java Tokens
Java programs consist of various tokens, including data types, keywords, variables, lengths, and constants.
1. Data Types in Java
Java supports both primitive and non-primitive data types. Primitive data types include int, float, double, char, boolean, byte, short, and long.
1// Primitive data types
2int age = 19;
3float salary = 45000.50f;
4double pi = 3.14159;
5char grade = 'A';
6boolean isStudent = true;
7byte numBytes = 100;
8short numShort = 30000;
9long numLong = 1234567890L;
10
11// Non-primitive data types
12int[] numbers = {16, 24, 32, 40, 56};
13String name = "Sehaj Bindra";
14
2. Literals in Java
Literals are fixed values assigned to variables, such as numeric literals, character literals, boolean literals, and string literals.
1// Numeric literals
2int num1 = 10;
3double num2 = 3.14;
4float num3 = 2.5f;
5
6// Character literals
7char ch = 'A';
8
9// Boolean literals
10boolean isJavaFun = true;
11
12// String literals
13String message = "Hello, World!";
3. Variables in Java
Variables store data during program execution and must be declared with a specific data type.
1// Declare and initialize variables
2int num1 = 10;
3double pi = 3.14159;
4String name = "Manpreet";
5
6// Update the value of a variable
7num1 = 20;
8name = "Japanjot";
9
4. Scope and Lifetime of Variables
A variable's scope determines where it can be accessed within the program, affecting its lifetime and visibility.
1public class ScopeExample {
2// Global variable with class scope
3private int globalVar = 10;
4
5public void myMethod() {
6// Local variable with method scope
7int localVar = 5;
8System.out.println("Local variable: " + localVar);
9 }
10
11public void anotherMethod() {
12 // Accessing global variable
13System.out.println("Global variable: " + globalVar);
14 }
15}
5. Operators in Java
Java supports various operators, such as arithmetic, relational, logical, and bitwise operators, enabling a wide range of operations.
1int num1 = 10;
2int num2 = 5;
3
4// Arithmetic operators
5int sum = num1 + num2;
6int difference = num1 - num2;
7int product = num1 * num2;
8int quotient = num1 / num2;
9int remainder = num1 % num2;
10
11// Relational operators
12boolean isGreaterThan = num1 > num2;
13boolean isLessThan = num1 < num2;
14boolean isEqual = num1 == num2;
15boolean isNotEqual = num1 != num2;
16
17// Logical operators
18boolean isTrue = true;
19boolean isFalse = false;
20boolean logicalAnd = isTrue && isFalse;
21boolean logicalOr = isTrue || isFalse;
22boolean logicalNot = !isTrue;
23
Control Structures in Java
Control structures allow developers to control the flow of program execution based on conditions. Main control structures include if-else, switch, while, do-while, and for loops.
1// If-else statement
2int age = 18;
3if (age >= 18) {
4 System.out.println("You are an adult.");
5} else {
6 System.out.println("You are a minor.");
7}
8
9// Switch statement
10char grade = 'A';
11switch (grade) {
12 case 'A':
13 System.out.println("Excellent!");
14 break;
15 case 'B':
16 System.out.println("Good!");
17 break;
18 case 'C':
19 System.out.println("Average!");
20 break;
21 default:
22 System.out.println("Need Improvement!");
23 break;
24}
25
26// While loop
27int count = 1;
28while (count <= 5) {
29 System.out.println("Count: " + count);
30 count++;
31}
32
33// Do-while loop
34int i = 1;
35do {
36 System.out.println("i: " + i);
37 i++;
38} while (i <= 5);
39
40// For loop
41for (int j = 1; j <= 5; j++) {
42 System.out.println("j: " + j);
43}
Arrays in Java
Arrays are essential data structures used to store multiple elements of the same data type in contiguous memory locations.
1// Declaration and initialization of an array
2int[] numbers = {1, 2, 3, 4, 5};
3
4// Accessing elements of the array
5System.out.println("Element at index 0: " + numbers[0]);
6System.out.println("Element at index 2: " + numbers[2]);
7
8// Modifying elements of the array
9numbers[3] = 10;
10
11// Array length
12int length = numbers.length;
13System.out.println("Length of the array: " + length);
Classes in Java
Classes are fundamental to object-oriented programming in Java. They provide a blueprint for creating objects, encapsulating data, and defining behavior.
1// Define a class named "Person"
2public class Person {
3 // Properties
4 private String name;
5 private int age;
6
7 // Constructor
8 public Person(String name, int age) {
9 this.name = name;
10 this.age = age;
11 }
12
13 // Method to display information
14 public void displayInfo() {
15 System.out.println("Name: " + name);
16 System.out.println("Age: " + age);
17 }
18}
19
20// Create objects of the class
21Person person1 = new Person("John", 30);
22Person person2 = new Person("Jane", 25);
23
24// Display information of the objects
25person1.displayInfo();
26person2.displayInfo();
27
Access Modifiers in Java
Access modifiers determine the accessibility of classes, methods, and variables. The main access modifiers in Java are public, private, protected, and default (package-private).
Method Overloading in Java
Method overloading enables creating several methods with the same name but different sets of inputs.
1public class MathOperations {
2 // Method to add two integers
3 public int add(int num1, int num2) {
4 return num1 + num2;
5 }
6
7 // Method to add three integers
8 public int add(int num1, int num2, int num3) {
9 return num1 + num2 + num3;
10 }
11
12 // Method to add two double values
13 public double add(double num1, double num2) {
14 return num1 + num2;
15 }
16}
Garbage Collection in Java
Java's garbage collector automatically reclaims memory occupied by objects no longer in use, preventing memory leaks and simplifying memory management.
"This" Keyword in Java
In Java, "this" keyword refers to the current instance of a class. It distinguishes between different instances and resolves ambiguity when variables have the same name.
1public class Person {
2 private String name;
3
4 public Person(String name) {
5 this.name = name;
6 }
7
8 public void displayName() {
9 System.out.println("Name: " + this.name);
10 }
11}
Static (Variable, Method, Block) in Java
The "static" keyword indicates that a variable, method, or block belongs to a class rather than an instance of a class. You can use static members without making any objects.
1public class MathUtility {
2 public static final double PI = 3.14159;
3 public static int counter = 0;
4
5 public static int add(int num1, int num2) {
6 return num1 + num2;
7 }
8
9 static {
10 // This is a static block that runs when the class is loaded
11 System.out.println("Static block executed.");
12 }
13}
final Keyword in Java
The "final" keyword indicates that a variable, method, or class cannot be modified or extended. It ensures stability and prevents method overriding.
1public class Circle {
2 public static final double PI = 3.14159;
3 private final double radius;
4
5 public Circle(double radius) {
6 this.radius = radius;
7 }
8
9 public double calculateArea() {
10 return PI * radius * radius;
11 }
12}
Wrapper Classes in Java
Wrapper classes offer a method to deal with basic data types like objects. They encapsulate primitive values and offer useful methods for manipulation.
String Class in Java
The String class is extensively used in Java to represent sequences of characters. It offers a variety of methods for string manipulation, concatenation, and comparison.
1public class StringExamples {
2 public static void main(String[] args) {
3 String str1 = "Hello";
4 String str2 = "World";
5
6 // Concatenation
7 String result = str1 + " " + str2;
8 System.out.println(result);
9
10 // Length of the string
11 int length = str1.length();
12 System.out.println("Length of str1: " + length);
13
14 // Substring
15 String subStr = str2.substring(0, 3);
16 System.out.println("Substring of str2: " + subStr);
17
18 // IndexOf
19 int index = str1.indexOf('e');
20 System.out.println("Index of 'e' in str1: " + index);
21
22 // UpperCase and LowerCase
23 String upperCaseStr = str1.toUpperCase();
24 String lowerCaseStr = str2.toLowerCase();
25 System.out.println("Upper case: " + upperCaseStr);
26 System.out.println("Lower case: " + lowerCaseStr);
27 }
28}
Each of these topics is covered comprehensively in this composition, complete with numerical examples to enhance your understanding.