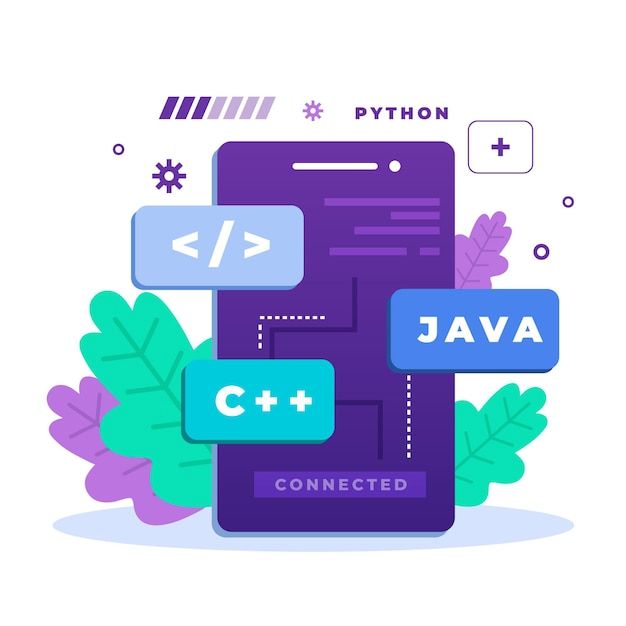
OOPS Concepts in Java with Examples
What is OOPs in Java?
- Object-Oriented Programming (OOP) in Java is a programming paradigm that organizes code around objects, classes, and their interactions.
- OOP comprises several principles such as abstraction, encapsulation, inheritance, and polymorphism.
OOPs Concepts in Java
- Objects
- Classes
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
Objects
- In Java, everything is an object. An object represents a real-world entity and can have attributes (characteristics) and methods (actions).
- Objects are instances of classes, which serve as blueprints for creating objects.
Classes
- To work with objects, you need to create a class that defines the blueprint for those objects.
- Let's create a simple class called Car to represent a car:
1public class Car {
2 // Attributes (Characteristics)
3 String color;
4 String model;
5
6 // Methods (Actions)
7 public void start() {
8 System.out.println("The car is starting.");
9 }
10
11 public void stop() {
12 System.out.println("The car is stopping.");
13 }
14}
In this class, we have attributes (color and model) and methods (start() and stop()) that a car can have.
Creating an Object
Now that we have our class, we can create an object based on it. In Java, we use the new keyword to create an instance (object) of a class. For example:
1Car myCar = new Car();
Here, myCar is an object of the Car class. It's created based on the Car blueprint.
Setting Object Attributes
You can set the attributes of an object using dot notation. For instance, to set the color and model of myCar:
1myCar.color = "black";
2myCar.model = "Toyota";
3
Using Object Methods
You can call the methods of an object using dot notation as well. For example, to start and stop the car:
1myCar.start();
2myCar.stop();
3
Here's the complete Java code combining all the steps:
1public class Car {
2 // Attributes (Characteristics)
3 String color;
4 String model;
5
6 // Methods (Actions)
7 public void start() {
8 System.out.println("The car is starting.");
9 }
10
11 public void stop() {
12 System.out.println("The car is stopping.");
13 }
14
15 public static void main(String[] args) {
16 // Creating an instance (object) of the Car class
17 Car myCar = new Car();
18
19 // Setting attributes for the car
20 myCar.color = "blue";
21 myCar.model = "Toyota";
22
23 // Using methods to perform actions
24 myCar.start();
25 myCar.stop();
26 }
27}
Abstraction in Java
- Abstraction involves showing only the necessary features of an object while hiding the unnecessary details.
- Think of a TV remote control - you don't need to know how it works internally. You just need to know the buttons to operate it.
- In Java, you achieve abstraction using abstract classes and interfaces.
Encapsulation in Java
- Encapsulation is the practice of hiding the internal state of an object and providing controlled access to it.
- It's like putting your data in a protective capsule.
- You use private variables and public methods to achieve encapsulation.
Inheritance in Java
- Inheritance is a concept that allows you to create new classes based on existing classes.
- It allows one class to inherit properties and behaviors from another class.
- It facilitates code reusability.
Polymorphism in Java
- Polymorphism in Java means one name, many forms.
- For example, both a "Car" and an "SUV" can be referred to as a "Vehicle" if they are inherited from a common class.
- Polymorphism in Java can be classified into two primary types compile-time (or static) polymorphism and runtime (or dynamic) polymorphism. Let's explore these two types
Compile-time Polymorphism in Java
- static-time polymorphism is also known as method overloading.
- It occurs when there are multiple methods in the same class with the same name but different parameters (method signature).
- The correct method to be executed is determined at compile time-based on the method's parameters.
Runtime Polymorphism in Java
- Dynamic time polymorphism is also known as method overriding.
- It occurs when a subclass offers a unique implementation of a method that already exists in its superclass.
Advantages of OOPS
Reusability
- OOP encourages code reusability through inheritance and composition.
- You can create new classes by extending or combining existing ones, reducing redundant code and development time.
Maintenance and Debugging
- OOP makes code maintenance and debugging more straightforward.
- Classes and objects encapsulate functionality, making it easier to locate and fix issues within specific components without affecting the entire application.
Scalability
- OOP supports the development of scalable systems.
- As the complexity of a project grows, OOP principles help manage that complexity by breaking it down into manageable, interconnected components.
Conclusion
We simplified Java Oops concepts: objects, classes, inheritance, polymorphism, abstraction, and encapsulation, with real-world examples.