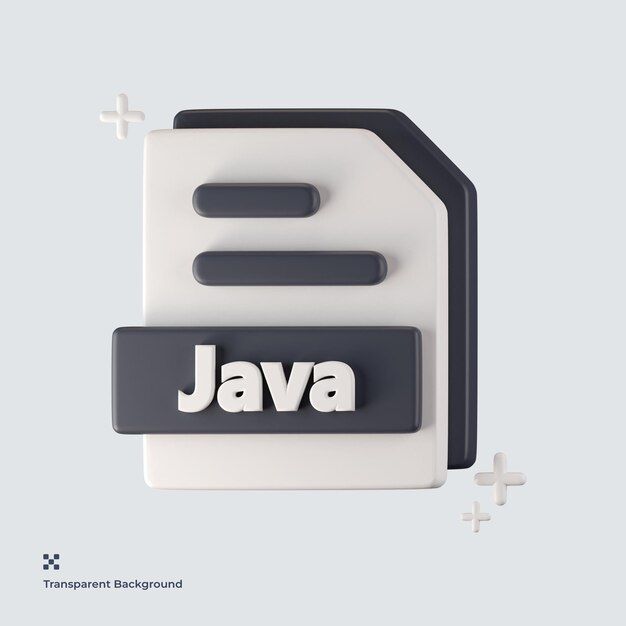
Constructors in Java
What is Constructor in Java?
Constructors are special methods within a class that are responsible for initializing the instance variables of an object when it is created. They have the same name as the class and do not have a return type, not even 'void'.
Types of Constructors in Java
Java Default Constructor
The default constructor is automatically generated by Java if no constructor is defined explicitly in the class. It initializes instance variables to their default values. For example, in a class named Person, the default constructor would be:
1public Person() {
2 // Default constructor code
3}
Java Parameterized Constructor
Parameterized constructors accept parameters to initialize instance variables during object creation. They provide a way to customize object initialization. Consider the following example for a Book class:
1public Book(String title, String author) {
2 this.title = title;
3 this.author = author;
4}
Constructor Overloading in Java
Constructor overloading involves having multiple constructors within a class, each with a different number or type of parameters. This allows for flexibility in object creation. Here's an example using a Rectangle class:
1public Rectangle() {
2 // Default constructor
3}
4
5public Rectangle(int width, int height) {
6 this.width = width;
7 this.height = height;
8}
Java Copy Constructor
A copy constructor creates a new object by copying the values of another object. It's useful for creating duplicates. Consider a Student class example:
1public Student(Student original) {
2 this.name = original.name;
3 this.age = original.age;
4}
Initializing Instance Variables
Constructors initialize instance variables and can perform additional setup tasks. For example, initializing collections or connecting to databases.
Chaining Constructors in Java
Chaining constructors allows one constructor to call another within the same class using this(). This reduces redundancy and ensures consistent initialization.
1public Car(String model) {
2 this(model, "Unknown");
3}
4
5public Car(String model, String make) {
6 this.model = model;
7 this.make = make;
8}
Calling Constructors from Constructors in Java
Constructors can also call constructors from their parent class using super(). This ensures that the parent class's initialization is taken care of.
Constructor Best Practices in Java
- Keep constructors concise.
- Avoid unnecessary logic.
- Favor method calls over logic duplication.
- Follow naming conventions.
Summary of Constructor Types
- Default Constructor
- Parameterized Constructor
- Copy Constructor
- Constructor Overloading
Step-by-Step Guide
Now, let's walk through a step-by-step guide with code examples showcasing different types of constructors. We'll use practical scenarios to illustrate their usage.
Step 1: Create a Java Class
Create a Java class with a meaningful name, for example, Employee.
1public class Employee {
2 // Class members will go here
3}
4
Step 2: Add a Default Constructor
Add a default constructor to the class.
1 public Employee() {
2 // Default constructor code
3}
Step 3: Implement Parameterized Constructor
1public Employee(String name, int age) {
2 this.name = name;
3 this.age = age;
4}
Continue this step-by-step guide with more examples and code explanations to cover various constructor scenarios.
Conclusion
Constructors are a cornerstone of Java programming, providing a means to set up objects and prepare them for use. They come in different types, each serving specific purposes. By following best practices and understanding when and how to use different types of constructors, you can write efficient and well-organized Java code.