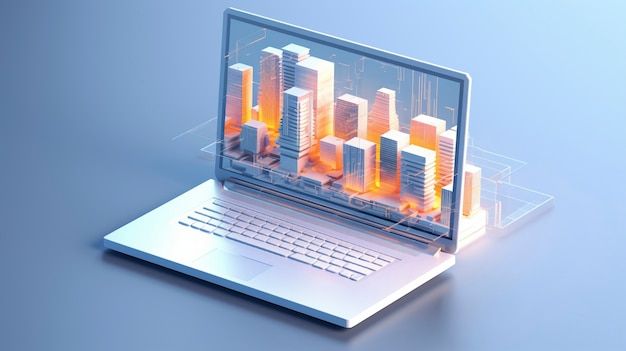
Line Graph, Bar charts, Pie-charts, Scatter plots, multiple plots
Line Graph
- Line graphs are used to visualize trends or changes over time.
- They are created using the plt.plot() function in Matplotlib.
- Labels and titles can be added to provide context and clarity.
1import matplotlib.pyplot as plt
2
3# Data
4x = [1, 2, 3, 4, 5]
5y = [2, 4, 6, 8, 10]
6
7# Create the line graph
8plt.plot(x, y)
9
10# Add labels and title
11plt.xlabel('X-axis')
12plt.ylabel('Y-axis')
13plt.title('Line Graph')
14
15# Display the graph
16plt.show()
- We define the data points for the x-axis and y-axis.Using plt.plot(), we plot the data points on the graph.
- We add labels for the x-axis and y-axis using plt.xlabel() and plt.ylabel(), respectively.
- We set a title for the graph using plt.title().Finally, we display the graph using plt.show().
Bar Chart
- Bar charts are used to visually compare categorical data by displaying the magnitude of different categories.
- In Matplotlib, bar charts are created using the plt.bar() function, which
- allows for customization of the bar width and color to enhance the visualization.
1import matplotlib.pyplot as plt
2
3# Data
4categories = ['A', 'B', 'C', 'D']
5values = [10, 20, 15, 25]
6
7# Create the bar chart
8plt.bar(categories, values)
9
10# Add labels and title
11plt.xlabel('Categories')
12plt.ylabel('Values')
13plt.title('Bar Chart')
14
15# Display the chart
16plt.show()
Pie Chart
- Pie charts are used to visually display the proportion or percentage of each part
- within a whole, providing a clear representation of the relative sizes of different components.
- They are created using the plt.pie() function in Matplotlib.
1import matplotlib.pyplot as plt
2
3# Data
4labels = ['Category 1', 'Category 2', 'Category 3']
5sizes = [30, 50, 20]
6
7# Create the pie chart
8plt.pie(sizes, labels=labels, autopct='%1.1f%%')
9
10# Add a title
11plt.title('Pie Chart')
12
13# Display the chart
14plt.show()
Scatter Plot
- Scatter plots are used to graphically illustrate the correlation or relationship between two variables,
- allowing for the visualization of patterns, trends, and associations between the variables.
- They are created using the plt.scatter() function in Matplotlib.
1import matplotlib.pyplot as plt
2
3# Data
4x = [1, 2, 3, 4, 5]
5y = [2, 4, 6, 8, 10]
6
7# Create the scatter plot
8plt.scatter(x, y)
9
10# Add labels and title
11plt.xlabel('X-axis')
12plt.ylabel('Y-axis')
13plt.title('Scatter Plot')
14
15# Display the plot
16plt.show()
Multiple Plots
- Multiple plots can be created in a single figure using the subplots() function.
- Each subplot can have its own plot type and customization.
- Spacing between subplots can be adjusted using plt.tight_layout().
1import matplotlib.pyplot as plt
2
3# Create a figure and subplots
4fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
5
6# Plot 1
7ax1.plot([1, 2, 3], [4, 5, 6])
8ax1.set_title('Plot 1')
9
10# Plot 2
11ax2.bar(['A', 'B', 'C'], [10, 20, 15])
12ax2.set_title('Plot 2')
13
14# Adjust spacing between subplots
15plt.tight_layout()
16
17# Display the plots
18plt.show()
- We create a figure and subplots using plt.subplots().
- In this example, we create a figure with two subplots arranged horizontally.
- We access each subplot using ax1 and ax2 and create different plots on each subplot.
- We set titles for each subplot using ax1.set_title() and ax2.set_title().We adjust the spacing between subplots using plt.tight_layout().
Subplots
- Subplots allow the creation of multiple plots within a single figure.
- They are created using the plt.subplots() function in Matplotlib.
- Subplots can be arranged in a grid-like structure.
1import matplotlib.pyplot as plt
2
3# Create a figure and subplots
4fig, axs = plt.subplots(2, 2, figsize=(8, 8))
5
6# Plot 1
7axs[0, 0].plot([1, 2, 3], [4, 5, 6])
8axs[0, 0].set_title('Plot 1')
9
10# Plot 2
11axs[0, 1].scatter([1, 2, 3], [4, 5, 6])
12axs[0, 1].set_title('Plot 2')
13
14# Plot 3
15axs[1, 0].bar(['A', 'B', 'C'], [10, 20, 15])
16axs[1, 0].set_title('Plot 3')
17
18# Plot 4
19sizes = [30, 50, 20]
20axs[1, 1].pie(sizes)
21axs[1, 1].set_title('Plot 4')
22
23# Adjust spacing between subplots
24plt.tight_layout()
25
26# Display the plots
27plt.show()
- We define the data points for the x-axis and two different y-axis values (y1 and y2).
- We create two lines on the same plot using plt.plot() and provide labels for each line using the label parameter.
- We add labels and a title similar to the previous examples.
- We add a legend to the plot using plt.legend(), which automatically uses the labels provided in the plot() function.
Legends
- Legends provide a key to understand the different elements in a plot.
- They are added to a plot using the plt.legend() function.
- Legends automatically use the labels provided in the plotting functions.
1import matplotlib.pyplot as plt
2
3# Data
4x = [1, 2, 3, 4, 5]
5y1 = [2, 4, 6, 8, 10]
6y2 = [1, 3, 5, 7, 9]
7
8# Create the plot
9plt.plot(x, y1, label='Line 1')
10plt.plot(x, y2, label='Line 2')
11
12# Add labels and title
13plt.xlabel('X-axis')
14plt.ylabel('Y-axis')
15plt.title('Plot with Legend')
16
17# Add a legend
18plt.legend()
19
20# Display the plot
21plt.show()
- We define the data points for the x-axis and two different y-axis values (y1 and y2).
- We create two lines on the same plot using plt.plot() and provide labels for each line using the label parameter.
- We add labels and a title similar to the previous examples.
- We add a legend to the plot using plt.legend(), which automatically uses the labels provided in the plot() function.
Changing Figure Size
- The figure size can be adjusted using the figsize parameter in the plt.figure() function.
- The figsize parameter in Matplotlib accepts a tuple of two values, which specify the width and height of the figure in inches.
- Changing the figure size allows you to control the overall dimensions of the plot.
Example code:
1import matplotlib.pyplot as plt
2
3# Create a figure with a specific size
4plt.figure(figsize=(8, 6))
5
6# Create your plot here
7plt.plot([1, 2, 3], [4, 5, 6])
8
9plt.show()
Styling Plots using Matplotlib Library
- Matplotlib provides various built-in styles that can be applied to plots.
- Styles can be set using the plt.style.use() function.
- Some popular styles include 'default', 'ggplot', 'seaborn', and 'fivethirtyeight'.
- Example code:
1import matplotlib.pyplot as plt
2
3# Set the style
4plt.style.use('ggplot')
5
6# Create your plot here
7plt.plot([1, 2, 3], [4, 5, 6])
8
9plt.title('Plot with ggplot Style')
10plt.xlabel('X-axis')
11plt.ylabel('Y-axis')
12
13plt.show()
- In the first example, we use plt.figure(figsize=(8, 6)) to create a figure with a width of 8 inches and a height of 6 inches.
- This allows us to control the size of the plot based on our requirements.
- In the second example, we use plt.style.use('ggplot') to apply the 'ggplot' style to the plot.
- The 'ggplot' style mimics the aesthetics of the ggplot2 library in R, providing a visually appealing and professional look to the plot.
Seaborn Library
- Seaborn is a statistical visualization library that leverages Matplotlib to create informative and visually
- appealing statistical graphics, offering a high-level interface for users to easily generate a wide range of statistical plots.
- Seaborn integrates well with Pandas data structures and supports numpy and scipy.
Line Plot
- Line plots in Seaborn are used to visualize trends and patterns in data over a continuous variable.
- They are created using the sns.lineplot() function.
- Example code:
1import seaborn as sns
2import matplotlib.pyplot as plt
3
4# Load the example dataset
5tips = sns.load_dataset("tips")
6
7# Create a line plot
8sns.lineplot(x="total_bill", y="tip", data=tips)
9
10plt.show()
Dist Plot
- Dist plots (distribution plots) in Seaborn are used to visualize the distribution of a univariate set of observations.
- They are created using the sns.distplot() function.
- Example code:
1import seaborn as sns
2import matplotlib.pyplot as plt
3
4# Load the example dataset
5tips = sns.load_dataset("tips")
6
7# Create a dist plot
8sns.distplot(tips["total_bill"])
9
10plt.show()
11
Lmplot
- Lmplot (regression plot) in Seaborn is used to plot data and regression model fits across a FacetGrid.
- It is created using the sns.lmplot() function.
- Example code:
1import seaborn as sns
2import matplotlib.pyplot as plt
3
4# Load the example dataset
5tips = sns.load_dataset("tips")
6
7# Create an lmplot
8sns.lmplot(x="total_bill", y="tip", data=tips)
9
10plt.show()
Count Plot
- Count plots in Seaborn are used to show the counts of observations in each categorical bin using bars.
- They are created using the sns.countplot() function.
1import seaborn as sns
2import matplotlib.pyplot as plt
3
4# Load the example dataset
5tips = sns.load_dataset("tips")
6
7# Create a count plot
8sns.countplot(x="day", data=tips)
9
10plt.show()
Color Palettes
- Seaborn provides a variety of color palettes to enhance the aesthetics of your plots.
- Color palettes can be set using the sns.set_palette() function.
1import seaborn as sns
2import matplotlib.pyplot as plt
3
4# Load the example dataset
5tips = sns.load_dataset("tips")
6
7# Set the color palette
8sns.set_palette("husl")
9
10# Create a scatter plot with the color palette applied
11sns.scatterplot(x="total_bill", y="tip", data=tips)
12
13plt.show()
Conclusion
We have covered basics of Line Graph, Bar charts, Pie-charts, Scatter plots, multiple plots, Subplots, Legends, Functions like relplot(), displot() ,catplot ()
and Seaborn Library
and Seaborn Library