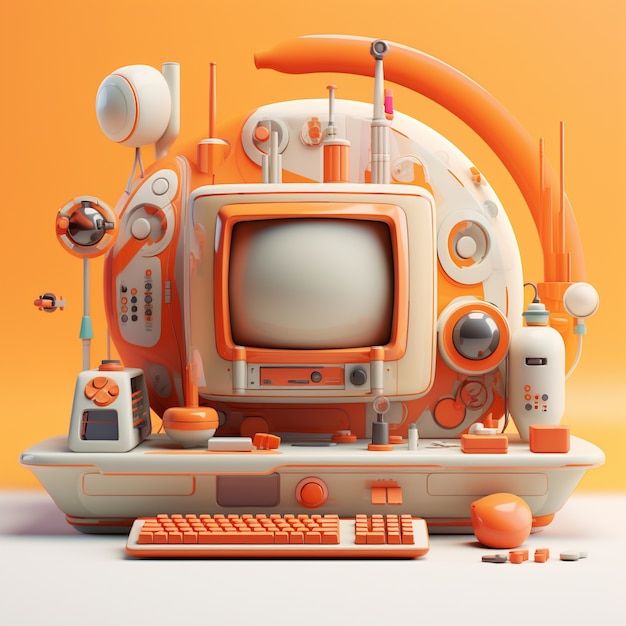
GUI Programming, GUI Widgets and Database Access | DV
GUI Programming with Tkinter
- Tkinter is a built-in Python module that allows you to create graphical user interfaces (GUIs) for your Python applications.
- It provides a wide range of widgets and tools to build interactive and user-friendly interfaces.
Creating a User Interface
To create a user interface using Tkinter, you need to follow these steps:
Import the Tkinter module
1import tkinter as tk
Create the main window
1window = tk.Tk()
2
3window.title("My App")
4
5window.geometry("400x300")
Add widgets to the window
1label = tk.Label(window, text="Hello, Tkinter!")
2
3label.pack()
4
5Start the main event loop:
6
7window.mainloop()
GUI Widgets
Tkinter provides various widgets that you can use to build your user interface. Here are some commonly used widgets:
Label: Displays text or images.
1label = tk.Label(window, text="This is a label")
2
3label.pack()
Button: Creates a clickable button.
1button = tk.Button(window, text="Click me!", command=button_clicked)
2
3button.pack()
Entry: Allows user input in a single-line text field.
1entry = tk.Entry(window)
2
3entry.pack()
Text: Provides a multi-line text area for displaying or editing text.
1text = tk.Text(window, height=5, width=30)
2
3text.pack()
Check Box
- A Check Box enables the user to either select or deselect an option.
- Use the Checkbutton widget to create a check box.
- You can associate a variable with the check box to retrieve its state.
1var = tk.BooleanVar()
2
3checkbutton = tk.Checkbutton(window, text="Option", variable=var)
4
5checkbutton.pack()
Radio Buttons
- Radio Buttons allow the user to select one option from a group of options.
- Use the Radiobutton widget to create radio buttons.
- Radio buttons in the same group should share the same variable to ensure mutual exclusivity.
1var = tk.IntVar()
2
3radio1 = tk.Radiobutton(window, text="Option 1", variable=var, value=1)
4
5radio2 = tk.Radiobutton(window, text="Option 2", variable=var, value=2)
6
7radio1.pack()
8
9radio2.pack()
List Box
- A List Box displays a list of items from which the user can select one or multiple items.
- Use the Listbox widget to create a list box.
- You can add items to the list box using the insert() method and retrieve the selected item(s) using the curselection() method.
1listbox = tk.Listbox(window)
2
3listbox.insert(1, "Item 1")
4
5listbox.insert(2, "Item 2")
6
7listbox.pack()
Creating Layouts
Tkinter provides several layout managers to arrange widgets in a window. The most commonly used layout managers are:
Pack Layout
- The Pack layout arranges widgets in a vertical or horizontal direction.
- Widgets are added to the window or frame in a sequential order.
- Use the pack() method to add widgets to the layout.
1label.pack(side=tk.LEFT, padx=10)
2
3button.pack(side=tk.RIGHT, pady=5)
Grid Layout
- The Grid layout arranges widgets in a table-like structure.
- Widgets are placed in specific rows and columns using the grid() method.
- You can specify the number of rows and columns and adjust the spacing between cells.
1label.grid(row=0, column=0)
2
3entry.grid(row=0, column=1)
4
5button.grid(row=1, columnspan=2)
Place Layout
- The Place layout allows precise positioning of widgets using coordinates.
- Widgets are placed at specific x and y coordinates using the place() method.
- You can specify absolute or relative coordinates for positioning.
1label.place(x=50, y=100)
2
3button.place(relx=0.5, rely=0.5, anchor=tk.CENTER)
Menus
- Menus provide a hierarchical structure for organizing commands and options.
- Use the Menu widget to create menus.
- Menus can be attached to the main window or to other widgets like buttons.
Menu Options
- Menu options are the individual items within a menu.
- Use the add_command() method to add options to a menu.
- You can associate commands or functions with menu options using the command parameter.
1menubar = tk.Menu(window)
2
3filemenu = tk.Menu(menubar, tearoff=0)
4
5filemenu.add_command(label="Open", command=open_file)
6
7filemenu.add_command(label="Save", command=save_file)
8
9filemenu.add_separator()
10
11filemenu.add_command(label="Exit", command=window.quit)
12
13menubar.add_cascade(label="File", menu=filemenu)
14
15window.config(menu=menubar)
Dialog Boxes
- Dialog Boxes are separate windows that display information or prompt the user for input.
- Tkinter provides various built-in dialog boxes, such as messagebox, filedialog, and colorchooser.
- Dialog boxes can be used for displaying messages, selecting files or directories, choosing colors, and more.
1File Dialog:
2
3from tkinter import filedialog
4
5file_path = filedialog.askopenfilename()
6
7Message Box:
8
9from tkinter import messagebox
10
11messagebox.showinfo("Info", "This is an information message")
12
13Input Dialog:
14
15from tkinter import simpledialog
16
17user_input = simpledialog.askstring("Input", "Enter your name:")
Database Access
- Python provides various modules for connecting to and interacting with databases.
- One commonly used module is sqlite3, which allows you to work with SQLite databases.
Database Connectivity Operations
Here are the basic database connectivity operations using sqlite3:
Connect to the database:
1import sqlite3
2
3conn = sqlite3.connect('example.db')
4
5Create a table:
6
7c = conn.cursor()
8
9c.execute('''CREATE TABLE IF NOT EXISTS users
10
11(id INTEGER PRIMARY KEY,
12
13name TEXT,
14
15age INTEGER)''')
16
17conn.commit()
18
19Insert data into the table:
20
21c.execute("INSERT INTO users (name, age) VALUES (?, ?)", ('John', 25))
22
23conn.commit()
24
25Select data from the table:
26
27c.execute("SELECT * FROM users")
28
29rows = c.fetchall()
30
31for row in rows:
32
33print(row)
34
35Update data in the table:
36
37c.execute("UPDATE users SET age = ? WHERE name = ?", (30, 'John'))
38
39conn.commit()
40
41Delete data from the table:
42
43c.execute("DELETE FROM users WHERE name = ?", ('John',))
44
45conn.commit()
46
47Drop the table:
48
49c.execute("DROP TABLE IF EXISTS users")
50
51conn.commit()
52
53Close the database connection:
54
55conn.close()
- These are just a few examples of the database operations you can perform using sqlite3.
- You can execute various SQL queries and statements to manipulate and retrieve data from the database.
- Remember to always commit your changes after executing write operations (INSERT, UPDATE, DELETE) to ensure they are saved in the database.
Example
Here's a complete example that demonstrates creating a simple GUI application with a database connection:
1import tkinter as tk
2
3import sqlite3
4
5
6
7def save_data():
8
9name = entry_name.get()
10
11age = entry_age.get()
12
13conn = sqlite3.connect('example.db')
14
15c = conn.cursor()
16
17c.execute("INSERT INTO users (name, age) VALUES (?, ?)", (name, age))
18
19conn.commit()
20
21conn.close()
22
23entry_name.delete(0, tk.END)
24
25entry_age.delete(0, tk.END)
26
27
28
29def display_data():
30
31conn = sqlite3.connect('example.db')
32
33c = conn.cursor()
34
35c.execute("SELECT * FROM users")
36
37rows = c.fetchall()
38
39conn.close()
40
41listbox.delete(0, tk.END)
42
43for row in rows:
44
45listbox.insert(tk.END, row)
46
47
48
49window = tk.Tk()
50
51window.title("User Management")
52
53
54
55label_name = tk.Label(window, text="Name:")
56
57label_name.pack()
58
59entry_name = tk.Entry(window)
60
61entry_name.pack()
62
63
64
65label_age = tk.Label(window, text="Age:")
66
67label_age.pack()
68
69entry_age = tk.Entry(window)
70
71entry_age.pack()
72
73
74
75button_save = tk.Button(window, text="Save", command=save_data)
76
77button_save.pack()
78
79
80
81button_display = tk.Button(window, text="Display", command=display_data)
82
83button_display.pack()
84
85
86
87listbox = tk.Listbox(window)
88
89listbox.pack()
90
91
92
93conn = sqlite3.connect('example.db')
94
95c = conn.cursor()
96
97c.execute('''CREATE TABLE IF NOT EXISTS users
98
99(id INTEGER PRIMARY KEY,
100
101name TEXT,
102
103age INTEGER)''')
104
105conn.commit()
106
107conn.close()
108
109
110
111window.mainloop()
- This example creates a simple user management application with a GUI.
- It allows you to enter a name and age, save the data to a SQLite database, and display the stored data in a listbox widget.
- The save_data() function is called when the "Save" button is clicked.
- It retrieves the entered name and age, connects to the database, inserts the data into the "users" table, and clears the entry fields.
- The display_data() function is called when the "Display" button is clicked.
- It connects to the database, retrieves all the rows from the "users" table, and displays them in the listbox widget.
- The code also creates the necessary table in the database if it doesn't exist when the application starts.
Conclusion
Now we have basic understanding of GUI Programming: Creating User-interface, GUI Widgets with Tkinter, Creating Layouts, Check Box, Radio Buttons, List Box, Menus,Database Access: Database Connectivity Operations: