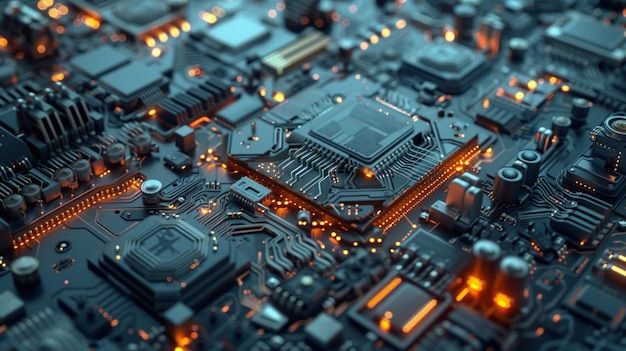
Arduino platform boards & anatomy Arduino IDE
Programming the Arduino for IoT
- Arduino is a popular open-source platform widely used for developing IoT applications.
- It provides a simple and accessible way to program microcontrollers and create interactive projects.
Arduino Platform Boards Anatomy
Arduino boards come in various forms and configurations, but they share some common components:
Microcontroller
- The central component of an Arduino board is its microcontroller, an integrated circuit that can be programmed.
- It executes the code and controls the board's functionality.
- Common microcontrollers used in Arduino boards include ATmega328P, ATmega2560, and ARM Cortex-M.
Digital I/O Pins
- Arduino boards have digital input/output (I/O) pins that can be used to connect sensors, actuators, and other devices.
- These pins can be configured as either inputs or outputs and can read or write digital values (HIGH or LOW).
Analog Input Pins
- Analog input pins allow Arduino boards to read analog voltages from sensors such as temperature sensors, light sensors, or potentiometers.
- These pins have an analog-to-digital converter (ADC) that converts the analog signal into a digital value.
Power Pins
- Arduino boards have power pins that provide regulated voltage to power the microcontroller and connected components.
- The common power pins are VCC (positive voltage) and GND (ground).
Communication Interfaces
- Arduino boards often include communication interfaces such as
- UART (Universal Asynchronous Receiver/Transmitter) and I2C (Inter-Integrated
- Circuit) are all communication protocols commonly used in embedded systems and microcontroller-based devices.
- These interfaces allow the Arduino to communicate with other devices, sensors, or modules.
- Example: The Arduino Uno is a popular board that features an ATmega328P microcontroller, 14 digital I/O pins,
- 6 analog input pins, and a USB connection for programming and power.
Arduino IDE
Cross-platform Compatibility
- Arduino IDE is accessible on various operating systems, such as Windows, macOS, and Linux.
- This cross-platform compatibility allows developers to use the Arduino IDE on their preferred operating system without any constraints.
Integrated Code Editor
- The Arduino IDE includes a built-in code editor that provides features such as: Syntax highlighting , Code auto-completion and Error detection.
- The code editor supports the Arduino programming language, which is based on C/C++,
- making it easier for developers to write and edit code for Arduino boards.
- The code editor highlights different elements of the code, making it easier to read and debug.
- Auto-completion helps in speeding up the coding process by suggesting possible code completions.
- The IDE helps identify and highlight errors in the code, making debugging easier.
Libraries and Examples
- The Arduino IDE comes with a vast collection of pre-written libraries and example sketches.
- Libraries are pre-compiled code modules that provide additional functionality and simplify the programming process.
- The IDE includes a library manager that allows users to easily install, update, and manage libraries.
- Example sketches demonstrate how to use various features and peripherals of Arduino boards, serving as a starting point for beginners.
- The Library Manager allows for easy installation, updates, and management of libraries.
Example Sketches
Pre-written example sketches help users understand how to utilize different features and peripherals of Arduino boards.
Serial Monitor
- The Arduino IDE includes a built-in serial monitor that allows communication between the Arduino board and the computer.
- The serial monitor displays the output sent from the Arduino board and allows sending data from the computer to the board.
- This feature is valuable for debugging, monitoring sensor data, or sending commands to the Arduino board in real-time.
- The serial monitor is an essential tool for debugging and monitoring the performance of the Arduino board.
- It enables real-time communication between the Arduino board and the computer, which is useful for various applications.
Writing Code
- When using an Arduino emulator, you write code using the Arduino programming language, which is based on C/C++.
- The emulator provides a code editor where you can write your sketches (Arduino programs) just like you would in the Arduino IDE.
Simulating Components
- Arduino emulators often provide a virtual breadboard or schematic view
- where you can place and connect various components, such as LEDs, buttons, sensors, and displays.
- This allows you to simulate the behavior of your Arduino project and test how it interacts with different components.
Arduino Simulators
- Arduino simulators are software tools that allow you to write, compile, and run Arduino code without requiring physical hardware.
- They provide a virtual environment that mimics the behavior of an Arduino board,
- enabling developers to test and debug their code before deploying it to actual hardware.
Debugging and Testing
- Emulators offer debugging features that help you identify and fix issues in your code.
- You can set breakpoints, step through your code line by line, and inspect variable values.
- This enables you to test different scenarios, detect bugs, and ensure your code functions as intended before deploying it to physical hardware.
Popular Emulators
- Arduino Simulator: A web-based emulator that allows you to write and run Arduino code directly in your browser.
- Tinkercad: An online platform that provides a virtual Arduino environment for coding and circuit simulation.
- Simulide: A desktop application that offers a complete electronic design automation environment, including Arduino simulation.
Additions in Arduino
Shields
- Arduino shields are modular expansion boards that can be stacked on top of Arduino boards.
- They add specific functionalities such as Wi-Fi connectivity, Ethernet, GPS, motor control, and more.
- Shields provide a convenient way to extend the capabilities of Arduino boards without complex wiring or soldering.
Sensors
- Arduino boards can interface with a wide range of sensors to measure physical quantities.
- These include temperature, humidity, light intensity, pressure, motion, and more.
- Sensors enable Arduino boards to gather data from the environment for interactive and responsive IoT applications.
Actuators
- Actuators convert electrical signals into physical actions.
- Arduino boards can control actuators such as motors, servos, relays, and solenoids.
- This enables the creation of IoT applications involving movement, automation, and external device control.
Wireless Modules
- Arduino boards can be equipped with wireless modules like Wi-Fi, Bluetooth, LoRa, and ZigBee.
- These modules enable wireless communication, networking, and data exchange.
- They facilitate the development of IoT applications that require connectivity and communication with other devices.
Display Modules
- Display modules such as LCD screens and OLED displays can be connected to Arduino boards.
- They provide visual output and user interaction, displaying sensor readings, status information, and messages.
- Display modules enhance the user interface of IoT applications.
Real-Time Clocks
- Real-time clock (RTC) modules keep track of time and date accurately.
- They are useful in IoT applications requiring precise timing, scheduling, and data logging.
- RTCs ensure accurate timekeeping even when the Arduino board is powered off or reset.
Power Management
- Power management modules optimize power consumption and enable battery-powered IoT applications.
- They include battery chargers, voltage regulators, and solar panels.
- Power management modules extend operational time and portability of Arduino-based IoT devices.
Programming the Arduino for IoT
Connectivity
- Arduino boards need to be programmed to connect to the internet or networks using Wi-Fi, Ethernet, or cellular modules.
- Libraries such as WiFi, Ethernet, and GSM manage network connections in Arduino sketches.
Communication Protocols
- Arduino boards can communicate using IoT protocols like MQTT, HTTP, CoAP, and WebSocket.
- Libraries like PubSubClient (MQTT), HTTPClient, and CoAP implement these protocols in Arduino sketches.
Sensor Integration
- Arduino boards read data from sensors and use sensor-specific libraries for integration.
- This involves configuring pins, initializing libraries, and reading sensor values.
Data Processing
- Arduino boards process sensor data locally before sending it to the cloud or other devices.
- This includes filtering, aggregating, and performing calculations based on application requirements.
Cloud Integration
- Arduino boards send data to IoT cloud platforms like Arduino IoT Cloud, AWS IoT, IBM Watson IoT, etc.
- Libraries like ArduinoIo Cloud and PubSubClient facilitate cloud integration in Arduino sketches.
Over-the-Air (OTA) Updates
- Arduino boards support OTA updates for remote firmware updates.
- Libraries like ArduinoOTA and ESP8266HTTPUpdateServer enable OTA updates in Arduino sketches.
Security
- Arduino boards implement secure communication protocols (e.g., HTTPS, SSL/TLS) and encryption.
- Libraries like WiFiClientSecure and Arduino BearSSL provide support for secure communication in Arduino sketches.
Conclusion
We have covered basics of Programming the Arduino: Arduino platform boards anatomy, Arduino IDE, Coding using emulator, Using libraries, Additions in Arduino, Programming the Arduino for IoT.