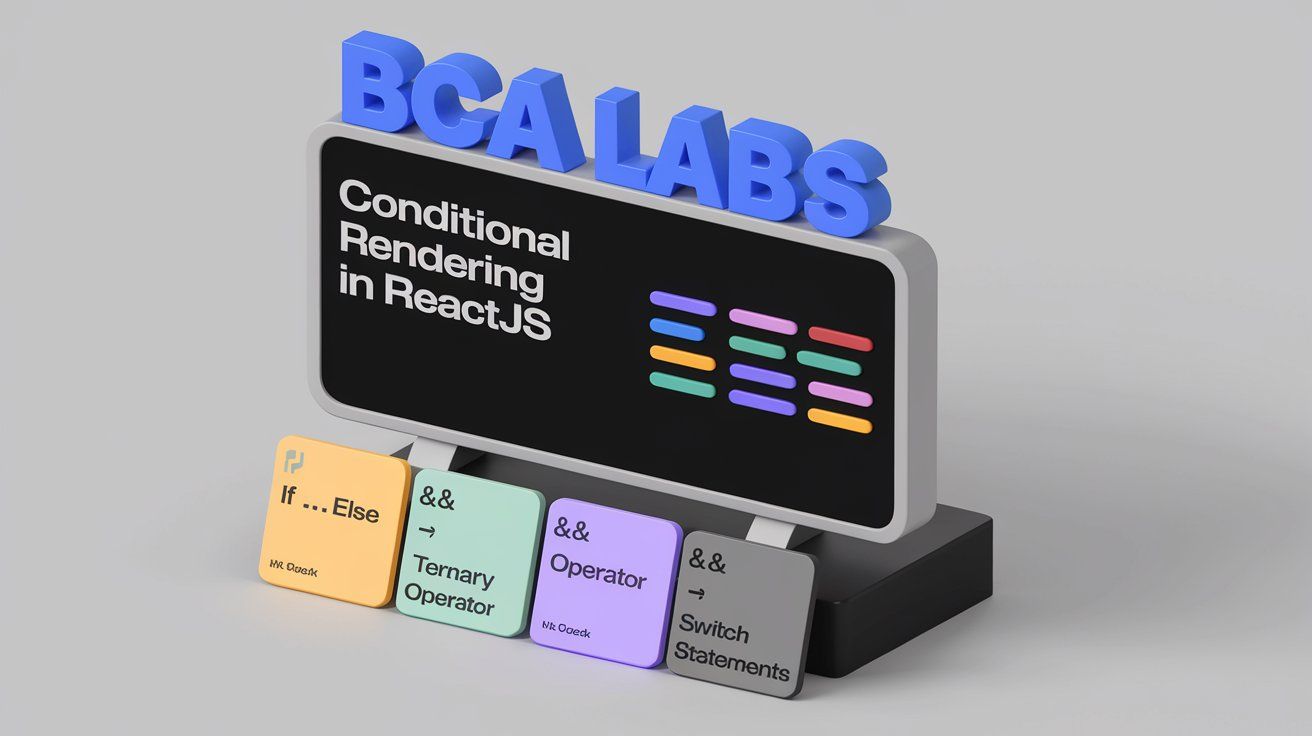
What is Conditional Rendering in ReactJS?
Hello fellow developers! It's time to switch things up a little bit. In this article, we will be discussing about ReactJS things! To start off, we will talk about Conditional Rendering in a react application.
Let's dive in!! 🚀🚀
What is Conditional Rendering?
Conditional Rendering is a concept that allows us to show or hide our components based on some conditions/check. It is a very common pattern when it comes to UI development.
It works the same way we use conditional statements in programming languages, if something is true, show this... else show this.
Why is it Important?
Say you have a full stack application that fetches data from a network location, what happens when a user with slow connection visits the application?
Without Conditional Rendering
- The page will just show blank white space until the data is fetched and then instantly populates the page.
- While we do serve the end purpose of "serving data to the user", we are not following "Good UX principles".
- While the user waits for the data, he has no idea about how long he has to wait, there is no indication whatsoever.
- This causes a bad user experience, and the user might leave before the application loads.
With Conditional Rendering
- We can add checks to see if the data is loaded or not and then according to the result of that check, we could display a loading animation or the data itself.
- This way, we let the user know that the data is being fetched, and you just need to wait for a few more seconds.
- With indications related to the progress, we adhere to the "Good UX principles".
So, Conditional Rendering not only helps with a better user experience, but also helps with:
Performance Optimization
- By conditionally rendering components, we can stop unnecessary renders of the components, and render only what's required.
- This procedure helps in reducing load time, specially in case of larger applications with a lot of components.
Flexible Layout
- Conditional rendering allows you to create more flexible and customizable components.
- By rendering different content, based on the application state, we can create components that can be used in different contexts and adapt to different user interactions.
How to Implement It?
To illustrate the concept of Conditional Rendering, we will create a very basic example of a login state.
- By default, it will show the text "Please Login" and a button to login. Not a fully functional authentication flow, but only fiddling with the state.
- After logging in, we will show our name, which will be rendered conditionally based on out state.
Setup a React Application
First things first, let's create a react application using vite:
- First off run "npx create-vite@latest <project_name>". It will then ask you which framework to use, select React.
- In the next prompt, it will ask you for the language, JS/TS, for this example, we will stick with JS.
- Next up, head into the project directory and install the required dependencies using "npm install".
- Then start the development server using "npm run dev"
All commands in order of execution:
1npx create-vite@latest cr-tut
2cd cr-tut
3npm install
4npm run dev
2 Ways to Implement
Using if... else Statements
1// App.jsx
2
3import { useState } from "react";
4import "./App.css";
5
6const LoggedInContent = ({ state, name }) => (
7 <>
8 <h2>Hello {name}</h2>
9 // Toggle the state on button click
10 <button onClick={() => state((prev) => !prev)}>Log Out</button>
11 </>
12);
13
14const LoggedOutContent = ({ state }) => (
15 <>
16 <h2>Please Log In</h2>
17 // Toggle the state on button click
18 <button onClick={() => state((prev) => !prev)}>Log In</button>
19 </>
20);
21
22function App() {
23 const [loggedIn, setLoggedIn] = useState(false);
24 const name = "Krishang Sharma";
25 let body; // actual content to be shown
26
27 // Check for login status
28 if (loggedIn) {
29 body = <LoggedInContent name={name} state={setLoggedIn} />;
30 } else {
31 body = <LoggedOutContent state={setLoggedIn} />;
32 }
33
34 return <>{body}</>;
35}
36
37export default App;
38
What's in the file?
First, we have two components: <LoggedInContent/> and <LoggedOutContent/>, which are used for the respective logged-in and logged-out states.
Then, in our App component:
- We define state and props: name and state to manage the login status and display user information.
- The state tracks whether the user is logged in or not, and name holds the username.
- We assign the body variable to either LoggedInContent or LoggedOutContent based on the login state, which is then rendered in the DOM.
If you’re unfamiliar with state and props, check out the Props and State Blog.
To learn more about how React interacts with the DOM and JSX, refer to Introduction to React Blog.
Using the Ternary Operator
1import { useState } from "react";
2import "./App.css";
3
4const LoggedInContent = ({ state, name }) => (
5 <>
6 <h2>Hello {name}</h2>
7 <button onClick={() => state((prev) => !prev)}>Log Out</button>
8 </>
9);
10
11const LoggedOutContent = ({ state }) => (
12 <>
13 <h2>Please Log In</h2>
14 <button onClick={() => state((prev) => !prev)}>Log In</button>
15 </>
16);
17
18function App() {
19 const [loggedIn, setLoggedIn] = useState(false);
20 const name = "Krishang Sharma";
21
22 return (
23 <>
24 {loggedIn ? (
25 <LoggedInContent name={name} state={setLoggedIn} />
26 ) : (
27 <LoggedOutContent state={setLoggedIn} />
28 )}
29 </>
30 );
31}
32
33export default App;
34
What's in the file?
You'll notice that we’ve replaced the if... else logic and removed the extra body variable.
With the ternary operator:
- We evaluate the loggedIn state directly inside the JSX.
- It’s more compact, performing the same task as the if... else statement in fewer lines.
How it works:
- Condition: The state variable loggedIn is evaluated.
- True Case: If loggedIn is true, it renders <LoggedInContent>.
- False Case: If loggedIn is false, it renders <LoggedOutContent>.
The ternary operator makes the code easier to read. The ? translates to "if true," while the : acts as an "else."
Using the Logical && Operator
1import { useState } from "react";
2import "./App.css";
3
4const LoggedInContent = ({ state, name }) => (
5 <>
6 <h2>Hello {name}</h2>
7 <button onClick={() => state((prev) => !prev)}>Log Out</button>
8 </>
9);
10
11const LoggedOutContent = ({ state }) => (
12 <>
13 <h2>Please Log In</h2>
14 <button onClick={() => state((prev) => !prev)}>Log In</button>
15 </>
16);
17
18function App() {
19 const [loggedIn, setLoggedIn] = useState(false);
20 const name = "Krishang Sharma";
21
22 return (
23 <>
24 {loggedIn && <LoggedInContent name={name} state={setLoggedIn} />}
25 {!loggedIn && <LoggedOutContent state={setLoggedIn} />}
26 </>
27 );
28}
29
30export default App;
31
What's in the file?
- We used the logical && operator to conditionally render LoggedInContent when loggedIn state is true.
- Similarly, LoggedOutContent is rendered when loggedIn is false by using !loggedIn which translates to not-loggedIn.
This code will work the same way, but with a simpler and more readable structure by leveraging the && operator.
Using the Switch Statement
While it's not used very commonly, we can also use the switch statement to conditionally render components.
- We can add different cases(check) for our state value(loggedIn, loggedOut)
- And for each state value, we can render a component of choice.
Conclusion
In this article we talked about:
- What is Conditional Rendering?
- Why it is important in an application?
- Different ways to implement it:
- if... else statements
- Ternary Operator
- Logical && Operator
- And Switch Statement
I hope the blog was informative and you learned something new! In our next React Blog, we will talk about the Lifecycle Methods of Components in a React application!
That is it for today! And I'll see you you in the next one!! 👋